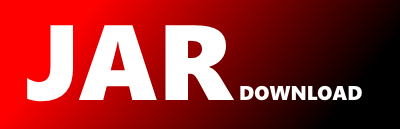
com.pulumi.azurenative.datareplication.DatareplicationFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datareplication;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.datareplication.inputs.GetDraArgs;
import com.pulumi.azurenative.datareplication.inputs.GetDraPlainArgs;
import com.pulumi.azurenative.datareplication.inputs.GetFabricArgs;
import com.pulumi.azurenative.datareplication.inputs.GetFabricPlainArgs;
import com.pulumi.azurenative.datareplication.inputs.GetPolicyArgs;
import com.pulumi.azurenative.datareplication.inputs.GetPolicyPlainArgs;
import com.pulumi.azurenative.datareplication.inputs.GetProtectedItemArgs;
import com.pulumi.azurenative.datareplication.inputs.GetProtectedItemPlainArgs;
import com.pulumi.azurenative.datareplication.inputs.GetReplicationExtensionArgs;
import com.pulumi.azurenative.datareplication.inputs.GetReplicationExtensionPlainArgs;
import com.pulumi.azurenative.datareplication.inputs.GetVaultArgs;
import com.pulumi.azurenative.datareplication.inputs.GetVaultPlainArgs;
import com.pulumi.azurenative.datareplication.outputs.GetDraResult;
import com.pulumi.azurenative.datareplication.outputs.GetFabricResult;
import com.pulumi.azurenative.datareplication.outputs.GetPolicyResult;
import com.pulumi.azurenative.datareplication.outputs.GetProtectedItemResult;
import com.pulumi.azurenative.datareplication.outputs.GetReplicationExtensionResult;
import com.pulumi.azurenative.datareplication.outputs.GetVaultResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class DatareplicationFunctions {
/**
* Gets the details of the fabric agent.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static Output getDra(GetDraArgs args) {
return getDra(args, InvokeOptions.Empty);
}
/**
* Gets the details of the fabric agent.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static CompletableFuture getDraPlain(GetDraPlainArgs args) {
return getDraPlain(args, InvokeOptions.Empty);
}
/**
* Gets the details of the fabric agent.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static Output getDra(GetDraArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:datareplication:getDra", TypeShape.of(GetDraResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the details of the fabric agent.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static CompletableFuture getDraPlain(GetDraPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:datareplication:getDra", TypeShape.of(GetDraResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the details of the fabric.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static Output getFabric(GetFabricArgs args) {
return getFabric(args, InvokeOptions.Empty);
}
/**
* Gets the details of the fabric.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static CompletableFuture getFabricPlain(GetFabricPlainArgs args) {
return getFabricPlain(args, InvokeOptions.Empty);
}
/**
* Gets the details of the fabric.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static Output getFabric(GetFabricArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:datareplication:getFabric", TypeShape.of(GetFabricResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the details of the fabric.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static CompletableFuture getFabricPlain(GetFabricPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:datareplication:getFabric", TypeShape.of(GetFabricResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the details of the policy.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static Output getPolicy(GetPolicyArgs args) {
return getPolicy(args, InvokeOptions.Empty);
}
/**
* Gets the details of the policy.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static CompletableFuture getPolicyPlain(GetPolicyPlainArgs args) {
return getPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Gets the details of the policy.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static Output getPolicy(GetPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:datareplication:getPolicy", TypeShape.of(GetPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the details of the policy.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static CompletableFuture getPolicyPlain(GetPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:datareplication:getPolicy", TypeShape.of(GetPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the details of the protected item.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static Output getProtectedItem(GetProtectedItemArgs args) {
return getProtectedItem(args, InvokeOptions.Empty);
}
/**
* Gets the details of the protected item.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static CompletableFuture getProtectedItemPlain(GetProtectedItemPlainArgs args) {
return getProtectedItemPlain(args, InvokeOptions.Empty);
}
/**
* Gets the details of the protected item.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static Output getProtectedItem(GetProtectedItemArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:datareplication:getProtectedItem", TypeShape.of(GetProtectedItemResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the details of the protected item.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static CompletableFuture getProtectedItemPlain(GetProtectedItemPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:datareplication:getProtectedItem", TypeShape.of(GetProtectedItemResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the details of the replication extension.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static Output getReplicationExtension(GetReplicationExtensionArgs args) {
return getReplicationExtension(args, InvokeOptions.Empty);
}
/**
* Gets the details of the replication extension.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static CompletableFuture getReplicationExtensionPlain(GetReplicationExtensionPlainArgs args) {
return getReplicationExtensionPlain(args, InvokeOptions.Empty);
}
/**
* Gets the details of the replication extension.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static Output getReplicationExtension(GetReplicationExtensionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:datareplication:getReplicationExtension", TypeShape.of(GetReplicationExtensionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the details of the replication extension.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static CompletableFuture getReplicationExtensionPlain(GetReplicationExtensionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:datareplication:getReplicationExtension", TypeShape.of(GetReplicationExtensionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the details of the vault.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static Output getVault(GetVaultArgs args) {
return getVault(args, InvokeOptions.Empty);
}
/**
* Gets the details of the vault.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static CompletableFuture getVaultPlain(GetVaultPlainArgs args) {
return getVaultPlain(args, InvokeOptions.Empty);
}
/**
* Gets the details of the vault.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static Output getVault(GetVaultArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:datareplication:getVault", TypeShape.of(GetVaultResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the details of the vault.
* Azure REST API version: 2021-02-16-preview.
*
*/
public static CompletableFuture getVaultPlain(GetVaultPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:datareplication:getVault", TypeShape.of(GetVaultResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy