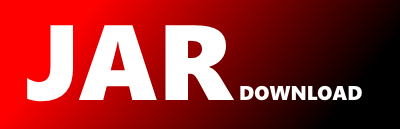
com.pulumi.azurenative.containerservice.LoadBalancerArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.containerservice;
import com.pulumi.azurenative.containerservice.inputs.LabelSelectorArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class LoadBalancerArgs extends com.pulumi.resources.ResourceArgs {
public static final LoadBalancerArgs Empty = new LoadBalancerArgs();
/**
* Whether to automatically place services on the load balancer. If not supplied, the default value is true. If set to false manually, both of the external and the internal load balancer will not be selected for services unless they explicitly target it.
*
*/
@Import(name="allowServicePlacement")
private @Nullable Output allowServicePlacement;
/**
* @return Whether to automatically place services on the load balancer. If not supplied, the default value is true. If set to false manually, both of the external and the internal load balancer will not be selected for services unless they explicitly target it.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy