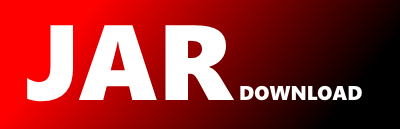
com.pulumi.azurenative.datalakeanalytics.outputs.HiveMetastoreResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datalakeanalytics.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class HiveMetastoreResponse {
/**
* @return The databaseName for the Hive MetaStore
*
*/
private String databaseName;
/**
* @return The resource identifier.
*
*/
private String id;
/**
* @return The resource name.
*
*/
private String name;
/**
* @return The current state of the NestedResource
*
*/
private String nestedResourceProvisioningState;
/**
* @return The password for the Hive MetaStore
*
*/
private String password;
/**
* @return The runtimeVersion for the Hive MetaStore
*
*/
private String runtimeVersion;
/**
* @return The serverUri for the Hive MetaStore
*
*/
private String serverUri;
/**
* @return The resource type.
*
*/
private String type;
/**
* @return The userName for the Hive MetaStore
*
*/
private String userName;
private HiveMetastoreResponse() {}
/**
* @return The databaseName for the Hive MetaStore
*
*/
public String databaseName() {
return this.databaseName;
}
/**
* @return The resource identifier.
*
*/
public String id() {
return this.id;
}
/**
* @return The resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return The current state of the NestedResource
*
*/
public String nestedResourceProvisioningState() {
return this.nestedResourceProvisioningState;
}
/**
* @return The password for the Hive MetaStore
*
*/
public String password() {
return this.password;
}
/**
* @return The runtimeVersion for the Hive MetaStore
*
*/
public String runtimeVersion() {
return this.runtimeVersion;
}
/**
* @return The serverUri for the Hive MetaStore
*
*/
public String serverUri() {
return this.serverUri;
}
/**
* @return The resource type.
*
*/
public String type() {
return this.type;
}
/**
* @return The userName for the Hive MetaStore
*
*/
public String userName() {
return this.userName;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(HiveMetastoreResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String databaseName;
private String id;
private String name;
private String nestedResourceProvisioningState;
private String password;
private String runtimeVersion;
private String serverUri;
private String type;
private String userName;
public Builder() {}
public Builder(HiveMetastoreResponse defaults) {
Objects.requireNonNull(defaults);
this.databaseName = defaults.databaseName;
this.id = defaults.id;
this.name = defaults.name;
this.nestedResourceProvisioningState = defaults.nestedResourceProvisioningState;
this.password = defaults.password;
this.runtimeVersion = defaults.runtimeVersion;
this.serverUri = defaults.serverUri;
this.type = defaults.type;
this.userName = defaults.userName;
}
@CustomType.Setter
public Builder databaseName(String databaseName) {
if (databaseName == null) {
throw new MissingRequiredPropertyException("HiveMetastoreResponse", "databaseName");
}
this.databaseName = databaseName;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("HiveMetastoreResponse", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("HiveMetastoreResponse", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder nestedResourceProvisioningState(String nestedResourceProvisioningState) {
if (nestedResourceProvisioningState == null) {
throw new MissingRequiredPropertyException("HiveMetastoreResponse", "nestedResourceProvisioningState");
}
this.nestedResourceProvisioningState = nestedResourceProvisioningState;
return this;
}
@CustomType.Setter
public Builder password(String password) {
if (password == null) {
throw new MissingRequiredPropertyException("HiveMetastoreResponse", "password");
}
this.password = password;
return this;
}
@CustomType.Setter
public Builder runtimeVersion(String runtimeVersion) {
if (runtimeVersion == null) {
throw new MissingRequiredPropertyException("HiveMetastoreResponse", "runtimeVersion");
}
this.runtimeVersion = runtimeVersion;
return this;
}
@CustomType.Setter
public Builder serverUri(String serverUri) {
if (serverUri == null) {
throw new MissingRequiredPropertyException("HiveMetastoreResponse", "serverUri");
}
this.serverUri = serverUri;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("HiveMetastoreResponse", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder userName(String userName) {
if (userName == null) {
throw new MissingRequiredPropertyException("HiveMetastoreResponse", "userName");
}
this.userName = userName;
return this;
}
public HiveMetastoreResponse build() {
final var _resultValue = new HiveMetastoreResponse();
_resultValue.databaseName = databaseName;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.nestedResourceProvisioningState = nestedResourceProvisioningState;
_resultValue.password = password;
_resultValue.runtimeVersion = runtimeVersion;
_resultValue.serverUri = serverUri;
_resultValue.type = type;
_resultValue.userName = userName;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy