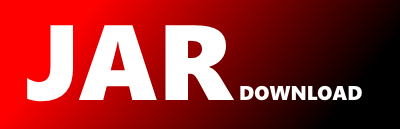
com.pulumi.azurenative.datafactory.outputs.AmazonS3CompatibleReadSettingsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AmazonS3CompatibleReadSettingsResponse {
/**
* @return Indicates whether the source files need to be deleted after copy completion. Default is false. Type: boolean (or Expression with resultType boolean).
*
*/
private @Nullable Object deleteFilesAfterCompletion;
/**
* @return If true, disable data store metrics collection. Default is false. Type: boolean (or Expression with resultType boolean).
*
*/
private @Nullable Object disableMetricsCollection;
/**
* @return Indicates whether to enable partition discovery. Type: boolean (or Expression with resultType boolean).
*
*/
private @Nullable Object enablePartitionDiscovery;
/**
* @return Point to a text file that lists each file (relative path to the path configured in the dataset) that you want to copy. Type: string (or Expression with resultType string).
*
*/
private @Nullable Object fileListPath;
/**
* @return The maximum concurrent connection count for the source data store. Type: integer (or Expression with resultType integer).
*
*/
private @Nullable Object maxConcurrentConnections;
/**
* @return The end of file's modified datetime. Type: string (or Expression with resultType string).
*
*/
private @Nullable Object modifiedDatetimeEnd;
/**
* @return The start of file's modified datetime. Type: string (or Expression with resultType string).
*
*/
private @Nullable Object modifiedDatetimeStart;
/**
* @return Specify the root path where partition discovery starts from. Type: string (or Expression with resultType string).
*
*/
private @Nullable Object partitionRootPath;
/**
* @return The prefix filter for the S3 Compatible object name. Type: string (or Expression with resultType string).
*
*/
private @Nullable Object prefix;
/**
* @return If true, files under the folder path will be read recursively. Default is true. Type: boolean (or Expression with resultType boolean).
*
*/
private @Nullable Object recursive;
/**
* @return The read setting type.
* Expected value is 'AmazonS3CompatibleReadSettings'.
*
*/
private String type;
/**
* @return Amazon S3 Compatible wildcardFileName. Type: string (or Expression with resultType string).
*
*/
private @Nullable Object wildcardFileName;
/**
* @return Amazon S3 Compatible wildcardFolderPath. Type: string (or Expression with resultType string).
*
*/
private @Nullable Object wildcardFolderPath;
private AmazonS3CompatibleReadSettingsResponse() {}
/**
* @return Indicates whether the source files need to be deleted after copy completion. Default is false. Type: boolean (or Expression with resultType boolean).
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy