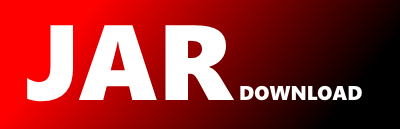
com.pulumi.azurenative.costmanagement.inputs.ReportDatasetArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.costmanagement.inputs;
import com.pulumi.azurenative.costmanagement.enums.GranularityType;
import com.pulumi.azurenative.costmanagement.inputs.ReportAggregationArgs;
import com.pulumi.azurenative.costmanagement.inputs.ReportDatasetConfigurationArgs;
import com.pulumi.azurenative.costmanagement.inputs.ReportFilterArgs;
import com.pulumi.azurenative.costmanagement.inputs.ReportGroupingArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The definition of data present in the report.
*
*/
public final class ReportDatasetArgs extends com.pulumi.resources.ResourceArgs {
public static final ReportDatasetArgs Empty = new ReportDatasetArgs();
/**
* Dictionary of aggregation expression to use in the report. The key of each item in the dictionary is the alias for the aggregated column. Report can have up to 2 aggregation clauses.
*
*/
@Import(name="aggregation")
private @Nullable Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy