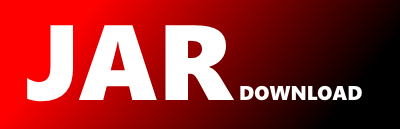
com.pulumi.azurenative.compute.outputs.CloudServicePropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute.outputs;
import com.pulumi.azurenative.compute.outputs.CloudServiceExtensionProfileResponse;
import com.pulumi.azurenative.compute.outputs.CloudServiceNetworkProfileResponse;
import com.pulumi.azurenative.compute.outputs.CloudServiceOsProfileResponse;
import com.pulumi.azurenative.compute.outputs.CloudServiceRoleProfileResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class CloudServicePropertiesResponse {
/**
* @return (Optional) Indicates whether the role sku properties (roleProfile.roles.sku) specified in the model/template should override the role instance count and vm size specified in the .cscfg and .csdef respectively.
* The default value is `false`.
*
*/
private @Nullable Boolean allowModelOverride;
/**
* @return Specifies the XML service configuration (.cscfg) for the cloud service.
*
*/
private @Nullable String configuration;
/**
* @return Specifies a URL that refers to the location of the service configuration in the Blob service. The service package URL can be Shared Access Signature (SAS) URI from any storage account.
* This is a write-only property and is not returned in GET calls.
*
*/
private @Nullable String configurationUrl;
/**
* @return Describes a cloud service extension profile.
*
*/
private @Nullable CloudServiceExtensionProfileResponse extensionProfile;
/**
* @return Network Profile for the cloud service.
*
*/
private @Nullable CloudServiceNetworkProfileResponse networkProfile;
/**
* @return Describes the OS profile for the cloud service.
*
*/
private @Nullable CloudServiceOsProfileResponse osProfile;
/**
* @return Specifies a URL that refers to the location of the service package in the Blob service. The service package URL can be Shared Access Signature (SAS) URI from any storage account.
* This is a write-only property and is not returned in GET calls.
*
*/
private @Nullable String packageUrl;
/**
* @return The provisioning state, which only appears in the response.
*
*/
private String provisioningState;
/**
* @return Describes the role profile for the cloud service.
*
*/
private @Nullable CloudServiceRoleProfileResponse roleProfile;
/**
* @return (Optional) Indicates whether to start the cloud service immediately after it is created. The default value is `true`.
* If false, the service model is still deployed, but the code is not run immediately. Instead, the service is PoweredOff until you call Start, at which time the service will be started. A deployed service still incurs charges, even if it is poweredoff.
*
*/
private @Nullable Boolean startCloudService;
/**
* @return The unique identifier for the cloud service.
*
*/
private String uniqueId;
/**
* @return Update mode for the cloud service. Role instances are allocated to update domains when the service is deployed. Updates can be initiated manually in each update domain or initiated automatically in all update domains.
* Possible Values are <br /><br />**Auto**<br /><br />**Manual** <br /><br />**Simultaneous**<br /><br />
* If not specified, the default value is Auto. If set to Manual, PUT UpdateDomain must be called to apply the update. If set to Auto, the update is automatically applied to each update domain in sequence.
*
*/
private @Nullable String upgradeMode;
private CloudServicePropertiesResponse() {}
/**
* @return (Optional) Indicates whether the role sku properties (roleProfile.roles.sku) specified in the model/template should override the role instance count and vm size specified in the .cscfg and .csdef respectively.
* The default value is `false`.
*
*/
public Optional allowModelOverride() {
return Optional.ofNullable(this.allowModelOverride);
}
/**
* @return Specifies the XML service configuration (.cscfg) for the cloud service.
*
*/
public Optional configuration() {
return Optional.ofNullable(this.configuration);
}
/**
* @return Specifies a URL that refers to the location of the service configuration in the Blob service. The service package URL can be Shared Access Signature (SAS) URI from any storage account.
* This is a write-only property and is not returned in GET calls.
*
*/
public Optional configurationUrl() {
return Optional.ofNullable(this.configurationUrl);
}
/**
* @return Describes a cloud service extension profile.
*
*/
public Optional extensionProfile() {
return Optional.ofNullable(this.extensionProfile);
}
/**
* @return Network Profile for the cloud service.
*
*/
public Optional networkProfile() {
return Optional.ofNullable(this.networkProfile);
}
/**
* @return Describes the OS profile for the cloud service.
*
*/
public Optional osProfile() {
return Optional.ofNullable(this.osProfile);
}
/**
* @return Specifies a URL that refers to the location of the service package in the Blob service. The service package URL can be Shared Access Signature (SAS) URI from any storage account.
* This is a write-only property and is not returned in GET calls.
*
*/
public Optional packageUrl() {
return Optional.ofNullable(this.packageUrl);
}
/**
* @return The provisioning state, which only appears in the response.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Describes the role profile for the cloud service.
*
*/
public Optional roleProfile() {
return Optional.ofNullable(this.roleProfile);
}
/**
* @return (Optional) Indicates whether to start the cloud service immediately after it is created. The default value is `true`.
* If false, the service model is still deployed, but the code is not run immediately. Instead, the service is PoweredOff until you call Start, at which time the service will be started. A deployed service still incurs charges, even if it is poweredoff.
*
*/
public Optional startCloudService() {
return Optional.ofNullable(this.startCloudService);
}
/**
* @return The unique identifier for the cloud service.
*
*/
public String uniqueId() {
return this.uniqueId;
}
/**
* @return Update mode for the cloud service. Role instances are allocated to update domains when the service is deployed. Updates can be initiated manually in each update domain or initiated automatically in all update domains.
* Possible Values are <br /><br />**Auto**<br /><br />**Manual** <br /><br />**Simultaneous**<br /><br />
* If not specified, the default value is Auto. If set to Manual, PUT UpdateDomain must be called to apply the update. If set to Auto, the update is automatically applied to each update domain in sequence.
*
*/
public Optional upgradeMode() {
return Optional.ofNullable(this.upgradeMode);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(CloudServicePropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean allowModelOverride;
private @Nullable String configuration;
private @Nullable String configurationUrl;
private @Nullable CloudServiceExtensionProfileResponse extensionProfile;
private @Nullable CloudServiceNetworkProfileResponse networkProfile;
private @Nullable CloudServiceOsProfileResponse osProfile;
private @Nullable String packageUrl;
private String provisioningState;
private @Nullable CloudServiceRoleProfileResponse roleProfile;
private @Nullable Boolean startCloudService;
private String uniqueId;
private @Nullable String upgradeMode;
public Builder() {}
public Builder(CloudServicePropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.allowModelOverride = defaults.allowModelOverride;
this.configuration = defaults.configuration;
this.configurationUrl = defaults.configurationUrl;
this.extensionProfile = defaults.extensionProfile;
this.networkProfile = defaults.networkProfile;
this.osProfile = defaults.osProfile;
this.packageUrl = defaults.packageUrl;
this.provisioningState = defaults.provisioningState;
this.roleProfile = defaults.roleProfile;
this.startCloudService = defaults.startCloudService;
this.uniqueId = defaults.uniqueId;
this.upgradeMode = defaults.upgradeMode;
}
@CustomType.Setter
public Builder allowModelOverride(@Nullable Boolean allowModelOverride) {
this.allowModelOverride = allowModelOverride;
return this;
}
@CustomType.Setter
public Builder configuration(@Nullable String configuration) {
this.configuration = configuration;
return this;
}
@CustomType.Setter
public Builder configurationUrl(@Nullable String configurationUrl) {
this.configurationUrl = configurationUrl;
return this;
}
@CustomType.Setter
public Builder extensionProfile(@Nullable CloudServiceExtensionProfileResponse extensionProfile) {
this.extensionProfile = extensionProfile;
return this;
}
@CustomType.Setter
public Builder networkProfile(@Nullable CloudServiceNetworkProfileResponse networkProfile) {
this.networkProfile = networkProfile;
return this;
}
@CustomType.Setter
public Builder osProfile(@Nullable CloudServiceOsProfileResponse osProfile) {
this.osProfile = osProfile;
return this;
}
@CustomType.Setter
public Builder packageUrl(@Nullable String packageUrl) {
this.packageUrl = packageUrl;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("CloudServicePropertiesResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder roleProfile(@Nullable CloudServiceRoleProfileResponse roleProfile) {
this.roleProfile = roleProfile;
return this;
}
@CustomType.Setter
public Builder startCloudService(@Nullable Boolean startCloudService) {
this.startCloudService = startCloudService;
return this;
}
@CustomType.Setter
public Builder uniqueId(String uniqueId) {
if (uniqueId == null) {
throw new MissingRequiredPropertyException("CloudServicePropertiesResponse", "uniqueId");
}
this.uniqueId = uniqueId;
return this;
}
@CustomType.Setter
public Builder upgradeMode(@Nullable String upgradeMode) {
this.upgradeMode = upgradeMode;
return this;
}
public CloudServicePropertiesResponse build() {
final var _resultValue = new CloudServicePropertiesResponse();
_resultValue.allowModelOverride = allowModelOverride;
_resultValue.configuration = configuration;
_resultValue.configurationUrl = configurationUrl;
_resultValue.extensionProfile = extensionProfile;
_resultValue.networkProfile = networkProfile;
_resultValue.osProfile = osProfile;
_resultValue.packageUrl = packageUrl;
_resultValue.provisioningState = provisioningState;
_resultValue.roleProfile = roleProfile;
_resultValue.startCloudService = startCloudService;
_resultValue.uniqueId = uniqueId;
_resultValue.upgradeMode = upgradeMode;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy