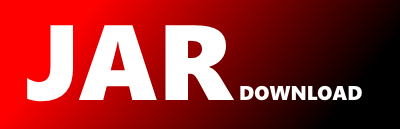
com.pulumi.azurenative.customerinsights.Link Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.customerinsights;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.customerinsights.LinkArgs;
import com.pulumi.azurenative.customerinsights.outputs.ParticipantPropertyReferenceResponse;
import com.pulumi.azurenative.customerinsights.outputs.TypePropertiesMappingResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The link resource format.
* Azure REST API version: 2017-04-26. Prior API version in Azure Native 1.x: 2017-04-26.
*
* Other available API versions: 2017-01-01.
*
* ## Example Usage
* ### Links_CreateOrUpdate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.customerinsights.Link;
* import com.pulumi.azurenative.customerinsights.LinkArgs;
* import com.pulumi.azurenative.customerinsights.inputs.TypePropertiesMappingArgs;
* import com.pulumi.azurenative.customerinsights.inputs.ParticipantPropertyReferenceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var link = new Link("link", LinkArgs.builder()
* .description(Map.of("en-us", "Link Description"))
* .displayName(Map.of("en-us", "Link DisplayName"))
* .hubName("sdkTestHub")
* .linkName("linkTest4806")
* .mappings(TypePropertiesMappingArgs.builder()
* .linkType("UpdateAlways")
* .sourcePropertyName("testInteraction1949")
* .targetPropertyName("testProfile1446")
* .build())
* .participantPropertyReferences(ParticipantPropertyReferenceArgs.builder()
* .sourcePropertyName("testInteraction1949")
* .targetPropertyName("ProfileId")
* .build())
* .resourceGroupName("TestHubRG")
* .sourceEntityType("Interaction")
* .sourceEntityTypeName("testInteraction1949")
* .targetEntityType("Profile")
* .targetEntityTypeName("testProfile1446")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:customerinsights:Link azSdkTestHub/linkTest4806 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.CustomerInsights/hubs/{hubName}/links/{linkName}
* ```
*
*/
@ResourceType(type="azure-native:customerinsights:Link")
public class Link extends com.pulumi.resources.CustomResource {
/**
* Localized descriptions for the Link.
*
*/
@Export(name="description", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> description;
/**
* @return Localized descriptions for the Link.
*
*/
public Output>> description() {
return Codegen.optional(this.description);
}
/**
* Localized display name for the Link.
*
*/
@Export(name="displayName", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> displayName;
/**
* @return Localized display name for the Link.
*
*/
public Output>> displayName() {
return Codegen.optional(this.displayName);
}
/**
* The link name.
*
*/
@Export(name="linkName", refs={String.class}, tree="[0]")
private Output linkName;
/**
* @return The link name.
*
*/
public Output linkName() {
return this.linkName;
}
/**
* The set of properties mappings between the source and target Types.
*
*/
@Export(name="mappings", refs={List.class,TypePropertiesMappingResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> mappings;
/**
* @return The set of properties mappings between the source and target Types.
*
*/
public Output>> mappings() {
return Codegen.optional(this.mappings);
}
/**
* Resource name.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource name.
*
*/
public Output name() {
return this.name;
}
/**
* Determines whether this link is supposed to create or delete instances if Link is NOT Reference Only.
*
*/
@Export(name="operationType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> operationType;
/**
* @return Determines whether this link is supposed to create or delete instances if Link is NOT Reference Only.
*
*/
public Output> operationType() {
return Codegen.optional(this.operationType);
}
/**
* The properties that represent the participating profile.
*
*/
@Export(name="participantPropertyReferences", refs={List.class,ParticipantPropertyReferenceResponse.class}, tree="[0,1]")
private Output> participantPropertyReferences;
/**
* @return The properties that represent the participating profile.
*
*/
public Output> participantPropertyReferences() {
return this.participantPropertyReferences;
}
/**
* Provisioning state.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Provisioning state.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* Indicating whether the link is reference only link. This flag is ignored if the Mappings are defined. If the mappings are not defined and it is set to true, links processing will not create or update profiles.
*
*/
@Export(name="referenceOnly", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> referenceOnly;
/**
* @return Indicating whether the link is reference only link. This flag is ignored if the Mappings are defined. If the mappings are not defined and it is set to true, links processing will not create or update profiles.
*
*/
public Output> referenceOnly() {
return Codegen.optional(this.referenceOnly);
}
/**
* Type of source entity.
*
*/
@Export(name="sourceEntityType", refs={String.class}, tree="[0]")
private Output sourceEntityType;
/**
* @return Type of source entity.
*
*/
public Output sourceEntityType() {
return this.sourceEntityType;
}
/**
* Name of the source Entity Type.
*
*/
@Export(name="sourceEntityTypeName", refs={String.class}, tree="[0]")
private Output sourceEntityTypeName;
/**
* @return Name of the source Entity Type.
*
*/
public Output sourceEntityTypeName() {
return this.sourceEntityTypeName;
}
/**
* Type of target entity.
*
*/
@Export(name="targetEntityType", refs={String.class}, tree="[0]")
private Output targetEntityType;
/**
* @return Type of target entity.
*
*/
public Output targetEntityType() {
return this.targetEntityType;
}
/**
* Name of the target Entity Type.
*
*/
@Export(name="targetEntityTypeName", refs={String.class}, tree="[0]")
private Output targetEntityTypeName;
/**
* @return Name of the target Entity Type.
*
*/
public Output targetEntityTypeName() {
return this.targetEntityTypeName;
}
/**
* The hub name.
*
*/
@Export(name="tenantId", refs={String.class}, tree="[0]")
private Output tenantId;
/**
* @return The hub name.
*
*/
public Output tenantId() {
return this.tenantId;
}
/**
* Resource type.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type.
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Link(java.lang.String name) {
this(name, LinkArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Link(java.lang.String name, LinkArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Link(java.lang.String name, LinkArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:customerinsights:Link", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Link(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:customerinsights:Link", name, null, makeResourceOptions(options, id), false);
}
private static LinkArgs makeArgs(LinkArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? LinkArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:customerinsights/v20170101:Link").build()),
Output.of(Alias.builder().type("azure-native:customerinsights/v20170426:Link").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Link get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Link(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy