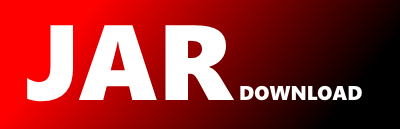
com.pulumi.azurenative.containerservice.LoadBalancer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.containerservice;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.containerservice.LoadBalancerArgs;
import com.pulumi.azurenative.containerservice.outputs.LabelSelectorResponse;
import com.pulumi.azurenative.containerservice.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The configurations regarding multiple standard load balancers. If not supplied, single load balancer mode will be used. Multiple standard load balancers mode will be used if at lease one configuration is supplied. There has to be a configuration named `kubernetes`.
* Azure REST API version: 2024-03-02-preview.
*
* Other available API versions: 2024-04-02-preview, 2024-05-02-preview, 2024-06-02-preview, 2024-07-02-preview.
*
* ## Example Usage
* ### Create or update a Load Balancer
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerservice.LoadBalancer;
* import com.pulumi.azurenative.containerservice.LoadBalancerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var loadBalancer = new LoadBalancer("loadBalancer", LoadBalancerArgs.builder()
* .allowServicePlacement(true)
* .loadBalancerName("kubernetes")
* .name("kubernetes")
* .primaryAgentPoolName("agentpool1")
* .resourceGroupName("rg1")
* .resourceName("clustername1")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:containerservice:LoadBalancer kubernetes /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ContainerService/managedClusters/{resourceName}/loadBalancers/{loadBalancerName}
* ```
*
*/
@ResourceType(type="azure-native:containerservice:LoadBalancer")
public class LoadBalancer extends com.pulumi.resources.CustomResource {
/**
* Whether to automatically place services on the load balancer. If not supplied, the default value is true. If set to false manually, both of the external and the internal load balancer will not be selected for services unless they explicitly target it.
*
*/
@Export(name="allowServicePlacement", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> allowServicePlacement;
/**
* @return Whether to automatically place services on the load balancer. If not supplied, the default value is true. If set to false manually, both of the external and the internal load balancer will not be selected for services unless they explicitly target it.
*
*/
public Output> allowServicePlacement() {
return Codegen.optional(this.allowServicePlacement);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* Nodes that match this selector will be possible members of this load balancer.
*
*/
@Export(name="nodeSelector", refs={LabelSelectorResponse.class}, tree="[0]")
private Output* @Nullable */ LabelSelectorResponse> nodeSelector;
/**
* @return Nodes that match this selector will be possible members of this load balancer.
*
*/
public Output> nodeSelector() {
return Codegen.optional(this.nodeSelector);
}
/**
* Required field. A string value that must specify the ID of an existing agent pool. All nodes in the given pool will always be added to this load balancer. This agent pool must have at least one node and minCount>=1 for autoscaling operations. An agent pool can only be the primary pool for a single load balancer.
*
*/
@Export(name="primaryAgentPoolName", refs={String.class}, tree="[0]")
private Output primaryAgentPoolName;
/**
* @return Required field. A string value that must specify the ID of an existing agent pool. All nodes in the given pool will always be added to this load balancer. This agent pool must have at least one node and minCount>=1 for autoscaling operations. An agent pool can only be the primary pool for a single load balancer.
*
*/
public Output primaryAgentPoolName() {
return this.primaryAgentPoolName;
}
/**
* The current provisioning state.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The current provisioning state.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* Only services that must match this selector can be placed on this load balancer.
*
*/
@Export(name="serviceLabelSelector", refs={LabelSelectorResponse.class}, tree="[0]")
private Output* @Nullable */ LabelSelectorResponse> serviceLabelSelector;
/**
* @return Only services that must match this selector can be placed on this load balancer.
*
*/
public Output> serviceLabelSelector() {
return Codegen.optional(this.serviceLabelSelector);
}
/**
* Services created in namespaces that match the selector can be placed on this load balancer.
*
*/
@Export(name="serviceNamespaceSelector", refs={LabelSelectorResponse.class}, tree="[0]")
private Output* @Nullable */ LabelSelectorResponse> serviceNamespaceSelector;
/**
* @return Services created in namespaces that match the selector can be placed on this load balancer.
*
*/
public Output> serviceNamespaceSelector() {
return Codegen.optional(this.serviceNamespaceSelector);
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public LoadBalancer(java.lang.String name) {
this(name, LoadBalancerArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public LoadBalancer(java.lang.String name, LoadBalancerArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public LoadBalancer(java.lang.String name, LoadBalancerArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:containerservice:LoadBalancer", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private LoadBalancer(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:containerservice:LoadBalancer", name, null, makeResourceOptions(options, id), false);
}
private static LoadBalancerArgs makeArgs(LoadBalancerArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? LoadBalancerArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:containerservice/v20240302preview:LoadBalancer").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20240402preview:LoadBalancer").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20240502preview:LoadBalancer").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20240602preview:LoadBalancer").build()),
Output.of(Alias.builder().type("azure-native:containerservice/v20240702preview:LoadBalancer").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static LoadBalancer get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new LoadBalancer(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy