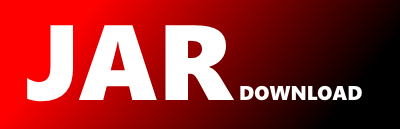
com.pulumi.azurenative.compute.inputs.ImageOSDiskArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute.inputs;
import com.pulumi.azurenative.compute.enums.CachingTypes;
import com.pulumi.azurenative.compute.enums.OperatingSystemStateTypes;
import com.pulumi.azurenative.compute.enums.OperatingSystemTypes;
import com.pulumi.azurenative.compute.enums.StorageAccountTypes;
import com.pulumi.azurenative.compute.inputs.DiskEncryptionSetParametersArgs;
import com.pulumi.azurenative.compute.inputs.SubResourceArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Describes an Operating System disk.
*
*/
public final class ImageOSDiskArgs extends com.pulumi.resources.ResourceArgs {
public static final ImageOSDiskArgs Empty = new ImageOSDiskArgs();
/**
* The Virtual Hard Disk.
*
*/
@Import(name="blobUri")
private @Nullable Output blobUri;
/**
* @return The Virtual Hard Disk.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy