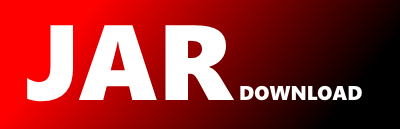
com.pulumi.azurenative.datafactory.inputs.ChainingTriggerArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.inputs;
import com.pulumi.azurenative.datafactory.inputs.PipelineReferenceArgs;
import com.pulumi.azurenative.datafactory.inputs.TriggerPipelineReferenceArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Trigger that allows the referenced pipeline to depend on other pipeline runs based on runDimension Name/Value pairs. Upstream pipelines should declare the same runDimension Name and their runs should have the values for those runDimensions. The referenced pipeline run would be triggered if the values for the runDimension match for all upstream pipeline runs.
*
*/
public final class ChainingTriggerArgs extends com.pulumi.resources.ResourceArgs {
public static final ChainingTriggerArgs Empty = new ChainingTriggerArgs();
/**
* List of tags that can be used for describing the trigger.
*
*/
@Import(name="annotations")
private @Nullable Output> annotations;
/**
* @return List of tags that can be used for describing the trigger.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy