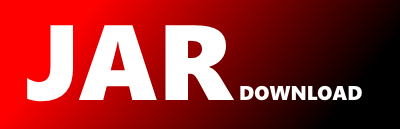
com.pulumi.azurenative.devhub.outputs.GetWorkflowResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.devhub.outputs;
import com.pulumi.azurenative.devhub.outputs.ACRResponse;
import com.pulumi.azurenative.devhub.outputs.DeploymentPropertiesResponse;
import com.pulumi.azurenative.devhub.outputs.GitHubWorkflowProfileResponseOidcCredentials;
import com.pulumi.azurenative.devhub.outputs.SystemDataResponse;
import com.pulumi.azurenative.devhub.outputs.WorkflowRunResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetWorkflowResult {
/**
* @return Information on the azure container registry
*
*/
private @Nullable ACRResponse acr;
/**
* @return The Azure Kubernetes Cluster Resource the application will be deployed to.
*
*/
private @Nullable String aksResourceId;
/**
* @return The name of the app.
*
*/
private @Nullable String appName;
/**
* @return Determines the authorization status of requests.
*
*/
private String authStatus;
/**
* @return Repository Branch Name
*
*/
private @Nullable String branchName;
/**
* @return The version of the language image used for building the code in the generated dockerfile.
*
*/
private @Nullable String builderVersion;
private @Nullable DeploymentPropertiesResponse deploymentProperties;
/**
* @return Path to Dockerfile Build Context within the repository.
*
*/
private @Nullable String dockerBuildContext;
/**
* @return Path to the Dockerfile within the repository.
*
*/
private @Nullable String dockerfile;
/**
* @return The mode of generation to be used for generating Dockerfiles.
*
*/
private @Nullable String dockerfileGenerationMode;
/**
* @return The directory to output the generated Dockerfile to.
*
*/
private @Nullable String dockerfileOutputDirectory;
/**
* @return The programming language used.
*
*/
private @Nullable String generationLanguage;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The name of the image to be generated.
*
*/
private @Nullable String imageName;
/**
* @return The tag to apply to the generated image.
*
*/
private @Nullable String imageTag;
/**
* @return The version of the language image used for execution in the generated dockerfile.
*
*/
private @Nullable String languageVersion;
private @Nullable WorkflowRunResponse lastWorkflowRun;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return The mode of generation to be used for generating Manifest.
*
*/
private @Nullable String manifestGenerationMode;
/**
* @return The directory to output the generated manifests to.
*
*/
private @Nullable String manifestOutputDirectory;
/**
* @return Determines the type of manifests to be generated.
*
*/
private @Nullable String manifestType;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Kubernetes namespace the application is deployed to.
*
*/
private @Nullable String namespace;
/**
* @return The fields needed for OIDC with GitHub.
*
*/
private @Nullable GitHubWorkflowProfileResponseOidcCredentials oidcCredentials;
/**
* @return The port the application is exposed on.
*
*/
private @Nullable String port;
/**
* @return The status of the Pull Request submitted against the users repository.
*
*/
private String prStatus;
/**
* @return The URL to the Pull Request submitted against the users repository.
*
*/
private String prURL;
/**
* @return The number associated with the submitted pull request.
*
*/
private Integer pullNumber;
/**
* @return Repository Name
*
*/
private @Nullable String repositoryName;
/**
* @return Repository Owner
*
*/
private @Nullable String repositoryOwner;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetWorkflowResult() {}
/**
* @return Information on the azure container registry
*
*/
public Optional acr() {
return Optional.ofNullable(this.acr);
}
/**
* @return The Azure Kubernetes Cluster Resource the application will be deployed to.
*
*/
public Optional aksResourceId() {
return Optional.ofNullable(this.aksResourceId);
}
/**
* @return The name of the app.
*
*/
public Optional appName() {
return Optional.ofNullable(this.appName);
}
/**
* @return Determines the authorization status of requests.
*
*/
public String authStatus() {
return this.authStatus;
}
/**
* @return Repository Branch Name
*
*/
public Optional branchName() {
return Optional.ofNullable(this.branchName);
}
/**
* @return The version of the language image used for building the code in the generated dockerfile.
*
*/
public Optional builderVersion() {
return Optional.ofNullable(this.builderVersion);
}
public Optional deploymentProperties() {
return Optional.ofNullable(this.deploymentProperties);
}
/**
* @return Path to Dockerfile Build Context within the repository.
*
*/
public Optional dockerBuildContext() {
return Optional.ofNullable(this.dockerBuildContext);
}
/**
* @return Path to the Dockerfile within the repository.
*
*/
public Optional dockerfile() {
return Optional.ofNullable(this.dockerfile);
}
/**
* @return The mode of generation to be used for generating Dockerfiles.
*
*/
public Optional dockerfileGenerationMode() {
return Optional.ofNullable(this.dockerfileGenerationMode);
}
/**
* @return The directory to output the generated Dockerfile to.
*
*/
public Optional dockerfileOutputDirectory() {
return Optional.ofNullable(this.dockerfileOutputDirectory);
}
/**
* @return The programming language used.
*
*/
public Optional generationLanguage() {
return Optional.ofNullable(this.generationLanguage);
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The name of the image to be generated.
*
*/
public Optional imageName() {
return Optional.ofNullable(this.imageName);
}
/**
* @return The tag to apply to the generated image.
*
*/
public Optional imageTag() {
return Optional.ofNullable(this.imageTag);
}
/**
* @return The version of the language image used for execution in the generated dockerfile.
*
*/
public Optional languageVersion() {
return Optional.ofNullable(this.languageVersion);
}
public Optional lastWorkflowRun() {
return Optional.ofNullable(this.lastWorkflowRun);
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return The mode of generation to be used for generating Manifest.
*
*/
public Optional manifestGenerationMode() {
return Optional.ofNullable(this.manifestGenerationMode);
}
/**
* @return The directory to output the generated manifests to.
*
*/
public Optional manifestOutputDirectory() {
return Optional.ofNullable(this.manifestOutputDirectory);
}
/**
* @return Determines the type of manifests to be generated.
*
*/
public Optional manifestType() {
return Optional.ofNullable(this.manifestType);
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Kubernetes namespace the application is deployed to.
*
*/
public Optional namespace() {
return Optional.ofNullable(this.namespace);
}
/**
* @return The fields needed for OIDC with GitHub.
*
*/
public Optional oidcCredentials() {
return Optional.ofNullable(this.oidcCredentials);
}
/**
* @return The port the application is exposed on.
*
*/
public Optional port() {
return Optional.ofNullable(this.port);
}
/**
* @return The status of the Pull Request submitted against the users repository.
*
*/
public String prStatus() {
return this.prStatus;
}
/**
* @return The URL to the Pull Request submitted against the users repository.
*
*/
public String prURL() {
return this.prURL;
}
/**
* @return The number associated with the submitted pull request.
*
*/
public Integer pullNumber() {
return this.pullNumber;
}
/**
* @return Repository Name
*
*/
public Optional repositoryName() {
return Optional.ofNullable(this.repositoryName);
}
/**
* @return Repository Owner
*
*/
public Optional repositoryOwner() {
return Optional.ofNullable(this.repositoryOwner);
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetWorkflowResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable ACRResponse acr;
private @Nullable String aksResourceId;
private @Nullable String appName;
private String authStatus;
private @Nullable String branchName;
private @Nullable String builderVersion;
private @Nullable DeploymentPropertiesResponse deploymentProperties;
private @Nullable String dockerBuildContext;
private @Nullable String dockerfile;
private @Nullable String dockerfileGenerationMode;
private @Nullable String dockerfileOutputDirectory;
private @Nullable String generationLanguage;
private String id;
private @Nullable String imageName;
private @Nullable String imageTag;
private @Nullable String languageVersion;
private @Nullable WorkflowRunResponse lastWorkflowRun;
private String location;
private @Nullable String manifestGenerationMode;
private @Nullable String manifestOutputDirectory;
private @Nullable String manifestType;
private String name;
private @Nullable String namespace;
private @Nullable GitHubWorkflowProfileResponseOidcCredentials oidcCredentials;
private @Nullable String port;
private String prStatus;
private String prURL;
private Integer pullNumber;
private @Nullable String repositoryName;
private @Nullable String repositoryOwner;
private SystemDataResponse systemData;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetWorkflowResult defaults) {
Objects.requireNonNull(defaults);
this.acr = defaults.acr;
this.aksResourceId = defaults.aksResourceId;
this.appName = defaults.appName;
this.authStatus = defaults.authStatus;
this.branchName = defaults.branchName;
this.builderVersion = defaults.builderVersion;
this.deploymentProperties = defaults.deploymentProperties;
this.dockerBuildContext = defaults.dockerBuildContext;
this.dockerfile = defaults.dockerfile;
this.dockerfileGenerationMode = defaults.dockerfileGenerationMode;
this.dockerfileOutputDirectory = defaults.dockerfileOutputDirectory;
this.generationLanguage = defaults.generationLanguage;
this.id = defaults.id;
this.imageName = defaults.imageName;
this.imageTag = defaults.imageTag;
this.languageVersion = defaults.languageVersion;
this.lastWorkflowRun = defaults.lastWorkflowRun;
this.location = defaults.location;
this.manifestGenerationMode = defaults.manifestGenerationMode;
this.manifestOutputDirectory = defaults.manifestOutputDirectory;
this.manifestType = defaults.manifestType;
this.name = defaults.name;
this.namespace = defaults.namespace;
this.oidcCredentials = defaults.oidcCredentials;
this.port = defaults.port;
this.prStatus = defaults.prStatus;
this.prURL = defaults.prURL;
this.pullNumber = defaults.pullNumber;
this.repositoryName = defaults.repositoryName;
this.repositoryOwner = defaults.repositoryOwner;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder acr(@Nullable ACRResponse acr) {
this.acr = acr;
return this;
}
@CustomType.Setter
public Builder aksResourceId(@Nullable String aksResourceId) {
this.aksResourceId = aksResourceId;
return this;
}
@CustomType.Setter
public Builder appName(@Nullable String appName) {
this.appName = appName;
return this;
}
@CustomType.Setter
public Builder authStatus(String authStatus) {
if (authStatus == null) {
throw new MissingRequiredPropertyException("GetWorkflowResult", "authStatus");
}
this.authStatus = authStatus;
return this;
}
@CustomType.Setter
public Builder branchName(@Nullable String branchName) {
this.branchName = branchName;
return this;
}
@CustomType.Setter
public Builder builderVersion(@Nullable String builderVersion) {
this.builderVersion = builderVersion;
return this;
}
@CustomType.Setter
public Builder deploymentProperties(@Nullable DeploymentPropertiesResponse deploymentProperties) {
this.deploymentProperties = deploymentProperties;
return this;
}
@CustomType.Setter
public Builder dockerBuildContext(@Nullable String dockerBuildContext) {
this.dockerBuildContext = dockerBuildContext;
return this;
}
@CustomType.Setter
public Builder dockerfile(@Nullable String dockerfile) {
this.dockerfile = dockerfile;
return this;
}
@CustomType.Setter
public Builder dockerfileGenerationMode(@Nullable String dockerfileGenerationMode) {
this.dockerfileGenerationMode = dockerfileGenerationMode;
return this;
}
@CustomType.Setter
public Builder dockerfileOutputDirectory(@Nullable String dockerfileOutputDirectory) {
this.dockerfileOutputDirectory = dockerfileOutputDirectory;
return this;
}
@CustomType.Setter
public Builder generationLanguage(@Nullable String generationLanguage) {
this.generationLanguage = generationLanguage;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetWorkflowResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder imageName(@Nullable String imageName) {
this.imageName = imageName;
return this;
}
@CustomType.Setter
public Builder imageTag(@Nullable String imageTag) {
this.imageTag = imageTag;
return this;
}
@CustomType.Setter
public Builder languageVersion(@Nullable String languageVersion) {
this.languageVersion = languageVersion;
return this;
}
@CustomType.Setter
public Builder lastWorkflowRun(@Nullable WorkflowRunResponse lastWorkflowRun) {
this.lastWorkflowRun = lastWorkflowRun;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetWorkflowResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder manifestGenerationMode(@Nullable String manifestGenerationMode) {
this.manifestGenerationMode = manifestGenerationMode;
return this;
}
@CustomType.Setter
public Builder manifestOutputDirectory(@Nullable String manifestOutputDirectory) {
this.manifestOutputDirectory = manifestOutputDirectory;
return this;
}
@CustomType.Setter
public Builder manifestType(@Nullable String manifestType) {
this.manifestType = manifestType;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetWorkflowResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder namespace(@Nullable String namespace) {
this.namespace = namespace;
return this;
}
@CustomType.Setter
public Builder oidcCredentials(@Nullable GitHubWorkflowProfileResponseOidcCredentials oidcCredentials) {
this.oidcCredentials = oidcCredentials;
return this;
}
@CustomType.Setter
public Builder port(@Nullable String port) {
this.port = port;
return this;
}
@CustomType.Setter
public Builder prStatus(String prStatus) {
if (prStatus == null) {
throw new MissingRequiredPropertyException("GetWorkflowResult", "prStatus");
}
this.prStatus = prStatus;
return this;
}
@CustomType.Setter
public Builder prURL(String prURL) {
if (prURL == null) {
throw new MissingRequiredPropertyException("GetWorkflowResult", "prURL");
}
this.prURL = prURL;
return this;
}
@CustomType.Setter
public Builder pullNumber(Integer pullNumber) {
if (pullNumber == null) {
throw new MissingRequiredPropertyException("GetWorkflowResult", "pullNumber");
}
this.pullNumber = pullNumber;
return this;
}
@CustomType.Setter
public Builder repositoryName(@Nullable String repositoryName) {
this.repositoryName = repositoryName;
return this;
}
@CustomType.Setter
public Builder repositoryOwner(@Nullable String repositoryOwner) {
this.repositoryOwner = repositoryOwner;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetWorkflowResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetWorkflowResult", "type");
}
this.type = type;
return this;
}
public GetWorkflowResult build() {
final var _resultValue = new GetWorkflowResult();
_resultValue.acr = acr;
_resultValue.aksResourceId = aksResourceId;
_resultValue.appName = appName;
_resultValue.authStatus = authStatus;
_resultValue.branchName = branchName;
_resultValue.builderVersion = builderVersion;
_resultValue.deploymentProperties = deploymentProperties;
_resultValue.dockerBuildContext = dockerBuildContext;
_resultValue.dockerfile = dockerfile;
_resultValue.dockerfileGenerationMode = dockerfileGenerationMode;
_resultValue.dockerfileOutputDirectory = dockerfileOutputDirectory;
_resultValue.generationLanguage = generationLanguage;
_resultValue.id = id;
_resultValue.imageName = imageName;
_resultValue.imageTag = imageTag;
_resultValue.languageVersion = languageVersion;
_resultValue.lastWorkflowRun = lastWorkflowRun;
_resultValue.location = location;
_resultValue.manifestGenerationMode = manifestGenerationMode;
_resultValue.manifestOutputDirectory = manifestOutputDirectory;
_resultValue.manifestType = manifestType;
_resultValue.name = name;
_resultValue.namespace = namespace;
_resultValue.oidcCredentials = oidcCredentials;
_resultValue.port = port;
_resultValue.prStatus = prStatus;
_resultValue.prURL = prURL;
_resultValue.pullNumber = pullNumber;
_resultValue.repositoryName = repositoryName;
_resultValue.repositoryOwner = repositoryOwner;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy