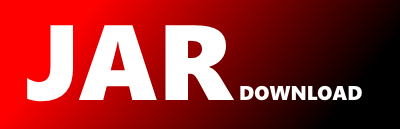
com.pulumi.azurenative.consumption.outputs.GetBudgetResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.consumption.outputs;
import com.pulumi.azurenative.consumption.outputs.BudgetFilterResponse;
import com.pulumi.azurenative.consumption.outputs.BudgetTimePeriodResponse;
import com.pulumi.azurenative.consumption.outputs.CurrentSpendResponse;
import com.pulumi.azurenative.consumption.outputs.ForecastSpendResponse;
import com.pulumi.azurenative.consumption.outputs.NotificationResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetBudgetResult {
/**
* @return The total amount of cost to track with the budget
*
*/
private Double amount;
/**
* @return The category of the budget, whether the budget tracks cost or usage.
*
*/
private String category;
/**
* @return The current amount of cost which is being tracked for a budget.
*
*/
private CurrentSpendResponse currentSpend;
/**
* @return eTag of the resource. To handle concurrent update scenario, this field will be used to determine whether the user is updating the latest version or not.
*
*/
private @Nullable String eTag;
/**
* @return May be used to filter budgets by user-specified dimensions and/or tags.
*
*/
private @Nullable BudgetFilterResponse filter;
/**
* @return The forecasted cost which is being tracked for a budget.
*
*/
private ForecastSpendResponse forecastSpend;
/**
* @return Resource Id.
*
*/
private String id;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return Dictionary of notifications associated with the budget. Budget can have up to five notifications.
*
*/
private @Nullable Map notifications;
/**
* @return The time covered by a budget. Tracking of the amount will be reset based on the time grain. BillingMonth, BillingQuarter, and BillingAnnual are only supported by WD customers
*
*/
private String timeGrain;
/**
* @return Has start and end date of the budget. The start date must be first of the month and should be less than the end date. Budget start date must be on or after June 1, 2017. Future start date should not be more than twelve months. Past start date should be selected within the timegrain period. There are no restrictions on the end date.
*
*/
private BudgetTimePeriodResponse timePeriod;
/**
* @return Resource type.
*
*/
private String type;
private GetBudgetResult() {}
/**
* @return The total amount of cost to track with the budget
*
*/
public Double amount() {
return this.amount;
}
/**
* @return The category of the budget, whether the budget tracks cost or usage.
*
*/
public String category() {
return this.category;
}
/**
* @return The current amount of cost which is being tracked for a budget.
*
*/
public CurrentSpendResponse currentSpend() {
return this.currentSpend;
}
/**
* @return eTag of the resource. To handle concurrent update scenario, this field will be used to determine whether the user is updating the latest version or not.
*
*/
public Optional eTag() {
return Optional.ofNullable(this.eTag);
}
/**
* @return May be used to filter budgets by user-specified dimensions and/or tags.
*
*/
public Optional filter() {
return Optional.ofNullable(this.filter);
}
/**
* @return The forecasted cost which is being tracked for a budget.
*
*/
public ForecastSpendResponse forecastSpend() {
return this.forecastSpend;
}
/**
* @return Resource Id.
*
*/
public String id() {
return this.id;
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return Dictionary of notifications associated with the budget. Budget can have up to five notifications.
*
*/
public Map notifications() {
return this.notifications == null ? Map.of() : this.notifications;
}
/**
* @return The time covered by a budget. Tracking of the amount will be reset based on the time grain. BillingMonth, BillingQuarter, and BillingAnnual are only supported by WD customers
*
*/
public String timeGrain() {
return this.timeGrain;
}
/**
* @return Has start and end date of the budget. The start date must be first of the month and should be less than the end date. Budget start date must be on or after June 1, 2017. Future start date should not be more than twelve months. Past start date should be selected within the timegrain period. There are no restrictions on the end date.
*
*/
public BudgetTimePeriodResponse timePeriod() {
return this.timePeriod;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetBudgetResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Double amount;
private String category;
private CurrentSpendResponse currentSpend;
private @Nullable String eTag;
private @Nullable BudgetFilterResponse filter;
private ForecastSpendResponse forecastSpend;
private String id;
private String name;
private @Nullable Map notifications;
private String timeGrain;
private BudgetTimePeriodResponse timePeriod;
private String type;
public Builder() {}
public Builder(GetBudgetResult defaults) {
Objects.requireNonNull(defaults);
this.amount = defaults.amount;
this.category = defaults.category;
this.currentSpend = defaults.currentSpend;
this.eTag = defaults.eTag;
this.filter = defaults.filter;
this.forecastSpend = defaults.forecastSpend;
this.id = defaults.id;
this.name = defaults.name;
this.notifications = defaults.notifications;
this.timeGrain = defaults.timeGrain;
this.timePeriod = defaults.timePeriod;
this.type = defaults.type;
}
@CustomType.Setter
public Builder amount(Double amount) {
if (amount == null) {
throw new MissingRequiredPropertyException("GetBudgetResult", "amount");
}
this.amount = amount;
return this;
}
@CustomType.Setter
public Builder category(String category) {
if (category == null) {
throw new MissingRequiredPropertyException("GetBudgetResult", "category");
}
this.category = category;
return this;
}
@CustomType.Setter
public Builder currentSpend(CurrentSpendResponse currentSpend) {
if (currentSpend == null) {
throw new MissingRequiredPropertyException("GetBudgetResult", "currentSpend");
}
this.currentSpend = currentSpend;
return this;
}
@CustomType.Setter
public Builder eTag(@Nullable String eTag) {
this.eTag = eTag;
return this;
}
@CustomType.Setter
public Builder filter(@Nullable BudgetFilterResponse filter) {
this.filter = filter;
return this;
}
@CustomType.Setter
public Builder forecastSpend(ForecastSpendResponse forecastSpend) {
if (forecastSpend == null) {
throw new MissingRequiredPropertyException("GetBudgetResult", "forecastSpend");
}
this.forecastSpend = forecastSpend;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetBudgetResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetBudgetResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder notifications(@Nullable Map notifications) {
this.notifications = notifications;
return this;
}
@CustomType.Setter
public Builder timeGrain(String timeGrain) {
if (timeGrain == null) {
throw new MissingRequiredPropertyException("GetBudgetResult", "timeGrain");
}
this.timeGrain = timeGrain;
return this;
}
@CustomType.Setter
public Builder timePeriod(BudgetTimePeriodResponse timePeriod) {
if (timePeriod == null) {
throw new MissingRequiredPropertyException("GetBudgetResult", "timePeriod");
}
this.timePeriod = timePeriod;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetBudgetResult", "type");
}
this.type = type;
return this;
}
public GetBudgetResult build() {
final var _resultValue = new GetBudgetResult();
_resultValue.amount = amount;
_resultValue.category = category;
_resultValue.currentSpend = currentSpend;
_resultValue.eTag = eTag;
_resultValue.filter = filter;
_resultValue.forecastSpend = forecastSpend;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.notifications = notifications;
_resultValue.timeGrain = timeGrain;
_resultValue.timePeriod = timePeriod;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy