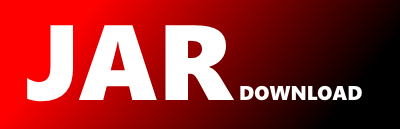
com.pulumi.azurenative.compute.outputs.GetImageResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute.outputs;
import com.pulumi.azurenative.compute.outputs.ExtendedLocationResponse;
import com.pulumi.azurenative.compute.outputs.ImageStorageProfileResponse;
import com.pulumi.azurenative.compute.outputs.SubResourceResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetImageResult {
/**
* @return The extended location of the Image.
*
*/
private @Nullable ExtendedLocationResponse extendedLocation;
/**
* @return Specifies the HyperVGenerationType of the VirtualMachine created from the image. From API Version 2019-03-01 if the image source is a blob, then we need the user to specify the value, if the source is managed resource like disk or snapshot, we may require the user to specify the property if we cannot deduce it from the source managed resource.
*
*/
private @Nullable String hyperVGeneration;
/**
* @return Resource Id
*
*/
private String id;
/**
* @return Resource location
*
*/
private String location;
/**
* @return Resource name
*
*/
private String name;
/**
* @return The provisioning state.
*
*/
private String provisioningState;
/**
* @return The source virtual machine from which Image is created.
*
*/
private @Nullable SubResourceResponse sourceVirtualMachine;
/**
* @return Specifies the storage settings for the virtual machine disks.
*
*/
private @Nullable ImageStorageProfileResponse storageProfile;
/**
* @return Resource tags
*
*/
private @Nullable Map tags;
/**
* @return Resource type
*
*/
private String type;
private GetImageResult() {}
/**
* @return The extended location of the Image.
*
*/
public Optional extendedLocation() {
return Optional.ofNullable(this.extendedLocation);
}
/**
* @return Specifies the HyperVGenerationType of the VirtualMachine created from the image. From API Version 2019-03-01 if the image source is a blob, then we need the user to specify the value, if the source is managed resource like disk or snapshot, we may require the user to specify the property if we cannot deduce it from the source managed resource.
*
*/
public Optional hyperVGeneration() {
return Optional.ofNullable(this.hyperVGeneration);
}
/**
* @return Resource Id
*
*/
public String id() {
return this.id;
}
/**
* @return Resource location
*
*/
public String location() {
return this.location;
}
/**
* @return Resource name
*
*/
public String name() {
return this.name;
}
/**
* @return The provisioning state.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The source virtual machine from which Image is created.
*
*/
public Optional sourceVirtualMachine() {
return Optional.ofNullable(this.sourceVirtualMachine);
}
/**
* @return Specifies the storage settings for the virtual machine disks.
*
*/
public Optional storageProfile() {
return Optional.ofNullable(this.storageProfile);
}
/**
* @return Resource tags
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Resource type
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetImageResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable ExtendedLocationResponse extendedLocation;
private @Nullable String hyperVGeneration;
private String id;
private String location;
private String name;
private String provisioningState;
private @Nullable SubResourceResponse sourceVirtualMachine;
private @Nullable ImageStorageProfileResponse storageProfile;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetImageResult defaults) {
Objects.requireNonNull(defaults);
this.extendedLocation = defaults.extendedLocation;
this.hyperVGeneration = defaults.hyperVGeneration;
this.id = defaults.id;
this.location = defaults.location;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.sourceVirtualMachine = defaults.sourceVirtualMachine;
this.storageProfile = defaults.storageProfile;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder extendedLocation(@Nullable ExtendedLocationResponse extendedLocation) {
this.extendedLocation = extendedLocation;
return this;
}
@CustomType.Setter
public Builder hyperVGeneration(@Nullable String hyperVGeneration) {
this.hyperVGeneration = hyperVGeneration;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetImageResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetImageResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetImageResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetImageResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder sourceVirtualMachine(@Nullable SubResourceResponse sourceVirtualMachine) {
this.sourceVirtualMachine = sourceVirtualMachine;
return this;
}
@CustomType.Setter
public Builder storageProfile(@Nullable ImageStorageProfileResponse storageProfile) {
this.storageProfile = storageProfile;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetImageResult", "type");
}
this.type = type;
return this;
}
public GetImageResult build() {
final var _resultValue = new GetImageResult();
_resultValue.extendedLocation = extendedLocation;
_resultValue.hyperVGeneration = hyperVGeneration;
_resultValue.id = id;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.sourceVirtualMachine = sourceVirtualMachine;
_resultValue.storageProfile = storageProfile;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy