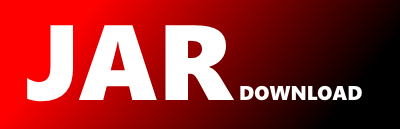
com.pulumi.azurenative.devhub.IacProfileArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.devhub;
import com.pulumi.azurenative.devhub.inputs.IacTemplatePropertiesArgs;
import com.pulumi.azurenative.devhub.inputs.StagePropertiesArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class IacProfileArgs extends com.pulumi.resources.ResourceArgs {
public static final IacProfileArgs Empty = new IacProfileArgs();
/**
* Repository Branch Name
*
*/
@Import(name="branchName")
private @Nullable Output branchName;
/**
* @return Repository Branch Name
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy