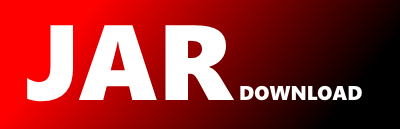
com.pulumi.azurenative.containerservice.AgentPool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.containerservice;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.containerservice.AgentPoolArgs;
import com.pulumi.azurenative.containerservice.outputs.AgentPoolUpgradeSettingsResponse;
import com.pulumi.azurenative.containerservice.outputs.CreationDataResponse;
import com.pulumi.azurenative.containerservice.outputs.KubeletConfigResponse;
import com.pulumi.azurenative.containerservice.outputs.LinuxOSConfigResponse;
import com.pulumi.azurenative.containerservice.outputs.PowerStateResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Agent Pool.
* Azure REST API version: 2023-04-01. Prior API version in Azure Native 1.x: 2021-03-01.
*
* Other available API versions: 2019-02-01, 2019-04-01, 2020-06-01, 2021-02-01, 2021-08-01, 2022-04-02-preview, 2023-05-02-preview, 2023-06-01, 2023-06-02-preview, 2023-07-01, 2023-07-02-preview, 2023-08-01, 2023-08-02-preview, 2023-09-01, 2023-09-02-preview, 2023-10-01, 2023-10-02-preview, 2023-11-01, 2023-11-02-preview, 2024-01-01, 2024-01-02-preview, 2024-02-01, 2024-02-02-preview, 2024-03-02-preview, 2024-04-02-preview, 2024-05-01, 2024-05-02-preview, 2024-06-02-preview, 2024-07-01, 2024-07-02-preview, 2024-08-01.
*
* ## Example Usage
* ### Create Agent Pool using an agent pool snapshot
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.AgentPool;
* import com.pulumi.azurenative.containerservice.AgentPoolArgs;
* import com.pulumi.azurenative.containerservice.inputs.CreationDataArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("agentpool1")
* .count(3)
* .creationData(CreationDataArgs.builder()
* .sourceResourceId("/subscriptions/subid1/resourceGroups/rg1/providers/Microsoft.ContainerService/snapshots/snapshot1")
* .build())
* .enableFIPS(true)
* .orchestratorVersion("")
* .osType("Linux")
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .vmSize("Standard_DS2_v2")
* .build());
*
* }
* }
*
* }
*
* ### Create Agent Pool with Dedicated Host Group
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.AgentPool;
* import com.pulumi.azurenative.containerservice.AgentPoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("agentpool1")
* .count(3)
* .hostGroupID("/subscriptions/subid1/resourcegroups/rg/providers/Microsoft.Compute/hostGroups/hostgroup1")
* .orchestratorVersion("")
* .osType("Linux")
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .vmSize("Standard_DS2_v2")
* .build());
*
* }
* }
*
* }
*
* ### Create Agent Pool with EncryptionAtHost enabled
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.AgentPool;
* import com.pulumi.azurenative.containerservice.AgentPoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("agentpool1")
* .count(3)
* .enableEncryptionAtHost(true)
* .orchestratorVersion("")
* .osType("Linux")
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .vmSize("Standard_DS2_v2")
* .build());
*
* }
* }
*
* }
*
* ### Create Agent Pool with Ephemeral OS Disk
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.AgentPool;
* import com.pulumi.azurenative.containerservice.AgentPoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("agentpool1")
* .count(3)
* .orchestratorVersion("")
* .osDiskSizeGB(64)
* .osDiskType("Ephemeral")
* .osType("Linux")
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .vmSize("Standard_DS2_v2")
* .build());
*
* }
* }
*
* }
*
* ### Create Agent Pool with FIPS enabled OS
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.AgentPool;
* import com.pulumi.azurenative.containerservice.AgentPoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("agentpool1")
* .count(3)
* .enableFIPS(true)
* .orchestratorVersion("")
* .osType("Linux")
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .vmSize("Standard_DS2_v2")
* .build());
*
* }
* }
*
* }
*
* ### Create Agent Pool with GPUMIG
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.AgentPool;
* import com.pulumi.azurenative.containerservice.AgentPoolArgs;
* import com.pulumi.azurenative.containerservice.inputs.KubeletConfigArgs;
* import com.pulumi.azurenative.containerservice.inputs.LinuxOSConfigArgs;
* import com.pulumi.azurenative.containerservice.inputs.SysctlConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("agentpool1")
* .count(3)
* .gpuInstanceProfile("MIG2g")
* .kubeletConfig(KubeletConfigArgs.builder()
* .allowedUnsafeSysctls(
* "kernel.msg*",
* "net.core.somaxconn")
* .cpuCfsQuota(true)
* .cpuCfsQuotaPeriod("200ms")
* .cpuManagerPolicy("static")
* .failSwapOn(false)
* .imageGcHighThreshold(90)
* .imageGcLowThreshold(70)
* .topologyManagerPolicy("best-effort")
* .build())
* .linuxOSConfig(LinuxOSConfigArgs.builder()
* .swapFileSizeMB(1500)
* .sysctls(SysctlConfigArgs.builder()
* .kernelThreadsMax(99999)
* .netCoreWmemDefault(12345)
* .netIpv4IpLocalPortRange("20000 60000")
* .netIpv4TcpTwReuse(true)
* .build())
* .transparentHugePageDefrag("madvise")
* .transparentHugePageEnabled("always")
* .build())
* .orchestratorVersion("")
* .osType("Linux")
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .vmSize("Standard_ND96asr_v4")
* .build());
*
* }
* }
*
* }
*
* ### Create Agent Pool with Krustlet and the WASI runtime
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.AgentPool;
* import com.pulumi.azurenative.containerservice.AgentPoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("agentpool1")
* .count(3)
* .mode("User")
* .orchestratorVersion("")
* .osDiskSizeGB(64)
* .osType("Linux")
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .vmSize("Standard_DS2_v2")
* .workloadRuntime("WasmWasi")
* .build());
*
* }
* }
*
* }
*
* ### Create Agent Pool with KubeletConfig and LinuxOSConfig
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.AgentPool;
* import com.pulumi.azurenative.containerservice.AgentPoolArgs;
* import com.pulumi.azurenative.containerservice.inputs.KubeletConfigArgs;
* import com.pulumi.azurenative.containerservice.inputs.LinuxOSConfigArgs;
* import com.pulumi.azurenative.containerservice.inputs.SysctlConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("agentpool1")
* .count(3)
* .kubeletConfig(KubeletConfigArgs.builder()
* .allowedUnsafeSysctls(
* "kernel.msg*",
* "net.core.somaxconn")
* .cpuCfsQuota(true)
* .cpuCfsQuotaPeriod("200ms")
* .cpuManagerPolicy("static")
* .failSwapOn(false)
* .imageGcHighThreshold(90)
* .imageGcLowThreshold(70)
* .topologyManagerPolicy("best-effort")
* .build())
* .linuxOSConfig(LinuxOSConfigArgs.builder()
* .swapFileSizeMB(1500)
* .sysctls(SysctlConfigArgs.builder()
* .kernelThreadsMax(99999)
* .netCoreWmemDefault(12345)
* .netIpv4IpLocalPortRange("20000 60000")
* .netIpv4TcpTwReuse(true)
* .build())
* .transparentHugePageDefrag("madvise")
* .transparentHugePageEnabled("always")
* .build())
* .orchestratorVersion("")
* .osType("Linux")
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .vmSize("Standard_DS2_v2")
* .build());
*
* }
* }
*
* }
*
* ### Create Agent Pool with OSSKU
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.AgentPool;
* import com.pulumi.azurenative.containerservice.AgentPoolArgs;
* import com.pulumi.azurenative.containerservice.inputs.KubeletConfigArgs;
* import com.pulumi.azurenative.containerservice.inputs.LinuxOSConfigArgs;
* import com.pulumi.azurenative.containerservice.inputs.SysctlConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("agentpool1")
* .count(3)
* .kubeletConfig(KubeletConfigArgs.builder()
* .allowedUnsafeSysctls(
* "kernel.msg*",
* "net.core.somaxconn")
* .cpuCfsQuota(true)
* .cpuCfsQuotaPeriod("200ms")
* .cpuManagerPolicy("static")
* .failSwapOn(false)
* .imageGcHighThreshold(90)
* .imageGcLowThreshold(70)
* .topologyManagerPolicy("best-effort")
* .build())
* .linuxOSConfig(LinuxOSConfigArgs.builder()
* .swapFileSizeMB(1500)
* .sysctls(SysctlConfigArgs.builder()
* .kernelThreadsMax(99999)
* .netCoreWmemDefault(12345)
* .netIpv4IpLocalPortRange("20000 60000")
* .netIpv4TcpTwReuse(true)
* .build())
* .transparentHugePageDefrag("madvise")
* .transparentHugePageEnabled("always")
* .build())
* .orchestratorVersion("")
* .osSKU("AzureLinux")
* .osType("Linux")
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .vmSize("Standard_DS2_v2")
* .build());
*
* }
* }
*
* }
*
* ### Create Agent Pool with PPG
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.AgentPool;
* import com.pulumi.azurenative.containerservice.AgentPoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("agentpool1")
* .count(3)
* .orchestratorVersion("")
* .osType("Linux")
* .proximityPlacementGroupID("/subscriptions/subid1/resourcegroups/rg1/providers/Microsoft.Compute/proximityPlacementGroups/ppg1")
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .vmSize("Standard_DS2_v2")
* .build());
*
* }
* }
*
* }
*
* ### Create Agent Pool with UltraSSD enabled
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.AgentPool;
* import com.pulumi.azurenative.containerservice.AgentPoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("agentpool1")
* .count(3)
* .enableUltraSSD(true)
* .orchestratorVersion("")
* .osType("Linux")
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .vmSize("Standard_DS2_v2")
* .build());
*
* }
* }
*
* }
*
* ### Create Agent Pool with Windows OSSKU
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.AgentPool;
* import com.pulumi.azurenative.containerservice.AgentPoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("wnp2")
* .count(3)
* .orchestratorVersion("1.23.3")
* .osSKU("Windows2022")
* .osType("Windows")
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .vmSize("Standard_D4s_v3")
* .build());
*
* }
* }
*
* }
*
* ### Create Spot Agent Pool
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.AgentPool;
* import com.pulumi.azurenative.containerservice.AgentPoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("agentpool1")
* .count(3)
* .nodeLabels(Map.of("key1", "val1"))
* .nodeTaints("Key1=Value1:NoSchedule")
* .orchestratorVersion("")
* .osType("Linux")
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .scaleSetEvictionPolicy("Delete")
* .scaleSetPriority("Spot")
* .tags(Map.of("name1", "val1"))
* .vmSize("Standard_DS1_v2")
* .build());
*
* }
* }
*
* }
*
* ### Create/Update Agent Pool
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.AgentPool;
* import com.pulumi.azurenative.containerservice.AgentPoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("agentpool1")
* .count(3)
* .mode("User")
* .nodeLabels(Map.of("key1", "val1"))
* .nodeTaints("Key1=Value1:NoSchedule")
* .orchestratorVersion("")
* .osType("Linux")
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .scaleSetEvictionPolicy("Delete")
* .scaleSetPriority("Spot")
* .tags(Map.of("name1", "val1"))
* .vmSize("Standard_DS1_v2")
* .build());
*
* }
* }
*
* }
*
* ### Start Agent Pool
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.AgentPool;
* import com.pulumi.azurenative.containerservice.AgentPoolArgs;
* import com.pulumi.azurenative.containerservice.inputs.PowerStateArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("agentpool1")
* .powerState(PowerStateArgs.builder()
* .code("Running")
* .build())
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .build());
*
* }
* }
*
* }
*
* ### Stop Agent Pool
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.AgentPool;
* import com.pulumi.azurenative.containerservice.AgentPoolArgs;
* import com.pulumi.azurenative.containerservice.inputs.PowerStateArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("agentpool1")
* .powerState(PowerStateArgs.builder()
* .code("Stopped")
* .build())
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .build());
*
* }
* }
*
* }
*
* ### Update Agent Pool
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.AgentPool;
* import com.pulumi.azurenative.containerservice.AgentPoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("agentpool1")
* .count(3)
* .enableAutoScaling(true)
* .maxCount(2)
* .minCount(2)
* .nodeTaints("Key1=Value1:NoSchedule")
* .orchestratorVersion("")
* .osType("Linux")
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .scaleSetEvictionPolicy("Delete")
* .scaleSetPriority("Spot")
* .vmSize("Standard_DS1_v2")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:containerservice:AgentPool agentpool1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ContainerService/managedClusters/{resourceName}/agentPools/{agentPoolName}
* ```
*
*/
@ResourceType(type="azure-native:containerservice:AgentPool")
public class AgentPool extends com.pulumi.resources.CustomResource {
/**
* The list of Availability zones to use for nodes. This can only be specified if the AgentPoolType property is 'VirtualMachineScaleSets'.
*
*/
@Export(name="availabilityZones", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> availabilityZones;
/**
* @return The list of Availability zones to use for nodes. This can only be specified if the AgentPoolType property is 'VirtualMachineScaleSets'.
*
*/
public Output>> availabilityZones() {
return Codegen.optional(this.availabilityZones);
}
/**
* Number of agents (VMs) to host docker containers. Allowed values must be in the range of 0 to 1000 (inclusive) for user pools and in the range of 1 to 1000 (inclusive) for system pools. The default value is 1.
*
*/
@Export(name="count", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> count;
/**
* @return Number of agents (VMs) to host docker containers. Allowed values must be in the range of 0 to 1000 (inclusive) for user pools and in the range of 1 to 1000 (inclusive) for system pools. The default value is 1.
*
*/
public Output> count() {
return Codegen.optional(this.count);
}
/**
* CreationData to be used to specify the source Snapshot ID if the node pool will be created/upgraded using a snapshot.
*
*/
@Export(name="creationData", refs={CreationDataResponse.class}, tree="[0]")
private Output* @Nullable */ CreationDataResponse> creationData;
/**
* @return CreationData to be used to specify the source Snapshot ID if the node pool will be created/upgraded using a snapshot.
*
*/
public Output> creationData() {
return Codegen.optional(this.creationData);
}
/**
* If orchestratorVersion is a fully specified version <major.minor.patch>, this field will be exactly equal to it. If orchestratorVersion is <major.minor>, this field will contain the full <major.minor.patch> version being used.
*
*/
@Export(name="currentOrchestratorVersion", refs={String.class}, tree="[0]")
private Output currentOrchestratorVersion;
/**
* @return If orchestratorVersion is a fully specified version <major.minor.patch>, this field will be exactly equal to it. If orchestratorVersion is <major.minor>, this field will contain the full <major.minor.patch> version being used.
*
*/
public Output currentOrchestratorVersion() {
return this.currentOrchestratorVersion;
}
/**
* Whether to enable auto-scaler
*
*/
@Export(name="enableAutoScaling", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableAutoScaling;
/**
* @return Whether to enable auto-scaler
*
*/
public Output> enableAutoScaling() {
return Codegen.optional(this.enableAutoScaling);
}
/**
* This is only supported on certain VM sizes and in certain Azure regions. For more information, see: https://docs.microsoft.com/azure/aks/enable-host-encryption
*
*/
@Export(name="enableEncryptionAtHost", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableEncryptionAtHost;
/**
* @return This is only supported on certain VM sizes and in certain Azure regions. For more information, see: https://docs.microsoft.com/azure/aks/enable-host-encryption
*
*/
public Output> enableEncryptionAtHost() {
return Codegen.optional(this.enableEncryptionAtHost);
}
/**
* See [Add a FIPS-enabled node pool](https://docs.microsoft.com/azure/aks/use-multiple-node-pools#add-a-fips-enabled-node-pool-preview) for more details.
*
*/
@Export(name="enableFIPS", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableFIPS;
/**
* @return See [Add a FIPS-enabled node pool](https://docs.microsoft.com/azure/aks/use-multiple-node-pools#add-a-fips-enabled-node-pool-preview) for more details.
*
*/
public Output> enableFIPS() {
return Codegen.optional(this.enableFIPS);
}
/**
* Some scenarios may require nodes in a node pool to receive their own dedicated public IP addresses. A common scenario is for gaming workloads, where a console needs to make a direct connection to a cloud virtual machine to minimize hops. For more information see [assigning a public IP per node](https://docs.microsoft.com/azure/aks/use-multiple-node-pools#assign-a-public-ip-per-node-for-your-node-pools). The default is false.
*
*/
@Export(name="enableNodePublicIP", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableNodePublicIP;
/**
* @return Some scenarios may require nodes in a node pool to receive their own dedicated public IP addresses. A common scenario is for gaming workloads, where a console needs to make a direct connection to a cloud virtual machine to minimize hops. For more information see [assigning a public IP per node](https://docs.microsoft.com/azure/aks/use-multiple-node-pools#assign-a-public-ip-per-node-for-your-node-pools). The default is false.
*
*/
public Output> enableNodePublicIP() {
return Codegen.optional(this.enableNodePublicIP);
}
/**
* Whether to enable UltraSSD
*
*/
@Export(name="enableUltraSSD", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableUltraSSD;
/**
* @return Whether to enable UltraSSD
*
*/
public Output> enableUltraSSD() {
return Codegen.optional(this.enableUltraSSD);
}
/**
* GPUInstanceProfile to be used to specify GPU MIG instance profile for supported GPU VM SKU.
*
*/
@Export(name="gpuInstanceProfile", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> gpuInstanceProfile;
/**
* @return GPUInstanceProfile to be used to specify GPU MIG instance profile for supported GPU VM SKU.
*
*/
public Output> gpuInstanceProfile() {
return Codegen.optional(this.gpuInstanceProfile);
}
/**
* This is of the form: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/hostGroups/{hostGroupName}. For more information see [Azure dedicated hosts](https://docs.microsoft.com/azure/virtual-machines/dedicated-hosts).
*
*/
@Export(name="hostGroupID", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> hostGroupID;
/**
* @return This is of the form: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/hostGroups/{hostGroupName}. For more information see [Azure dedicated hosts](https://docs.microsoft.com/azure/virtual-machines/dedicated-hosts).
*
*/
public Output> hostGroupID() {
return Codegen.optional(this.hostGroupID);
}
/**
* The Kubelet configuration on the agent pool nodes.
*
*/
@Export(name="kubeletConfig", refs={KubeletConfigResponse.class}, tree="[0]")
private Output* @Nullable */ KubeletConfigResponse> kubeletConfig;
/**
* @return The Kubelet configuration on the agent pool nodes.
*
*/
public Output> kubeletConfig() {
return Codegen.optional(this.kubeletConfig);
}
/**
* Determines the placement of emptyDir volumes, container runtime data root, and Kubelet ephemeral storage.
*
*/
@Export(name="kubeletDiskType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> kubeletDiskType;
/**
* @return Determines the placement of emptyDir volumes, container runtime data root, and Kubelet ephemeral storage.
*
*/
public Output> kubeletDiskType() {
return Codegen.optional(this.kubeletDiskType);
}
/**
* The OS configuration of Linux agent nodes.
*
*/
@Export(name="linuxOSConfig", refs={LinuxOSConfigResponse.class}, tree="[0]")
private Output* @Nullable */ LinuxOSConfigResponse> linuxOSConfig;
/**
* @return The OS configuration of Linux agent nodes.
*
*/
public Output> linuxOSConfig() {
return Codegen.optional(this.linuxOSConfig);
}
/**
* The maximum number of nodes for auto-scaling
*
*/
@Export(name="maxCount", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> maxCount;
/**
* @return The maximum number of nodes for auto-scaling
*
*/
public Output> maxCount() {
return Codegen.optional(this.maxCount);
}
/**
* The maximum number of pods that can run on a node.
*
*/
@Export(name="maxPods", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> maxPods;
/**
* @return The maximum number of pods that can run on a node.
*
*/
public Output> maxPods() {
return Codegen.optional(this.maxPods);
}
/**
* The minimum number of nodes for auto-scaling
*
*/
@Export(name="minCount", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> minCount;
/**
* @return The minimum number of nodes for auto-scaling
*
*/
public Output> minCount() {
return Codegen.optional(this.minCount);
}
/**
* A cluster must have at least one 'System' Agent Pool at all times. For additional information on agent pool restrictions and best practices, see: https://docs.microsoft.com/azure/aks/use-system-pools
*
*/
@Export(name="mode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> mode;
/**
* @return A cluster must have at least one 'System' Agent Pool at all times. For additional information on agent pool restrictions and best practices, see: https://docs.microsoft.com/azure/aks/use-system-pools
*
*/
public Output> mode() {
return Codegen.optional(this.mode);
}
/**
* The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
public Output name() {
return this.name;
}
/**
* The version of node image
*
*/
@Export(name="nodeImageVersion", refs={String.class}, tree="[0]")
private Output nodeImageVersion;
/**
* @return The version of node image
*
*/
public Output nodeImageVersion() {
return this.nodeImageVersion;
}
/**
* The node labels to be persisted across all nodes in agent pool.
*
*/
@Export(name="nodeLabels", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> nodeLabels;
/**
* @return The node labels to be persisted across all nodes in agent pool.
*
*/
public Output>> nodeLabels() {
return Codegen.optional(this.nodeLabels);
}
/**
* This is of the form: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/publicIPPrefixes/{publicIPPrefixName}
*
*/
@Export(name="nodePublicIPPrefixID", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> nodePublicIPPrefixID;
/**
* @return This is of the form: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/publicIPPrefixes/{publicIPPrefixName}
*
*/
public Output> nodePublicIPPrefixID() {
return Codegen.optional(this.nodePublicIPPrefixID);
}
/**
* The taints added to new nodes during node pool create and scale. For example, key=value:NoSchedule.
*
*/
@Export(name="nodeTaints", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> nodeTaints;
/**
* @return The taints added to new nodes during node pool create and scale. For example, key=value:NoSchedule.
*
*/
public Output>> nodeTaints() {
return Codegen.optional(this.nodeTaints);
}
/**
* Both patch version <major.minor.patch> (e.g. 1.20.13) and <major.minor> (e.g. 1.20) are supported. When <major.minor> is specified, the latest supported GA patch version is chosen automatically. Updating the cluster with the same <major.minor> once it has been created (e.g. 1.14.x -> 1.14) will not trigger an upgrade, even if a newer patch version is available. As a best practice, you should upgrade all node pools in an AKS cluster to the same Kubernetes version. The node pool version must have the same major version as the control plane. The node pool minor version must be within two minor versions of the control plane version. The node pool version cannot be greater than the control plane version. For more information see [upgrading a node pool](https://docs.microsoft.com/azure/aks/use-multiple-node-pools#upgrade-a-node-pool).
*
*/
@Export(name="orchestratorVersion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> orchestratorVersion;
/**
* @return Both patch version <major.minor.patch> (e.g. 1.20.13) and <major.minor> (e.g. 1.20) are supported. When <major.minor> is specified, the latest supported GA patch version is chosen automatically. Updating the cluster with the same <major.minor> once it has been created (e.g. 1.14.x -> 1.14) will not trigger an upgrade, even if a newer patch version is available. As a best practice, you should upgrade all node pools in an AKS cluster to the same Kubernetes version. The node pool version must have the same major version as the control plane. The node pool minor version must be within two minor versions of the control plane version. The node pool version cannot be greater than the control plane version. For more information see [upgrading a node pool](https://docs.microsoft.com/azure/aks/use-multiple-node-pools#upgrade-a-node-pool).
*
*/
public Output> orchestratorVersion() {
return Codegen.optional(this.orchestratorVersion);
}
/**
* OS Disk Size in GB to be used to specify the disk size for every machine in the master/agent pool. If you specify 0, it will apply the default osDisk size according to the vmSize specified.
*
*/
@Export(name="osDiskSizeGB", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> osDiskSizeGB;
/**
* @return OS Disk Size in GB to be used to specify the disk size for every machine in the master/agent pool. If you specify 0, it will apply the default osDisk size according to the vmSize specified.
*
*/
public Output> osDiskSizeGB() {
return Codegen.optional(this.osDiskSizeGB);
}
/**
* The default is 'Ephemeral' if the VM supports it and has a cache disk larger than the requested OSDiskSizeGB. Otherwise, defaults to 'Managed'. May not be changed after creation. For more information see [Ephemeral OS](https://docs.microsoft.com/azure/aks/cluster-configuration#ephemeral-os).
*
*/
@Export(name="osDiskType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> osDiskType;
/**
* @return The default is 'Ephemeral' if the VM supports it and has a cache disk larger than the requested OSDiskSizeGB. Otherwise, defaults to 'Managed'. May not be changed after creation. For more information see [Ephemeral OS](https://docs.microsoft.com/azure/aks/cluster-configuration#ephemeral-os).
*
*/
public Output> osDiskType() {
return Codegen.optional(this.osDiskType);
}
/**
* Specifies the OS SKU used by the agent pool. The default is Ubuntu if OSType is Linux. The default is Windows2019 when Kubernetes <= 1.24 or Windows2022 when Kubernetes >= 1.25 if OSType is Windows.
*
*/
@Export(name="osSKU", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> osSKU;
/**
* @return Specifies the OS SKU used by the agent pool. The default is Ubuntu if OSType is Linux. The default is Windows2019 when Kubernetes <= 1.24 or Windows2022 when Kubernetes >= 1.25 if OSType is Windows.
*
*/
public Output> osSKU() {
return Codegen.optional(this.osSKU);
}
/**
* The operating system type. The default is Linux.
*
*/
@Export(name="osType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> osType;
/**
* @return The operating system type. The default is Linux.
*
*/
public Output> osType() {
return Codegen.optional(this.osType);
}
/**
* If omitted, pod IPs are statically assigned on the node subnet (see vnetSubnetID for more details). This is of the form: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/virtualNetworks/{virtualNetworkName}/subnets/{subnetName}
*
*/
@Export(name="podSubnetID", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> podSubnetID;
/**
* @return If omitted, pod IPs are statically assigned on the node subnet (see vnetSubnetID for more details). This is of the form: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/virtualNetworks/{virtualNetworkName}/subnets/{subnetName}
*
*/
public Output> podSubnetID() {
return Codegen.optional(this.podSubnetID);
}
/**
* When an Agent Pool is first created it is initially Running. The Agent Pool can be stopped by setting this field to Stopped. A stopped Agent Pool stops all of its VMs and does not accrue billing charges. An Agent Pool can only be stopped if it is Running and provisioning state is Succeeded
*
*/
@Export(name="powerState", refs={PowerStateResponse.class}, tree="[0]")
private Output* @Nullable */ PowerStateResponse> powerState;
/**
* @return When an Agent Pool is first created it is initially Running. The Agent Pool can be stopped by setting this field to Stopped. A stopped Agent Pool stops all of its VMs and does not accrue billing charges. An Agent Pool can only be stopped if it is Running and provisioning state is Succeeded
*
*/
public Output> powerState() {
return Codegen.optional(this.powerState);
}
/**
* The current deployment or provisioning state.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The current deployment or provisioning state.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* The ID for Proximity Placement Group.
*
*/
@Export(name="proximityPlacementGroupID", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> proximityPlacementGroupID;
/**
* @return The ID for Proximity Placement Group.
*
*/
public Output> proximityPlacementGroupID() {
return Codegen.optional(this.proximityPlacementGroupID);
}
/**
* This also effects the cluster autoscaler behavior. If not specified, it defaults to Delete.
*
*/
@Export(name="scaleDownMode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> scaleDownMode;
/**
* @return This also effects the cluster autoscaler behavior. If not specified, it defaults to Delete.
*
*/
public Output> scaleDownMode() {
return Codegen.optional(this.scaleDownMode);
}
/**
* This cannot be specified unless the scaleSetPriority is 'Spot'. If not specified, the default is 'Delete'.
*
*/
@Export(name="scaleSetEvictionPolicy", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> scaleSetEvictionPolicy;
/**
* @return This cannot be specified unless the scaleSetPriority is 'Spot'. If not specified, the default is 'Delete'.
*
*/
public Output> scaleSetEvictionPolicy() {
return Codegen.optional(this.scaleSetEvictionPolicy);
}
/**
* The Virtual Machine Scale Set priority. If not specified, the default is 'Regular'.
*
*/
@Export(name="scaleSetPriority", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> scaleSetPriority;
/**
* @return The Virtual Machine Scale Set priority. If not specified, the default is 'Regular'.
*
*/
public Output> scaleSetPriority() {
return Codegen.optional(this.scaleSetPriority);
}
/**
* Possible values are any decimal value greater than zero or -1 which indicates the willingness to pay any on-demand price. For more details on spot pricing, see [spot VMs pricing](https://docs.microsoft.com/azure/virtual-machines/spot-vms#pricing)
*
*/
@Export(name="spotMaxPrice", refs={Double.class}, tree="[0]")
private Output* @Nullable */ Double> spotMaxPrice;
/**
* @return Possible values are any decimal value greater than zero or -1 which indicates the willingness to pay any on-demand price. For more details on spot pricing, see [spot VMs pricing](https://docs.microsoft.com/azure/virtual-machines/spot-vms#pricing)
*
*/
public Output> spotMaxPrice() {
return Codegen.optional(this.spotMaxPrice);
}
/**
* The tags to be persisted on the agent pool virtual machine scale set.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return The tags to be persisted on the agent pool virtual machine scale set.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Resource type
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type
*
*/
public Output type() {
return this.type;
}
/**
* Settings for upgrading the agentpool
*
*/
@Export(name="upgradeSettings", refs={AgentPoolUpgradeSettingsResponse.class}, tree="[0]")
private Output* @Nullable */ AgentPoolUpgradeSettingsResponse> upgradeSettings;
/**
* @return Settings for upgrading the agentpool
*
*/
public Output> upgradeSettings() {
return Codegen.optional(this.upgradeSettings);
}
/**
* VM size availability varies by region. If a node contains insufficient compute resources (memory, cpu, etc) pods might fail to run correctly. For more details on restricted VM sizes, see: https://docs.microsoft.com/azure/aks/quotas-skus-regions
*
*/
@Export(name="vmSize", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> vmSize;
/**
* @return VM size availability varies by region. If a node contains insufficient compute resources (memory, cpu, etc) pods might fail to run correctly. For more details on restricted VM sizes, see: https://docs.microsoft.com/azure/aks/quotas-skus-regions
*
*/
public Output> vmSize() {
return Codegen.optional(this.vmSize);
}
/**
* If this is not specified, a VNET and subnet will be generated and used. If no podSubnetID is specified, this applies to nodes and pods, otherwise it applies to just nodes. This is of the form: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/virtualNetworks/{virtualNetworkName}/subnets/{subnetName}
*
*/
@Export(name="vnetSubnetID", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> vnetSubnetID;
/**
* @return If this is not specified, a VNET and subnet will be generated and used. If no podSubnetID is specified, this applies to nodes and pods, otherwise it applies to just nodes. This is of the form: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/virtualNetworks/{virtualNetworkName}/subnets/{subnetName}
*
*/
public Output> vnetSubnetID() {
return Codegen.optional(this.vnetSubnetID);
}
/**
* Determines the type of workload a node can run.
*
*/
@Export(name="workloadRuntime", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> workloadRuntime;
/**
* @return Determines the type of workload a node can run.
*
*/
public Output> workloadRuntime() {
return Codegen.optional(this.workloadRuntime);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public AgentPool(java.lang.String name) {
this(name, AgentPoolArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public AgentPool(java.lang.String name, AgentPoolArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public AgentPool(java.lang.String name, AgentPoolArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:containerservice:AgentPool", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private AgentPool(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:containerservice:AgentPool", name, null, makeResourceOptions(options, id), false);
}
private static AgentPoolArgs makeArgs(AgentPoolArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? AgentPoolArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:containerservice/v20190201:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20190401:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20190601:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20190801:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20191001:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20191101:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20200101:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20200201:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20200301:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20200401:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20200601:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20200701:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20200901:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20201101:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20201201:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20210201:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20210301:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20210501:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20210701:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20210801:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20210901:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20211001:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20211101preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20220101:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20220102preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20220201:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20220202preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20220301:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20220302preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20220401:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20220402preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20220502preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20220601:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20220602preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20220701:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20220702preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20220802preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20220803preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20220901:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20220902preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20221002preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20221101:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20221102preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230101:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230102preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230201:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230202preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230301:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230302preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230401:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230402preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230501:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230502preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230601:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230602preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230701:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230702preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230801:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230802preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230901:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20230902preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20231001:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20231002preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20231101:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20231102preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20240101:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20240102preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20240201:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20240202preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20240302preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20240402preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20240501:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20240502preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20240602preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20240701:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20240702preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20240801:AgentPool").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static AgentPool get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new AgentPool(name, id, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy