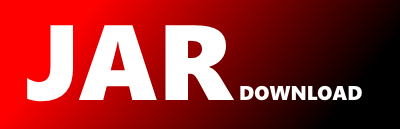
com.pulumi.azurenative.datafactory.inputs.HDInsightOnDemandLinkedServiceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.inputs;
import com.pulumi.azurenative.datafactory.inputs.AzureKeyVaultSecretReferenceArgs;
import com.pulumi.azurenative.datafactory.inputs.CredentialReferenceArgs;
import com.pulumi.azurenative.datafactory.inputs.IntegrationRuntimeReferenceArgs;
import com.pulumi.azurenative.datafactory.inputs.LinkedServiceReferenceArgs;
import com.pulumi.azurenative.datafactory.inputs.ParameterSpecificationArgs;
import com.pulumi.azurenative.datafactory.inputs.ScriptActionArgs;
import com.pulumi.azurenative.datafactory.inputs.SecureStringArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* HDInsight ondemand linked service.
*
*/
public final class HDInsightOnDemandLinkedServiceArgs extends com.pulumi.resources.ResourceArgs {
public static final HDInsightOnDemandLinkedServiceArgs Empty = new HDInsightOnDemandLinkedServiceArgs();
/**
* Specifies additional storage accounts for the HDInsight linked service so that the Data Factory service can register them on your behalf.
*
*/
@Import(name="additionalLinkedServiceNames")
private @Nullable Output> additionalLinkedServiceNames;
/**
* @return Specifies additional storage accounts for the HDInsight linked service so that the Data Factory service can register them on your behalf.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy