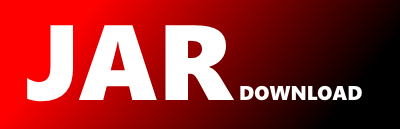
com.pulumi.azurenative.databox.inputs.DataBoxCustomerDiskJobDetailsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.databox.inputs;
import com.pulumi.azurenative.databox.inputs.ContactDetailsArgs;
import com.pulumi.azurenative.databox.inputs.DataExportDetailsArgs;
import com.pulumi.azurenative.databox.inputs.DataImportDetailsArgs;
import com.pulumi.azurenative.databox.inputs.ImportDiskDetailsArgs;
import com.pulumi.azurenative.databox.inputs.KeyEncryptionKeyArgs;
import com.pulumi.azurenative.databox.inputs.PackageCarrierDetailsArgs;
import com.pulumi.azurenative.databox.inputs.PreferencesArgs;
import com.pulumi.azurenative.databox.inputs.ReverseShippingDetailsArgs;
import com.pulumi.azurenative.databox.inputs.ShippingAddressArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Customer disk job details.
*
*/
public final class DataBoxCustomerDiskJobDetailsArgs extends com.pulumi.resources.ResourceArgs {
public static final DataBoxCustomerDiskJobDetailsArgs Empty = new DataBoxCustomerDiskJobDetailsArgs();
/**
* Contact details for notification and shipping.
*
*/
@Import(name="contactDetails", required=true)
private Output contactDetails;
/**
* @return Contact details for notification and shipping.
*
*/
public Output contactDetails() {
return this.contactDetails;
}
/**
* Details of the data to be exported from azure.
*
*/
@Import(name="dataExportDetails")
private @Nullable Output> dataExportDetails;
/**
* @return Details of the data to be exported from azure.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy