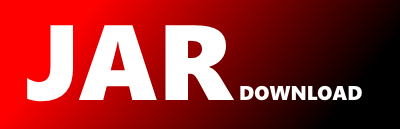
com.pulumi.azurenative.databricks.outputs.GetWorkspaceResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.databricks.outputs;
import com.pulumi.azurenative.databricks.outputs.CreatedByResponse;
import com.pulumi.azurenative.databricks.outputs.ManagedIdentityConfigurationResponse;
import com.pulumi.azurenative.databricks.outputs.PrivateEndpointConnectionResponse;
import com.pulumi.azurenative.databricks.outputs.SkuResponse;
import com.pulumi.azurenative.databricks.outputs.SystemDataResponse;
import com.pulumi.azurenative.databricks.outputs.WorkspaceCustomParametersResponse;
import com.pulumi.azurenative.databricks.outputs.WorkspacePropertiesResponseEncryption;
import com.pulumi.azurenative.databricks.outputs.WorkspaceProviderAuthorizationResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetWorkspaceResult {
/**
* @return The workspace provider authorizations.
*
*/
private @Nullable List authorizations;
/**
* @return Indicates the Object ID, PUID and Application ID of entity that created the workspace.
*
*/
private @Nullable CreatedByResponse createdBy;
/**
* @return Specifies the date and time when the workspace is created.
*
*/
private String createdDateTime;
/**
* @return The resource Id of the managed disk encryption set.
*
*/
private String diskEncryptionSetId;
/**
* @return Encryption properties for databricks workspace
*
*/
private @Nullable WorkspacePropertiesResponseEncryption encryption;
/**
* @return Fully qualified resource Id for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return The details of Managed Identity of Disk Encryption Set used for Managed Disk Encryption
*
*/
private @Nullable ManagedIdentityConfigurationResponse managedDiskIdentity;
/**
* @return The managed resource group Id.
*
*/
private String managedResourceGroupId;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The workspace's custom parameters.
*
*/
private @Nullable WorkspaceCustomParametersResponse parameters;
/**
* @return Private endpoint connections created on the workspace
*
*/
private List privateEndpointConnections;
/**
* @return The workspace provisioning state.
*
*/
private String provisioningState;
/**
* @return The network access type for accessing workspace. Set value to disabled to access workspace only via private link.
*
*/
private @Nullable String publicNetworkAccess;
/**
* @return Gets or sets a value indicating whether data plane (clusters) to control plane communication happen over private endpoint. Supported values are 'AllRules' and 'NoAzureDatabricksRules'. 'NoAzureServiceRules' value is for internal use only.
*
*/
private @Nullable String requiredNsgRules;
/**
* @return The SKU of the resource.
*
*/
private @Nullable SkuResponse sku;
/**
* @return The details of Managed Identity of Storage Account
*
*/
private @Nullable ManagedIdentityConfigurationResponse storageAccountIdentity;
/**
* @return The system metadata relating to this resource
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource. Ex- Microsoft.Compute/virtualMachines or Microsoft.Storage/storageAccounts.
*
*/
private String type;
/**
* @return The blob URI where the UI definition file is located.
*
*/
private @Nullable String uiDefinitionUri;
/**
* @return Indicates the Object ID, PUID and Application ID of entity that last updated the workspace.
*
*/
private @Nullable CreatedByResponse updatedBy;
/**
* @return The unique identifier of the databricks workspace in databricks control plane.
*
*/
private String workspaceId;
/**
* @return The workspace URL which is of the format 'adb-{workspaceId}.{random}.azuredatabricks.net'
*
*/
private String workspaceUrl;
private GetWorkspaceResult() {}
/**
* @return The workspace provider authorizations.
*
*/
public List authorizations() {
return this.authorizations == null ? List.of() : this.authorizations;
}
/**
* @return Indicates the Object ID, PUID and Application ID of entity that created the workspace.
*
*/
public Optional createdBy() {
return Optional.ofNullable(this.createdBy);
}
/**
* @return Specifies the date and time when the workspace is created.
*
*/
public String createdDateTime() {
return this.createdDateTime;
}
/**
* @return The resource Id of the managed disk encryption set.
*
*/
public String diskEncryptionSetId() {
return this.diskEncryptionSetId;
}
/**
* @return Encryption properties for databricks workspace
*
*/
public Optional encryption() {
return Optional.ofNullable(this.encryption);
}
/**
* @return Fully qualified resource Id for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return The details of Managed Identity of Disk Encryption Set used for Managed Disk Encryption
*
*/
public Optional managedDiskIdentity() {
return Optional.ofNullable(this.managedDiskIdentity);
}
/**
* @return The managed resource group Id.
*
*/
public String managedResourceGroupId() {
return this.managedResourceGroupId;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return The workspace's custom parameters.
*
*/
public Optional parameters() {
return Optional.ofNullable(this.parameters);
}
/**
* @return Private endpoint connections created on the workspace
*
*/
public List privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* @return The workspace provisioning state.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The network access type for accessing workspace. Set value to disabled to access workspace only via private link.
*
*/
public Optional publicNetworkAccess() {
return Optional.ofNullable(this.publicNetworkAccess);
}
/**
* @return Gets or sets a value indicating whether data plane (clusters) to control plane communication happen over private endpoint. Supported values are 'AllRules' and 'NoAzureDatabricksRules'. 'NoAzureServiceRules' value is for internal use only.
*
*/
public Optional requiredNsgRules() {
return Optional.ofNullable(this.requiredNsgRules);
}
/**
* @return The SKU of the resource.
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return The details of Managed Identity of Storage Account
*
*/
public Optional storageAccountIdentity() {
return Optional.ofNullable(this.storageAccountIdentity);
}
/**
* @return The system metadata relating to this resource
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource. Ex- Microsoft.Compute/virtualMachines or Microsoft.Storage/storageAccounts.
*
*/
public String type() {
return this.type;
}
/**
* @return The blob URI where the UI definition file is located.
*
*/
public Optional uiDefinitionUri() {
return Optional.ofNullable(this.uiDefinitionUri);
}
/**
* @return Indicates the Object ID, PUID and Application ID of entity that last updated the workspace.
*
*/
public Optional updatedBy() {
return Optional.ofNullable(this.updatedBy);
}
/**
* @return The unique identifier of the databricks workspace in databricks control plane.
*
*/
public String workspaceId() {
return this.workspaceId;
}
/**
* @return The workspace URL which is of the format 'adb-{workspaceId}.{random}.azuredatabricks.net'
*
*/
public String workspaceUrl() {
return this.workspaceUrl;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetWorkspaceResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List authorizations;
private @Nullable CreatedByResponse createdBy;
private String createdDateTime;
private String diskEncryptionSetId;
private @Nullable WorkspacePropertiesResponseEncryption encryption;
private String id;
private String location;
private @Nullable ManagedIdentityConfigurationResponse managedDiskIdentity;
private String managedResourceGroupId;
private String name;
private @Nullable WorkspaceCustomParametersResponse parameters;
private List privateEndpointConnections;
private String provisioningState;
private @Nullable String publicNetworkAccess;
private @Nullable String requiredNsgRules;
private @Nullable SkuResponse sku;
private @Nullable ManagedIdentityConfigurationResponse storageAccountIdentity;
private SystemDataResponse systemData;
private @Nullable Map tags;
private String type;
private @Nullable String uiDefinitionUri;
private @Nullable CreatedByResponse updatedBy;
private String workspaceId;
private String workspaceUrl;
public Builder() {}
public Builder(GetWorkspaceResult defaults) {
Objects.requireNonNull(defaults);
this.authorizations = defaults.authorizations;
this.createdBy = defaults.createdBy;
this.createdDateTime = defaults.createdDateTime;
this.diskEncryptionSetId = defaults.diskEncryptionSetId;
this.encryption = defaults.encryption;
this.id = defaults.id;
this.location = defaults.location;
this.managedDiskIdentity = defaults.managedDiskIdentity;
this.managedResourceGroupId = defaults.managedResourceGroupId;
this.name = defaults.name;
this.parameters = defaults.parameters;
this.privateEndpointConnections = defaults.privateEndpointConnections;
this.provisioningState = defaults.provisioningState;
this.publicNetworkAccess = defaults.publicNetworkAccess;
this.requiredNsgRules = defaults.requiredNsgRules;
this.sku = defaults.sku;
this.storageAccountIdentity = defaults.storageAccountIdentity;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.type = defaults.type;
this.uiDefinitionUri = defaults.uiDefinitionUri;
this.updatedBy = defaults.updatedBy;
this.workspaceId = defaults.workspaceId;
this.workspaceUrl = defaults.workspaceUrl;
}
@CustomType.Setter
public Builder authorizations(@Nullable List authorizations) {
this.authorizations = authorizations;
return this;
}
public Builder authorizations(WorkspaceProviderAuthorizationResponse... authorizations) {
return authorizations(List.of(authorizations));
}
@CustomType.Setter
public Builder createdBy(@Nullable CreatedByResponse createdBy) {
this.createdBy = createdBy;
return this;
}
@CustomType.Setter
public Builder createdDateTime(String createdDateTime) {
if (createdDateTime == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "createdDateTime");
}
this.createdDateTime = createdDateTime;
return this;
}
@CustomType.Setter
public Builder diskEncryptionSetId(String diskEncryptionSetId) {
if (diskEncryptionSetId == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "diskEncryptionSetId");
}
this.diskEncryptionSetId = diskEncryptionSetId;
return this;
}
@CustomType.Setter
public Builder encryption(@Nullable WorkspacePropertiesResponseEncryption encryption) {
this.encryption = encryption;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder managedDiskIdentity(@Nullable ManagedIdentityConfigurationResponse managedDiskIdentity) {
this.managedDiskIdentity = managedDiskIdentity;
return this;
}
@CustomType.Setter
public Builder managedResourceGroupId(String managedResourceGroupId) {
if (managedResourceGroupId == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "managedResourceGroupId");
}
this.managedResourceGroupId = managedResourceGroupId;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder parameters(@Nullable WorkspaceCustomParametersResponse parameters) {
this.parameters = parameters;
return this;
}
@CustomType.Setter
public Builder privateEndpointConnections(List privateEndpointConnections) {
if (privateEndpointConnections == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "privateEndpointConnections");
}
this.privateEndpointConnections = privateEndpointConnections;
return this;
}
public Builder privateEndpointConnections(PrivateEndpointConnectionResponse... privateEndpointConnections) {
return privateEndpointConnections(List.of(privateEndpointConnections));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder publicNetworkAccess(@Nullable String publicNetworkAccess) {
this.publicNetworkAccess = publicNetworkAccess;
return this;
}
@CustomType.Setter
public Builder requiredNsgRules(@Nullable String requiredNsgRules) {
this.requiredNsgRules = requiredNsgRules;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable SkuResponse sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder storageAccountIdentity(@Nullable ManagedIdentityConfigurationResponse storageAccountIdentity) {
this.storageAccountIdentity = storageAccountIdentity;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder uiDefinitionUri(@Nullable String uiDefinitionUri) {
this.uiDefinitionUri = uiDefinitionUri;
return this;
}
@CustomType.Setter
public Builder updatedBy(@Nullable CreatedByResponse updatedBy) {
this.updatedBy = updatedBy;
return this;
}
@CustomType.Setter
public Builder workspaceId(String workspaceId) {
if (workspaceId == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "workspaceId");
}
this.workspaceId = workspaceId;
return this;
}
@CustomType.Setter
public Builder workspaceUrl(String workspaceUrl) {
if (workspaceUrl == null) {
throw new MissingRequiredPropertyException("GetWorkspaceResult", "workspaceUrl");
}
this.workspaceUrl = workspaceUrl;
return this;
}
public GetWorkspaceResult build() {
final var _resultValue = new GetWorkspaceResult();
_resultValue.authorizations = authorizations;
_resultValue.createdBy = createdBy;
_resultValue.createdDateTime = createdDateTime;
_resultValue.diskEncryptionSetId = diskEncryptionSetId;
_resultValue.encryption = encryption;
_resultValue.id = id;
_resultValue.location = location;
_resultValue.managedDiskIdentity = managedDiskIdentity;
_resultValue.managedResourceGroupId = managedResourceGroupId;
_resultValue.name = name;
_resultValue.parameters = parameters;
_resultValue.privateEndpointConnections = privateEndpointConnections;
_resultValue.provisioningState = provisioningState;
_resultValue.publicNetworkAccess = publicNetworkAccess;
_resultValue.requiredNsgRules = requiredNsgRules;
_resultValue.sku = sku;
_resultValue.storageAccountIdentity = storageAccountIdentity;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.uiDefinitionUri = uiDefinitionUri;
_resultValue.updatedBy = updatedBy;
_resultValue.workspaceId = workspaceId;
_resultValue.workspaceUrl = workspaceUrl;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy