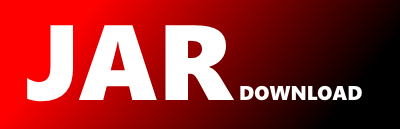
com.pulumi.azurenative.containerregistry.inputs.OverrideTaskStepPropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.containerregistry.inputs;
import com.pulumi.azurenative.containerregistry.inputs.ArgumentArgs;
import com.pulumi.azurenative.containerregistry.inputs.SetValueArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class OverrideTaskStepPropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final OverrideTaskStepPropertiesArgs Empty = new OverrideTaskStepPropertiesArgs();
/**
* Gets or sets the collection of override arguments to be used when
* executing a build step.
*
*/
@Import(name="arguments")
private @Nullable Output> arguments;
/**
* @return Gets or sets the collection of override arguments to be used when
* executing a build step.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy