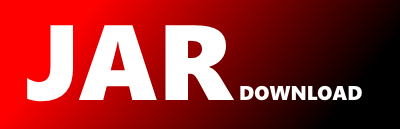
com.pulumi.azurenative.desktopvirtualization.HostPool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.desktopvirtualization;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.desktopvirtualization.HostPoolArgs;
import com.pulumi.azurenative.desktopvirtualization.outputs.AgentUpdatePropertiesResponse;
import com.pulumi.azurenative.desktopvirtualization.outputs.RegistrationInfoResponse;
import com.pulumi.azurenative.desktopvirtualization.outputs.ResourceModelWithAllowedPropertySetResponseIdentity;
import com.pulumi.azurenative.desktopvirtualization.outputs.ResourceModelWithAllowedPropertySetResponsePlan;
import com.pulumi.azurenative.desktopvirtualization.outputs.ResourceModelWithAllowedPropertySetResponseSku;
import com.pulumi.azurenative.desktopvirtualization.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Represents a HostPool definition.
* Azure REST API version: 2022-09-09. Prior API version in Azure Native 1.x: 2021-02-01-preview.
*
* Other available API versions: 2020-11-02-preview, 2020-11-10-preview, 2022-04-01-preview, 2022-10-14-preview, 2023-07-07-preview, 2023-09-05, 2023-10-04-preview, 2023-11-01-preview, 2024-01-16-preview, 2024-03-06-preview, 2024-04-03, 2024-04-08-preview.
*
* ## Example Usage
* ### HostPool_Create
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.desktopvirtualization.HostPool;
* import com.pulumi.azurenative.desktopvirtualization.HostPoolArgs;
* import com.pulumi.azurenative.desktopvirtualization.inputs.AgentUpdatePropertiesArgs;
* import com.pulumi.azurenative.desktopvirtualization.inputs.RegistrationInfoArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var hostPool = new HostPool("hostPool", HostPoolArgs.builder()
* .agentUpdate(AgentUpdatePropertiesArgs.builder()
* .maintenanceWindowTimeZone("Alaskan Standard Time")
* .maintenanceWindows(
* MaintenanceWindowPropertiesArgs.builder()
* .dayOfWeek("Friday")
* .hour(7)
* .build(),
* MaintenanceWindowPropertiesArgs.builder()
* .dayOfWeek("Saturday")
* .hour(8)
* .build())
* .type("Scheduled")
* .useSessionHostLocalTime(false)
* .build())
* .description("des1")
* .friendlyName("friendly")
* .hostPoolName("hostPool1")
* .hostPoolType("Pooled")
* .loadBalancerType("BreadthFirst")
* .location("centralus")
* .maxSessionLimit(999999)
* .personalDesktopAssignmentType("Automatic")
* .preferredAppGroupType("Desktop")
* .registrationInfo(RegistrationInfoArgs.builder()
* .expirationTime("2020-10-01T14:01:54.9571247Z")
* .registrationTokenOperation("Update")
* .build())
* .resourceGroupName("resourceGroup1")
* .ssoClientId("client")
* .ssoClientSecretKeyVaultPath("https://keyvault/secret")
* .ssoSecretType("SharedKey")
* .ssoadfsAuthority("https://adfs")
* .startVMOnConnect(false)
* .tags(Map.ofEntries(
* Map.entry("tag1", "value1"),
* Map.entry("tag2", "value2")
* ))
* .vmTemplate("{json:json}")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:desktopvirtualization:HostPool hostPool1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DesktopVirtualization/hostPools/{hostPoolName}
* ```
*
*/
@ResourceType(type="azure-native:desktopvirtualization:HostPool")
public class HostPool extends com.pulumi.resources.CustomResource {
/**
* The session host configuration for updating agent, monitoring agent, and stack component.
*
*/
@Export(name="agentUpdate", refs={AgentUpdatePropertiesResponse.class}, tree="[0]")
private Output* @Nullable */ AgentUpdatePropertiesResponse> agentUpdate;
/**
* @return The session host configuration for updating agent, monitoring agent, and stack component.
*
*/
public Output> agentUpdate() {
return Codegen.optional(this.agentUpdate);
}
/**
* List of applicationGroup links.
*
*/
@Export(name="applicationGroupReferences", refs={List.class,String.class}, tree="[0,1]")
private Output> applicationGroupReferences;
/**
* @return List of applicationGroup links.
*
*/
public Output> applicationGroupReferences() {
return this.applicationGroupReferences;
}
/**
* Is cloud pc resource.
*
*/
@Export(name="cloudPcResource", refs={Boolean.class}, tree="[0]")
private Output cloudPcResource;
/**
* @return Is cloud pc resource.
*
*/
public Output cloudPcResource() {
return this.cloudPcResource;
}
/**
* Custom rdp property of HostPool.
*
*/
@Export(name="customRdpProperty", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> customRdpProperty;
/**
* @return Custom rdp property of HostPool.
*
*/
public Output> customRdpProperty() {
return Codegen.optional(this.customRdpProperty);
}
/**
* Description of HostPool.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Description of HostPool.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* The etag field is *not* required. If it is provided in the response body, it must also be provided as a header per the normal etag convention. Entity tags are used for comparing two or more entities from the same requested resource. HTTP/1.1 uses entity tags in the etag (section 14.19), If-Match (section 14.24), If-None-Match (section 14.26), and If-Range (section 14.27) header fields.
*
*/
@Export(name="etag", refs={String.class}, tree="[0]")
private Output etag;
/**
* @return The etag field is *not* required. If it is provided in the response body, it must also be provided as a header per the normal etag convention. Entity tags are used for comparing two or more entities from the same requested resource. HTTP/1.1 uses entity tags in the etag (section 14.19), If-Match (section 14.24), If-None-Match (section 14.26), and If-Range (section 14.27) header fields.
*
*/
public Output etag() {
return this.etag;
}
/**
* Friendly name of HostPool.
*
*/
@Export(name="friendlyName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> friendlyName;
/**
* @return Friendly name of HostPool.
*
*/
public Output> friendlyName() {
return Codegen.optional(this.friendlyName);
}
/**
* HostPool type for desktop.
*
*/
@Export(name="hostPoolType", refs={String.class}, tree="[0]")
private Output hostPoolType;
/**
* @return HostPool type for desktop.
*
*/
public Output hostPoolType() {
return this.hostPoolType;
}
@Export(name="identity", refs={ResourceModelWithAllowedPropertySetResponseIdentity.class}, tree="[0]")
private Output* @Nullable */ ResourceModelWithAllowedPropertySetResponseIdentity> identity;
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* Metadata used by portal/tooling/etc to render different UX experiences for resources of the same type; e.g. ApiApps are a kind of Microsoft.Web/sites type. If supported, the resource provider must validate and persist this value.
*
*/
@Export(name="kind", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> kind;
/**
* @return Metadata used by portal/tooling/etc to render different UX experiences for resources of the same type; e.g. ApiApps are a kind of Microsoft.Web/sites type. If supported, the resource provider must validate and persist this value.
*
*/
public Output> kind() {
return Codegen.optional(this.kind);
}
/**
* The type of the load balancer.
*
*/
@Export(name="loadBalancerType", refs={String.class}, tree="[0]")
private Output loadBalancerType;
/**
* @return The type of the load balancer.
*
*/
public Output loadBalancerType() {
return this.loadBalancerType;
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> location;
/**
* @return The geo-location where the resource lives
*
*/
public Output> location() {
return Codegen.optional(this.location);
}
/**
* The fully qualified resource ID of the resource that manages this resource. Indicates if this resource is managed by another Azure resource. If this is present, complete mode deployment will not delete the resource if it is removed from the template since it is managed by another resource.
*
*/
@Export(name="managedBy", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> managedBy;
/**
* @return The fully qualified resource ID of the resource that manages this resource. Indicates if this resource is managed by another Azure resource. If this is present, complete mode deployment will not delete the resource if it is removed from the template since it is managed by another resource.
*
*/
public Output> managedBy() {
return Codegen.optional(this.managedBy);
}
/**
* The max session limit of HostPool.
*
*/
@Export(name="maxSessionLimit", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> maxSessionLimit;
/**
* @return The max session limit of HostPool.
*
*/
public Output> maxSessionLimit() {
return Codegen.optional(this.maxSessionLimit);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* ObjectId of HostPool. (internal use)
*
*/
@Export(name="objectId", refs={String.class}, tree="[0]")
private Output objectId;
/**
* @return ObjectId of HostPool. (internal use)
*
*/
public Output objectId() {
return this.objectId;
}
/**
* PersonalDesktopAssignment type for HostPool.
*
*/
@Export(name="personalDesktopAssignmentType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> personalDesktopAssignmentType;
/**
* @return PersonalDesktopAssignment type for HostPool.
*
*/
public Output> personalDesktopAssignmentType() {
return Codegen.optional(this.personalDesktopAssignmentType);
}
@Export(name="plan", refs={ResourceModelWithAllowedPropertySetResponsePlan.class}, tree="[0]")
private Output* @Nullable */ ResourceModelWithAllowedPropertySetResponsePlan> plan;
public Output> plan() {
return Codegen.optional(this.plan);
}
/**
* The type of preferred application group type, default to Desktop Application Group
*
*/
@Export(name="preferredAppGroupType", refs={String.class}, tree="[0]")
private Output preferredAppGroupType;
/**
* @return The type of preferred application group type, default to Desktop Application Group
*
*/
public Output preferredAppGroupType() {
return this.preferredAppGroupType;
}
/**
* The registration info of HostPool.
*
*/
@Export(name="registrationInfo", refs={RegistrationInfoResponse.class}, tree="[0]")
private Output* @Nullable */ RegistrationInfoResponse> registrationInfo;
/**
* @return The registration info of HostPool.
*
*/
public Output> registrationInfo() {
return Codegen.optional(this.registrationInfo);
}
/**
* The ring number of HostPool.
*
*/
@Export(name="ring", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> ring;
/**
* @return The ring number of HostPool.
*
*/
public Output> ring() {
return Codegen.optional(this.ring);
}
@Export(name="sku", refs={ResourceModelWithAllowedPropertySetResponseSku.class}, tree="[0]")
private Output* @Nullable */ ResourceModelWithAllowedPropertySetResponseSku> sku;
public Output> sku() {
return Codegen.optional(this.sku);
}
/**
* ClientId for the registered Relying Party used to issue WVD SSO certificates.
*
*/
@Export(name="ssoClientId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> ssoClientId;
/**
* @return ClientId for the registered Relying Party used to issue WVD SSO certificates.
*
*/
public Output> ssoClientId() {
return Codegen.optional(this.ssoClientId);
}
/**
* Path to Azure KeyVault storing the secret used for communication to ADFS.
*
*/
@Export(name="ssoClientSecretKeyVaultPath", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> ssoClientSecretKeyVaultPath;
/**
* @return Path to Azure KeyVault storing the secret used for communication to ADFS.
*
*/
public Output> ssoClientSecretKeyVaultPath() {
return Codegen.optional(this.ssoClientSecretKeyVaultPath);
}
/**
* The type of single sign on Secret Type.
*
*/
@Export(name="ssoSecretType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> ssoSecretType;
/**
* @return The type of single sign on Secret Type.
*
*/
public Output> ssoSecretType() {
return Codegen.optional(this.ssoSecretType);
}
/**
* URL to customer ADFS server for signing WVD SSO certificates.
*
*/
@Export(name="ssoadfsAuthority", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> ssoadfsAuthority;
/**
* @return URL to customer ADFS server for signing WVD SSO certificates.
*
*/
public Output> ssoadfsAuthority() {
return Codegen.optional(this.ssoadfsAuthority);
}
/**
* The flag to turn on/off StartVMOnConnect feature.
*
*/
@Export(name="startVMOnConnect", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> startVMOnConnect;
/**
* @return The flag to turn on/off StartVMOnConnect feature.
*
*/
public Output> startVMOnConnect() {
return Codegen.optional(this.startVMOnConnect);
}
/**
* Metadata pertaining to creation and last modification of the resource.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
* Is validation environment.
*
*/
@Export(name="validationEnvironment", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> validationEnvironment;
/**
* @return Is validation environment.
*
*/
public Output> validationEnvironment() {
return Codegen.optional(this.validationEnvironment);
}
/**
* VM template for sessionhosts configuration within hostpool.
*
*/
@Export(name="vmTemplate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> vmTemplate;
/**
* @return VM template for sessionhosts configuration within hostpool.
*
*/
public Output> vmTemplate() {
return Codegen.optional(this.vmTemplate);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public HostPool(java.lang.String name) {
this(name, HostPoolArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public HostPool(java.lang.String name, HostPoolArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public HostPool(java.lang.String name, HostPoolArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:desktopvirtualization:HostPool", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private HostPool(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:desktopvirtualization:HostPool", name, null, makeResourceOptions(options, id), false);
}
private static HostPoolArgs makeArgs(HostPoolArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? HostPoolArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20190123preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20190924preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20191210preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20200921preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20201019preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20201102preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20201110preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20210114preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20210201preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20210309preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20210401preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20210712:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20210903preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20220210preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20220401preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20220909:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20221014preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20230707preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20230905:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20231004preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20231101preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20240116preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20240306preview:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20240403:HostPool").build()),
Output.of(Alias.builder().type("azure-native:desktopvirtualization/v20240408preview:HostPool").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static HostPool get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new HostPool(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy