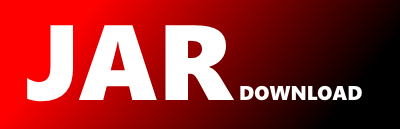
com.pulumi.azurenative.datafactory.outputs.ManagedIntegrationRuntimeStatusResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.outputs;
import com.pulumi.azurenative.datafactory.outputs.ManagedIntegrationRuntimeErrorResponse;
import com.pulumi.azurenative.datafactory.outputs.ManagedIntegrationRuntimeNodeResponse;
import com.pulumi.azurenative.datafactory.outputs.ManagedIntegrationRuntimeOperationResultResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class ManagedIntegrationRuntimeStatusResponse {
/**
* @return The time at which the integration runtime was created, in ISO8601 format.
*
*/
private String createTime;
/**
* @return The data factory name which the integration runtime belong to.
*
*/
private String dataFactoryName;
/**
* @return The last operation result that occurred on this integration runtime.
*
*/
private ManagedIntegrationRuntimeOperationResultResponse lastOperation;
/**
* @return The list of nodes for managed integration runtime.
*
*/
private List nodes;
/**
* @return The errors that occurred on this integration runtime.
*
*/
private List otherErrors;
/**
* @return The state of integration runtime.
*
*/
private String state;
/**
* @return The type of integration runtime.
* Expected value is 'Managed'.
*
*/
private String type;
private ManagedIntegrationRuntimeStatusResponse() {}
/**
* @return The time at which the integration runtime was created, in ISO8601 format.
*
*/
public String createTime() {
return this.createTime;
}
/**
* @return The data factory name which the integration runtime belong to.
*
*/
public String dataFactoryName() {
return this.dataFactoryName;
}
/**
* @return The last operation result that occurred on this integration runtime.
*
*/
public ManagedIntegrationRuntimeOperationResultResponse lastOperation() {
return this.lastOperation;
}
/**
* @return The list of nodes for managed integration runtime.
*
*/
public List nodes() {
return this.nodes;
}
/**
* @return The errors that occurred on this integration runtime.
*
*/
public List otherErrors() {
return this.otherErrors;
}
/**
* @return The state of integration runtime.
*
*/
public String state() {
return this.state;
}
/**
* @return The type of integration runtime.
* Expected value is 'Managed'.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ManagedIntegrationRuntimeStatusResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String createTime;
private String dataFactoryName;
private ManagedIntegrationRuntimeOperationResultResponse lastOperation;
private List nodes;
private List otherErrors;
private String state;
private String type;
public Builder() {}
public Builder(ManagedIntegrationRuntimeStatusResponse defaults) {
Objects.requireNonNull(defaults);
this.createTime = defaults.createTime;
this.dataFactoryName = defaults.dataFactoryName;
this.lastOperation = defaults.lastOperation;
this.nodes = defaults.nodes;
this.otherErrors = defaults.otherErrors;
this.state = defaults.state;
this.type = defaults.type;
}
@CustomType.Setter
public Builder createTime(String createTime) {
if (createTime == null) {
throw new MissingRequiredPropertyException("ManagedIntegrationRuntimeStatusResponse", "createTime");
}
this.createTime = createTime;
return this;
}
@CustomType.Setter
public Builder dataFactoryName(String dataFactoryName) {
if (dataFactoryName == null) {
throw new MissingRequiredPropertyException("ManagedIntegrationRuntimeStatusResponse", "dataFactoryName");
}
this.dataFactoryName = dataFactoryName;
return this;
}
@CustomType.Setter
public Builder lastOperation(ManagedIntegrationRuntimeOperationResultResponse lastOperation) {
if (lastOperation == null) {
throw new MissingRequiredPropertyException("ManagedIntegrationRuntimeStatusResponse", "lastOperation");
}
this.lastOperation = lastOperation;
return this;
}
@CustomType.Setter
public Builder nodes(List nodes) {
if (nodes == null) {
throw new MissingRequiredPropertyException("ManagedIntegrationRuntimeStatusResponse", "nodes");
}
this.nodes = nodes;
return this;
}
public Builder nodes(ManagedIntegrationRuntimeNodeResponse... nodes) {
return nodes(List.of(nodes));
}
@CustomType.Setter
public Builder otherErrors(List otherErrors) {
if (otherErrors == null) {
throw new MissingRequiredPropertyException("ManagedIntegrationRuntimeStatusResponse", "otherErrors");
}
this.otherErrors = otherErrors;
return this;
}
public Builder otherErrors(ManagedIntegrationRuntimeErrorResponse... otherErrors) {
return otherErrors(List.of(otherErrors));
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("ManagedIntegrationRuntimeStatusResponse", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("ManagedIntegrationRuntimeStatusResponse", "type");
}
this.type = type;
return this;
}
public ManagedIntegrationRuntimeStatusResponse build() {
final var _resultValue = new ManagedIntegrationRuntimeStatusResponse();
_resultValue.createTime = createTime;
_resultValue.dataFactoryName = dataFactoryName;
_resultValue.lastOperation = lastOperation;
_resultValue.nodes = nodes;
_resultValue.otherErrors = otherErrors;
_resultValue.state = state;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy