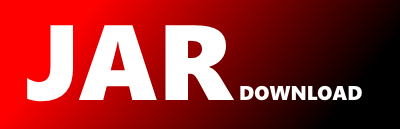
com.pulumi.azurenative.datalakeanalytics.AccountArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datalakeanalytics;
import com.pulumi.azurenative.datalakeanalytics.enums.FirewallAllowAzureIpsState;
import com.pulumi.azurenative.datalakeanalytics.enums.FirewallState;
import com.pulumi.azurenative.datalakeanalytics.enums.TierType;
import com.pulumi.azurenative.datalakeanalytics.inputs.AddDataLakeStoreWithAccountParametersArgs;
import com.pulumi.azurenative.datalakeanalytics.inputs.AddStorageAccountWithAccountParametersArgs;
import com.pulumi.azurenative.datalakeanalytics.inputs.CreateComputePolicyWithAccountParametersArgs;
import com.pulumi.azurenative.datalakeanalytics.inputs.CreateFirewallRuleWithAccountParametersArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AccountArgs extends com.pulumi.resources.ResourceArgs {
public static final AccountArgs Empty = new AccountArgs();
/**
* The name of the Data Lake Analytics account.
*
*/
@Import(name="accountName")
private @Nullable Output accountName;
/**
* @return The name of the Data Lake Analytics account.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy