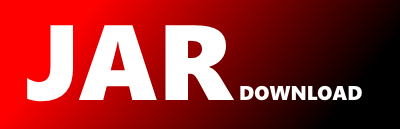
com.pulumi.azurenative.devhub.IacProfile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.devhub;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.devhub.IacProfileArgs;
import com.pulumi.azurenative.devhub.outputs.IacTemplatePropertiesResponse;
import com.pulumi.azurenative.devhub.outputs.StagePropertiesResponse;
import com.pulumi.azurenative.devhub.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Resource representation of a IacProfile.
* Azure REST API version: 2024-05-01-preview.
*
* Other available API versions: 2024-08-01-preview.
*
* ## Example Usage
* ### Create IacProfile
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.devhub.IacProfile;
* import com.pulumi.azurenative.devhub.IacProfileArgs;
* import com.pulumi.azurenative.devhub.inputs.StagePropertiesArgs;
* import com.pulumi.azurenative.devhub.inputs.IacTemplatePropertiesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var iacProfile = new IacProfile("iacProfile", IacProfileArgs.builder()
* .iacProfileName("profile1")
* .location("eastus")
* .repositoryName("localtest")
* .repositoryOwner("qfai")
* .resourceGroupName("resourceGroup1")
* .stages(
* StagePropertiesArgs.builder()
* .dependencies()
* .gitEnvironment("Terraform")
* .stageName("dev")
* .build(),
* StagePropertiesArgs.builder()
* .dependencies("dev")
* .gitEnvironment("Terraform")
* .stageName("qa")
* .build())
* .storageAccountName("iacbackend")
* .storageAccountResourceGroup("test")
* .storageAccountSubscription("586c20df-c465-4f10-8673-65aa4859e7ca")
* .storageContainerName("tfbackend")
* .templates(IacTemplatePropertiesArgs.builder()
* .instanceName("quickinstance")
* .instanceStage("dev")
* .quickStartTemplateType("HCIAKS")
* .templateName("hciaksss")
* .build())
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:devhub:IacProfile profile1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DevHub/iacProfiles/{iacProfileName}
* ```
*
*/
@ResourceType(type="azure-native:devhub:IacProfile")
public class IacProfile extends com.pulumi.resources.CustomResource {
/**
* Determines the authorization status of requests.
*
*/
@Export(name="authStatus", refs={String.class}, tree="[0]")
private Output authStatus;
/**
* @return Determines the authorization status of requests.
*
*/
public Output authStatus() {
return this.authStatus;
}
/**
* Repository Branch Name
*
*/
@Export(name="branchName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> branchName;
/**
* @return Repository Branch Name
*
*/
public Output> branchName() {
return Codegen.optional(this.branchName);
}
/**
* A unique read-only string that changes whenever the resource is updated.
*
*/
@Export(name="etag", refs={String.class}, tree="[0]")
private Output etag;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public Output etag() {
return this.etag;
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The geo-location where the resource lives
*
*/
public Output location() {
return this.location;
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* The status of the Pull Request submitted against the users repository.
*
*/
@Export(name="prStatus", refs={String.class}, tree="[0]")
private Output prStatus;
/**
* @return The status of the Pull Request submitted against the users repository.
*
*/
public Output prStatus() {
return this.prStatus;
}
/**
* The number associated with the submitted pull request.
*
*/
@Export(name="pullNumber", refs={Integer.class}, tree="[0]")
private Output pullNumber;
/**
* @return The number associated with the submitted pull request.
*
*/
public Output pullNumber() {
return this.pullNumber;
}
/**
* Repository Main Branch
*
*/
@Export(name="repositoryMainBranch", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> repositoryMainBranch;
/**
* @return Repository Main Branch
*
*/
public Output> repositoryMainBranch() {
return Codegen.optional(this.repositoryMainBranch);
}
/**
* Repository Name
*
*/
@Export(name="repositoryName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> repositoryName;
/**
* @return Repository Name
*
*/
public Output> repositoryName() {
return Codegen.optional(this.repositoryName);
}
/**
* Repository Owner
*
*/
@Export(name="repositoryOwner", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> repositoryOwner;
/**
* @return Repository Owner
*
*/
public Output> repositoryOwner() {
return Codegen.optional(this.repositoryOwner);
}
@Export(name="stages", refs={List.class,StagePropertiesResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> stages;
public Output>> stages() {
return Codegen.optional(this.stages);
}
/**
* Terraform Storage Account Name
*
*/
@Export(name="storageAccountName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> storageAccountName;
/**
* @return Terraform Storage Account Name
*
*/
public Output> storageAccountName() {
return Codegen.optional(this.storageAccountName);
}
/**
* Terraform Storage Account Resource Group
*
*/
@Export(name="storageAccountResourceGroup", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> storageAccountResourceGroup;
/**
* @return Terraform Storage Account Resource Group
*
*/
public Output> storageAccountResourceGroup() {
return Codegen.optional(this.storageAccountResourceGroup);
}
/**
* Terraform Storage Account Subscription
*
*/
@Export(name="storageAccountSubscription", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> storageAccountSubscription;
/**
* @return Terraform Storage Account Subscription
*
*/
public Output> storageAccountSubscription() {
return Codegen.optional(this.storageAccountSubscription);
}
/**
* Terraform Container Name
*
*/
@Export(name="storageContainerName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> storageContainerName;
/**
* @return Terraform Container Name
*
*/
public Output> storageContainerName() {
return Codegen.optional(this.storageContainerName);
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
@Export(name="templates", refs={List.class,IacTemplatePropertiesResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> templates;
public Output>> templates() {
return Codegen.optional(this.templates);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public IacProfile(java.lang.String name) {
this(name, IacProfileArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public IacProfile(java.lang.String name, IacProfileArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public IacProfile(java.lang.String name, IacProfileArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:devhub:IacProfile", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private IacProfile(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:devhub:IacProfile", name, null, makeResourceOptions(options, id), false);
}
private static IacProfileArgs makeArgs(IacProfileArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? IacProfileArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:devhub/v20240501preview:IacProfile").build()),
Output.of(Alias.builder().type("azure-native:devhub/v20240801preview:IacProfile").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static IacProfile get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new IacProfile(name, id, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy