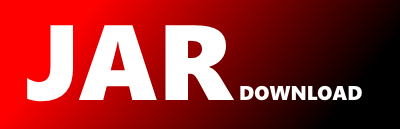
com.pulumi.azurenative.databox.inputs.ShippingAddressArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.databox.inputs;
import com.pulumi.azurenative.databox.enums.AddressType;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Shipping address where customer wishes to receive the device.
*
*/
public final class ShippingAddressArgs extends com.pulumi.resources.ResourceArgs {
public static final ShippingAddressArgs Empty = new ShippingAddressArgs();
/**
* Type of address.
*
*/
@Import(name="addressType")
private @Nullable Output> addressType;
/**
* @return Type of address.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy