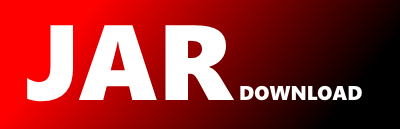
com.pulumi.azurenative.datafactory.outputs.SalesforceV2SinkResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class SalesforceV2SinkResponse {
/**
* @return If true, disable data store metrics collection. Default is false. Type: boolean (or Expression with resultType boolean).
*
*/
private @Nullable Object disableMetricsCollection;
/**
* @return The name of the external ID field for upsert operation. Default value is 'Id' column. Type: string (or Expression with resultType string).
*
*/
private @Nullable Object externalIdFieldName;
/**
* @return The flag indicating whether or not to ignore null values from input dataset (except key fields) during write operation. Default value is false. If set it to true, it means ADF will leave the data in the destination object unchanged when doing upsert/update operation and insert defined default value when doing insert operation, versus ADF will update the data in the destination object to NULL when doing upsert/update operation and insert NULL value when doing insert operation. Type: boolean (or Expression with resultType boolean).
*
*/
private @Nullable Object ignoreNullValues;
/**
* @return The maximum concurrent connection count for the sink data store. Type: integer (or Expression with resultType integer).
*
*/
private @Nullable Object maxConcurrentConnections;
/**
* @return Sink retry count. Type: integer (or Expression with resultType integer).
*
*/
private @Nullable Object sinkRetryCount;
/**
* @return Sink retry wait. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
*
*/
private @Nullable Object sinkRetryWait;
/**
* @return Copy sink type.
* Expected value is 'SalesforceV2Sink'.
*
*/
private String type;
/**
* @return Write batch size. Type: integer (or Expression with resultType integer), minimum: 0.
*
*/
private @Nullable Object writeBatchSize;
/**
* @return Write batch timeout. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
*
*/
private @Nullable Object writeBatchTimeout;
/**
* @return The write behavior for the operation. Default is Insert.
*
*/
private @Nullable String writeBehavior;
private SalesforceV2SinkResponse() {}
/**
* @return If true, disable data store metrics collection. Default is false. Type: boolean (or Expression with resultType boolean).
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy