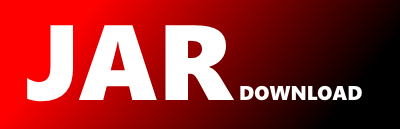
com.pulumi.azurenative.costmanagement.ViewByScope Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.costmanagement;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.costmanagement.ViewByScopeArgs;
import com.pulumi.azurenative.costmanagement.outputs.KpiPropertiesResponse;
import com.pulumi.azurenative.costmanagement.outputs.PivotPropertiesResponse;
import com.pulumi.azurenative.costmanagement.outputs.ReportConfigDatasetResponse;
import com.pulumi.azurenative.costmanagement.outputs.ReportConfigTimePeriodResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* States and configurations of Cost Analysis.
* Azure REST API version: 2023-03-01. Prior API version in Azure Native 1.x: 2019-11-01.
*
* Other available API versions: 2019-11-01, 2020-06-01, 2022-10-01, 2022-10-05-preview, 2023-04-01-preview, 2023-07-01-preview, 2023-08-01, 2023-09-01, 2023-11-01.
*
* ## Example Usage
* ### ResourceGroupCreateOrUpdateView
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.costmanagement.ViewByScope;
* import com.pulumi.azurenative.costmanagement.ViewByScopeArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ReportConfigDatasetArgs;
* import com.pulumi.azurenative.costmanagement.inputs.KpiPropertiesArgs;
* import com.pulumi.azurenative.costmanagement.inputs.PivotPropertiesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var viewByScope = new ViewByScope("viewByScope", ViewByScopeArgs.builder()
* .accumulated("true")
* .chart("Table")
* .dataSet(ReportConfigDatasetArgs.builder()
* .aggregation(Map.of("totalCost", Map.ofEntries(
* Map.entry("function", "Sum"),
* Map.entry("name", "PreTaxCost")
* )))
* .granularity("Daily")
* .grouping()
* .sorting(ReportConfigSortingArgs.builder()
* .direction("Ascending")
* .name("UsageDate")
* .build())
* .build())
* .displayName("swagger Example")
* .eTag("\"1d4ff9fe66f1d10\"")
* .kpis(
* KpiPropertiesArgs.builder()
* .enabled(true)
* .type("Forecast")
* .build(),
* KpiPropertiesArgs.builder()
* .enabled(true)
* .id("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Consumption/budgets/swaggerDemo")
* .type("Budget")
* .build())
* .metric("ActualCost")
* .pivots(
* PivotPropertiesArgs.builder()
* .name("ServiceName")
* .type("Dimension")
* .build(),
* PivotPropertiesArgs.builder()
* .name("MeterCategory")
* .type("Dimension")
* .build(),
* PivotPropertiesArgs.builder()
* .name("swaggerTagKey")
* .type("TagKey")
* .build())
* .scope("subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG")
* .timeframe("MonthToDate")
* .type("Usage")
* .viewName("swaggerExample")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:costmanagement:ViewByScope swaggerExample /{scope}/providers/Microsoft.CostManagement/views/{viewName}
* ```
*
*/
@ResourceType(type="azure-native:costmanagement:ViewByScope")
public class ViewByScope extends com.pulumi.resources.CustomResource {
/**
* Show costs accumulated over time.
*
*/
@Export(name="accumulated", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> accumulated;
/**
* @return Show costs accumulated over time.
*
*/
public Output> accumulated() {
return Codegen.optional(this.accumulated);
}
/**
* Chart type of the main view in Cost Analysis. Required.
*
*/
@Export(name="chart", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> chart;
/**
* @return Chart type of the main view in Cost Analysis. Required.
*
*/
public Output> chart() {
return Codegen.optional(this.chart);
}
/**
* Date the user created this view.
*
*/
@Export(name="createdOn", refs={String.class}, tree="[0]")
private Output createdOn;
/**
* @return Date the user created this view.
*
*/
public Output createdOn() {
return this.createdOn;
}
/**
* Currency of the current view.
*
*/
@Export(name="currency", refs={String.class}, tree="[0]")
private Output currency;
/**
* @return Currency of the current view.
*
*/
public Output currency() {
return this.currency;
}
/**
* Has definition for data in this report config.
*
*/
@Export(name="dataSet", refs={ReportConfigDatasetResponse.class}, tree="[0]")
private Output* @Nullable */ ReportConfigDatasetResponse> dataSet;
/**
* @return Has definition for data in this report config.
*
*/
public Output> dataSet() {
return Codegen.optional(this.dataSet);
}
/**
* Date range of the current view.
*
*/
@Export(name="dateRange", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> dateRange;
/**
* @return Date range of the current view.
*
*/
public Output> dateRange() {
return Codegen.optional(this.dateRange);
}
/**
* User input name of the view. Required.
*
*/
@Export(name="displayName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> displayName;
/**
* @return User input name of the view. Required.
*
*/
public Output> displayName() {
return Codegen.optional(this.displayName);
}
/**
* eTag of the resource. To handle concurrent update scenario, this field will be used to determine whether the user is updating the latest version or not.
*
*/
@Export(name="eTag", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> eTag;
/**
* @return eTag of the resource. To handle concurrent update scenario, this field will be used to determine whether the user is updating the latest version or not.
*
*/
public Output> eTag() {
return Codegen.optional(this.eTag);
}
/**
* If true, report includes monetary commitment.
*
*/
@Export(name="includeMonetaryCommitment", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> includeMonetaryCommitment;
/**
* @return If true, report includes monetary commitment.
*
*/
public Output> includeMonetaryCommitment() {
return Codegen.optional(this.includeMonetaryCommitment);
}
/**
* List of KPIs to show in Cost Analysis UI.
*
*/
@Export(name="kpis", refs={List.class,KpiPropertiesResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> kpis;
/**
* @return List of KPIs to show in Cost Analysis UI.
*
*/
public Output>> kpis() {
return Codegen.optional(this.kpis);
}
/**
* Metric to use when displaying costs.
*
*/
@Export(name="metric", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> metric;
/**
* @return Metric to use when displaying costs.
*
*/
public Output> metric() {
return Codegen.optional(this.metric);
}
/**
* Date when the user last modified this view.
*
*/
@Export(name="modifiedOn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> modifiedOn;
/**
* @return Date when the user last modified this view.
*
*/
public Output> modifiedOn() {
return Codegen.optional(this.modifiedOn);
}
/**
* Resource name.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource name.
*
*/
public Output name() {
return this.name;
}
/**
* Configuration of 3 sub-views in the Cost Analysis UI.
*
*/
@Export(name="pivots", refs={List.class,PivotPropertiesResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> pivots;
/**
* @return Configuration of 3 sub-views in the Cost Analysis UI.
*
*/
public Output>> pivots() {
return Codegen.optional(this.pivots);
}
/**
* Cost Management scope to save the view on. This includes 'subscriptions/{subscriptionId}' for subscription scope, 'subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for Department scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}' for EnrollmentAccount scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for BillingProfile scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/invoiceSections/{invoiceSectionId}' for InvoiceSection scope, 'providers/Microsoft.Management/managementGroups/{managementGroupId}' for Management Group scope, '/providers/Microsoft.CostManagement/externalBillingAccounts/{externalBillingAccountName}' for ExternalBillingAccount scope, and '/providers/Microsoft.CostManagement/externalSubscriptions/{externalSubscriptionName}' for ExternalSubscription scope.
*
*/
@Export(name="scope", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> scope;
/**
* @return Cost Management scope to save the view on. This includes 'subscriptions/{subscriptionId}' for subscription scope, 'subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for Department scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}' for EnrollmentAccount scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for BillingProfile scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/invoiceSections/{invoiceSectionId}' for InvoiceSection scope, 'providers/Microsoft.Management/managementGroups/{managementGroupId}' for Management Group scope, '/providers/Microsoft.CostManagement/externalBillingAccounts/{externalBillingAccountName}' for ExternalBillingAccount scope, and '/providers/Microsoft.CostManagement/externalSubscriptions/{externalSubscriptionName}' for ExternalSubscription scope.
*
*/
public Output> scope() {
return Codegen.optional(this.scope);
}
/**
* Has time period for pulling data for the report.
*
*/
@Export(name="timePeriod", refs={ReportConfigTimePeriodResponse.class}, tree="[0]")
private Output* @Nullable */ ReportConfigTimePeriodResponse> timePeriod;
/**
* @return Has time period for pulling data for the report.
*
*/
public Output> timePeriod() {
return Codegen.optional(this.timePeriod);
}
/**
* The time frame for pulling data for the report. If custom, then a specific time period must be provided.
*
*/
@Export(name="timeframe", refs={String.class}, tree="[0]")
private Output timeframe;
/**
* @return The time frame for pulling data for the report. If custom, then a specific time period must be provided.
*
*/
public Output timeframe() {
return this.timeframe;
}
/**
* Resource type.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type.
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ViewByScope(java.lang.String name) {
this(name, ViewByScopeArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ViewByScope(java.lang.String name, ViewByScopeArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ViewByScope(java.lang.String name, ViewByScopeArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:costmanagement:ViewByScope", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private ViewByScope(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:costmanagement:ViewByScope", name, null, makeResourceOptions(options, id), false);
}
private static ViewByScopeArgs makeArgs(ViewByScopeArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ViewByScopeArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:costmanagement/v20190401preview:ViewByScope").build()),
Output.of(Alias.builder().type("azure-native:costmanagement/v20191101:ViewByScope").build()),
Output.of(Alias.builder().type("azure-native:costmanagement/v20200601:ViewByScope").build()),
Output.of(Alias.builder().type("azure-native:costmanagement/v20211001:ViewByScope").build()),
Output.of(Alias.builder().type("azure-native:costmanagement/v20220801preview:ViewByScope").build()),
Output.of(Alias.builder().type("azure-native:costmanagement/v20221001:ViewByScope").build()),
Output.of(Alias.builder().type("azure-native:costmanagement/v20221001preview:ViewByScope").build()),
Output.of(Alias.builder().type("azure-native:costmanagement/v20221005preview:ViewByScope").build()),
Output.of(Alias.builder().type("azure-native:costmanagement/v20230301:ViewByScope").build()),
Output.of(Alias.builder().type("azure-native:costmanagement/v20230401preview:ViewByScope").build()),
Output.of(Alias.builder().type("azure-native:costmanagement/v20230701preview:ViewByScope").build()),
Output.of(Alias.builder().type("azure-native:costmanagement/v20230801:ViewByScope").build()),
Output.of(Alias.builder().type("azure-native:costmanagement/v20230901:ViewByScope").build()),
Output.of(Alias.builder().type("azure-native:costmanagement/v20231101:ViewByScope").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ViewByScope get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ViewByScope(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy