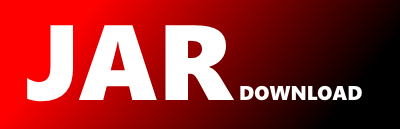
com.pulumi.azurenative.datafactory.inputs.PostgreSqlV2LinkedServiceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.inputs;
import com.pulumi.azurenative.datafactory.inputs.AzureKeyVaultSecretReferenceArgs;
import com.pulumi.azurenative.datafactory.inputs.IntegrationRuntimeReferenceArgs;
import com.pulumi.azurenative.datafactory.inputs.ParameterSpecificationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Linked service for PostgreSQLV2 data source.
*
*/
public final class PostgreSqlV2LinkedServiceArgs extends com.pulumi.resources.ResourceArgs {
public static final PostgreSqlV2LinkedServiceArgs Empty = new PostgreSqlV2LinkedServiceArgs();
/**
* List of tags that can be used for describing the linked service.
*
*/
@Import(name="annotations")
private @Nullable Output> annotations;
/**
* @return List of tags that can be used for describing the linked service.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy