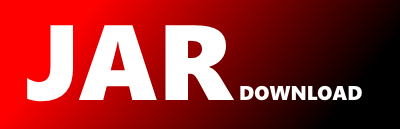
com.pulumi.azurenative.customerinsights.KpiArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.customerinsights;
import com.pulumi.azurenative.customerinsights.enums.CalculationWindowTypes;
import com.pulumi.azurenative.customerinsights.enums.EntityTypes;
import com.pulumi.azurenative.customerinsights.enums.KpiFunctions;
import com.pulumi.azurenative.customerinsights.inputs.KpiAliasArgs;
import com.pulumi.azurenative.customerinsights.inputs.KpiExtractArgs;
import com.pulumi.azurenative.customerinsights.inputs.KpiThresholdsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class KpiArgs extends com.pulumi.resources.ResourceArgs {
public static final KpiArgs Empty = new KpiArgs();
/**
* The aliases.
*
*/
@Import(name="aliases")
private @Nullable Output> aliases;
/**
* @return The aliases.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy