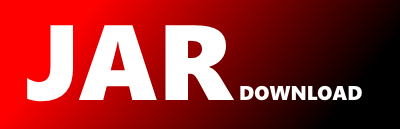
com.pulumi.azurenative.desktopvirtualization.outputs.GetScalingPlanPooledScheduleResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.desktopvirtualization.outputs;
import com.pulumi.azurenative.desktopvirtualization.outputs.SystemDataResponse;
import com.pulumi.azurenative.desktopvirtualization.outputs.TimeResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetScalingPlanPooledScheduleResult {
/**
* @return Set of days of the week on which this schedule is active.
*
*/
private @Nullable List daysOfWeek;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Load balancing algorithm for off-peak period.
*
*/
private @Nullable String offPeakLoadBalancingAlgorithm;
/**
* @return Starting time for off-peak period.
*
*/
private @Nullable TimeResponse offPeakStartTime;
/**
* @return Load balancing algorithm for peak period.
*
*/
private @Nullable String peakLoadBalancingAlgorithm;
/**
* @return Starting time for peak period.
*
*/
private @Nullable TimeResponse peakStartTime;
/**
* @return Capacity threshold for ramp down period.
*
*/
private @Nullable Integer rampDownCapacityThresholdPct;
/**
* @return Should users be logged off forcefully from hosts.
*
*/
private @Nullable Boolean rampDownForceLogoffUsers;
/**
* @return Load balancing algorithm for ramp down period.
*
*/
private @Nullable String rampDownLoadBalancingAlgorithm;
/**
* @return Minimum host percentage for ramp down period.
*
*/
private @Nullable Integer rampDownMinimumHostsPct;
/**
* @return Notification message for users during ramp down period.
*
*/
private @Nullable String rampDownNotificationMessage;
/**
* @return Starting time for ramp down period.
*
*/
private @Nullable TimeResponse rampDownStartTime;
/**
* @return Specifies when to stop hosts during ramp down period.
*
*/
private @Nullable String rampDownStopHostsWhen;
/**
* @return Number of minutes to wait to stop hosts during ramp down period.
*
*/
private @Nullable Integer rampDownWaitTimeMinutes;
/**
* @return Capacity threshold for ramp up period.
*
*/
private @Nullable Integer rampUpCapacityThresholdPct;
/**
* @return Load balancing algorithm for ramp up period.
*
*/
private @Nullable String rampUpLoadBalancingAlgorithm;
/**
* @return Minimum host percentage for ramp up period.
*
*/
private @Nullable Integer rampUpMinimumHostsPct;
/**
* @return Starting time for ramp up period.
*
*/
private @Nullable TimeResponse rampUpStartTime;
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
private SystemDataResponse systemData;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetScalingPlanPooledScheduleResult() {}
/**
* @return Set of days of the week on which this schedule is active.
*
*/
public List daysOfWeek() {
return this.daysOfWeek == null ? List.of() : this.daysOfWeek;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Load balancing algorithm for off-peak period.
*
*/
public Optional offPeakLoadBalancingAlgorithm() {
return Optional.ofNullable(this.offPeakLoadBalancingAlgorithm);
}
/**
* @return Starting time for off-peak period.
*
*/
public Optional offPeakStartTime() {
return Optional.ofNullable(this.offPeakStartTime);
}
/**
* @return Load balancing algorithm for peak period.
*
*/
public Optional peakLoadBalancingAlgorithm() {
return Optional.ofNullable(this.peakLoadBalancingAlgorithm);
}
/**
* @return Starting time for peak period.
*
*/
public Optional peakStartTime() {
return Optional.ofNullable(this.peakStartTime);
}
/**
* @return Capacity threshold for ramp down period.
*
*/
public Optional rampDownCapacityThresholdPct() {
return Optional.ofNullable(this.rampDownCapacityThresholdPct);
}
/**
* @return Should users be logged off forcefully from hosts.
*
*/
public Optional rampDownForceLogoffUsers() {
return Optional.ofNullable(this.rampDownForceLogoffUsers);
}
/**
* @return Load balancing algorithm for ramp down period.
*
*/
public Optional rampDownLoadBalancingAlgorithm() {
return Optional.ofNullable(this.rampDownLoadBalancingAlgorithm);
}
/**
* @return Minimum host percentage for ramp down period.
*
*/
public Optional rampDownMinimumHostsPct() {
return Optional.ofNullable(this.rampDownMinimumHostsPct);
}
/**
* @return Notification message for users during ramp down period.
*
*/
public Optional rampDownNotificationMessage() {
return Optional.ofNullable(this.rampDownNotificationMessage);
}
/**
* @return Starting time for ramp down period.
*
*/
public Optional rampDownStartTime() {
return Optional.ofNullable(this.rampDownStartTime);
}
/**
* @return Specifies when to stop hosts during ramp down period.
*
*/
public Optional rampDownStopHostsWhen() {
return Optional.ofNullable(this.rampDownStopHostsWhen);
}
/**
* @return Number of minutes to wait to stop hosts during ramp down period.
*
*/
public Optional rampDownWaitTimeMinutes() {
return Optional.ofNullable(this.rampDownWaitTimeMinutes);
}
/**
* @return Capacity threshold for ramp up period.
*
*/
public Optional rampUpCapacityThresholdPct() {
return Optional.ofNullable(this.rampUpCapacityThresholdPct);
}
/**
* @return Load balancing algorithm for ramp up period.
*
*/
public Optional rampUpLoadBalancingAlgorithm() {
return Optional.ofNullable(this.rampUpLoadBalancingAlgorithm);
}
/**
* @return Minimum host percentage for ramp up period.
*
*/
public Optional rampUpMinimumHostsPct() {
return Optional.ofNullable(this.rampUpMinimumHostsPct);
}
/**
* @return Starting time for ramp up period.
*
*/
public Optional rampUpStartTime() {
return Optional.ofNullable(this.rampUpStartTime);
}
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetScalingPlanPooledScheduleResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List daysOfWeek;
private String id;
private String name;
private @Nullable String offPeakLoadBalancingAlgorithm;
private @Nullable TimeResponse offPeakStartTime;
private @Nullable String peakLoadBalancingAlgorithm;
private @Nullable TimeResponse peakStartTime;
private @Nullable Integer rampDownCapacityThresholdPct;
private @Nullable Boolean rampDownForceLogoffUsers;
private @Nullable String rampDownLoadBalancingAlgorithm;
private @Nullable Integer rampDownMinimumHostsPct;
private @Nullable String rampDownNotificationMessage;
private @Nullable TimeResponse rampDownStartTime;
private @Nullable String rampDownStopHostsWhen;
private @Nullable Integer rampDownWaitTimeMinutes;
private @Nullable Integer rampUpCapacityThresholdPct;
private @Nullable String rampUpLoadBalancingAlgorithm;
private @Nullable Integer rampUpMinimumHostsPct;
private @Nullable TimeResponse rampUpStartTime;
private SystemDataResponse systemData;
private String type;
public Builder() {}
public Builder(GetScalingPlanPooledScheduleResult defaults) {
Objects.requireNonNull(defaults);
this.daysOfWeek = defaults.daysOfWeek;
this.id = defaults.id;
this.name = defaults.name;
this.offPeakLoadBalancingAlgorithm = defaults.offPeakLoadBalancingAlgorithm;
this.offPeakStartTime = defaults.offPeakStartTime;
this.peakLoadBalancingAlgorithm = defaults.peakLoadBalancingAlgorithm;
this.peakStartTime = defaults.peakStartTime;
this.rampDownCapacityThresholdPct = defaults.rampDownCapacityThresholdPct;
this.rampDownForceLogoffUsers = defaults.rampDownForceLogoffUsers;
this.rampDownLoadBalancingAlgorithm = defaults.rampDownLoadBalancingAlgorithm;
this.rampDownMinimumHostsPct = defaults.rampDownMinimumHostsPct;
this.rampDownNotificationMessage = defaults.rampDownNotificationMessage;
this.rampDownStartTime = defaults.rampDownStartTime;
this.rampDownStopHostsWhen = defaults.rampDownStopHostsWhen;
this.rampDownWaitTimeMinutes = defaults.rampDownWaitTimeMinutes;
this.rampUpCapacityThresholdPct = defaults.rampUpCapacityThresholdPct;
this.rampUpLoadBalancingAlgorithm = defaults.rampUpLoadBalancingAlgorithm;
this.rampUpMinimumHostsPct = defaults.rampUpMinimumHostsPct;
this.rampUpStartTime = defaults.rampUpStartTime;
this.systemData = defaults.systemData;
this.type = defaults.type;
}
@CustomType.Setter
public Builder daysOfWeek(@Nullable List daysOfWeek) {
this.daysOfWeek = daysOfWeek;
return this;
}
public Builder daysOfWeek(String... daysOfWeek) {
return daysOfWeek(List.of(daysOfWeek));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetScalingPlanPooledScheduleResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetScalingPlanPooledScheduleResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder offPeakLoadBalancingAlgorithm(@Nullable String offPeakLoadBalancingAlgorithm) {
this.offPeakLoadBalancingAlgorithm = offPeakLoadBalancingAlgorithm;
return this;
}
@CustomType.Setter
public Builder offPeakStartTime(@Nullable TimeResponse offPeakStartTime) {
this.offPeakStartTime = offPeakStartTime;
return this;
}
@CustomType.Setter
public Builder peakLoadBalancingAlgorithm(@Nullable String peakLoadBalancingAlgorithm) {
this.peakLoadBalancingAlgorithm = peakLoadBalancingAlgorithm;
return this;
}
@CustomType.Setter
public Builder peakStartTime(@Nullable TimeResponse peakStartTime) {
this.peakStartTime = peakStartTime;
return this;
}
@CustomType.Setter
public Builder rampDownCapacityThresholdPct(@Nullable Integer rampDownCapacityThresholdPct) {
this.rampDownCapacityThresholdPct = rampDownCapacityThresholdPct;
return this;
}
@CustomType.Setter
public Builder rampDownForceLogoffUsers(@Nullable Boolean rampDownForceLogoffUsers) {
this.rampDownForceLogoffUsers = rampDownForceLogoffUsers;
return this;
}
@CustomType.Setter
public Builder rampDownLoadBalancingAlgorithm(@Nullable String rampDownLoadBalancingAlgorithm) {
this.rampDownLoadBalancingAlgorithm = rampDownLoadBalancingAlgorithm;
return this;
}
@CustomType.Setter
public Builder rampDownMinimumHostsPct(@Nullable Integer rampDownMinimumHostsPct) {
this.rampDownMinimumHostsPct = rampDownMinimumHostsPct;
return this;
}
@CustomType.Setter
public Builder rampDownNotificationMessage(@Nullable String rampDownNotificationMessage) {
this.rampDownNotificationMessage = rampDownNotificationMessage;
return this;
}
@CustomType.Setter
public Builder rampDownStartTime(@Nullable TimeResponse rampDownStartTime) {
this.rampDownStartTime = rampDownStartTime;
return this;
}
@CustomType.Setter
public Builder rampDownStopHostsWhen(@Nullable String rampDownStopHostsWhen) {
this.rampDownStopHostsWhen = rampDownStopHostsWhen;
return this;
}
@CustomType.Setter
public Builder rampDownWaitTimeMinutes(@Nullable Integer rampDownWaitTimeMinutes) {
this.rampDownWaitTimeMinutes = rampDownWaitTimeMinutes;
return this;
}
@CustomType.Setter
public Builder rampUpCapacityThresholdPct(@Nullable Integer rampUpCapacityThresholdPct) {
this.rampUpCapacityThresholdPct = rampUpCapacityThresholdPct;
return this;
}
@CustomType.Setter
public Builder rampUpLoadBalancingAlgorithm(@Nullable String rampUpLoadBalancingAlgorithm) {
this.rampUpLoadBalancingAlgorithm = rampUpLoadBalancingAlgorithm;
return this;
}
@CustomType.Setter
public Builder rampUpMinimumHostsPct(@Nullable Integer rampUpMinimumHostsPct) {
this.rampUpMinimumHostsPct = rampUpMinimumHostsPct;
return this;
}
@CustomType.Setter
public Builder rampUpStartTime(@Nullable TimeResponse rampUpStartTime) {
this.rampUpStartTime = rampUpStartTime;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetScalingPlanPooledScheduleResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetScalingPlanPooledScheduleResult", "type");
}
this.type = type;
return this;
}
public GetScalingPlanPooledScheduleResult build() {
final var _resultValue = new GetScalingPlanPooledScheduleResult();
_resultValue.daysOfWeek = daysOfWeek;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.offPeakLoadBalancingAlgorithm = offPeakLoadBalancingAlgorithm;
_resultValue.offPeakStartTime = offPeakStartTime;
_resultValue.peakLoadBalancingAlgorithm = peakLoadBalancingAlgorithm;
_resultValue.peakStartTime = peakStartTime;
_resultValue.rampDownCapacityThresholdPct = rampDownCapacityThresholdPct;
_resultValue.rampDownForceLogoffUsers = rampDownForceLogoffUsers;
_resultValue.rampDownLoadBalancingAlgorithm = rampDownLoadBalancingAlgorithm;
_resultValue.rampDownMinimumHostsPct = rampDownMinimumHostsPct;
_resultValue.rampDownNotificationMessage = rampDownNotificationMessage;
_resultValue.rampDownStartTime = rampDownStartTime;
_resultValue.rampDownStopHostsWhen = rampDownStopHostsWhen;
_resultValue.rampDownWaitTimeMinutes = rampDownWaitTimeMinutes;
_resultValue.rampUpCapacityThresholdPct = rampUpCapacityThresholdPct;
_resultValue.rampUpLoadBalancingAlgorithm = rampUpLoadBalancingAlgorithm;
_resultValue.rampUpMinimumHostsPct = rampUpMinimumHostsPct;
_resultValue.rampUpStartTime = rampUpStartTime;
_resultValue.systemData = systemData;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy