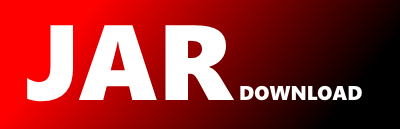
com.pulumi.azurenative.devices.DevicesFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.devices;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.devices.inputs.GetCertificateArgs;
import com.pulumi.azurenative.devices.inputs.GetCertificatePlainArgs;
import com.pulumi.azurenative.devices.inputs.GetDpsCertificateArgs;
import com.pulumi.azurenative.devices.inputs.GetDpsCertificatePlainArgs;
import com.pulumi.azurenative.devices.inputs.GetIotDpsResourceArgs;
import com.pulumi.azurenative.devices.inputs.GetIotDpsResourcePlainArgs;
import com.pulumi.azurenative.devices.inputs.GetIotDpsResourcePrivateEndpointConnectionArgs;
import com.pulumi.azurenative.devices.inputs.GetIotDpsResourcePrivateEndpointConnectionPlainArgs;
import com.pulumi.azurenative.devices.inputs.GetIotHubResourceArgs;
import com.pulumi.azurenative.devices.inputs.GetIotHubResourceEventHubConsumerGroupArgs;
import com.pulumi.azurenative.devices.inputs.GetIotHubResourceEventHubConsumerGroupPlainArgs;
import com.pulumi.azurenative.devices.inputs.GetIotHubResourcePlainArgs;
import com.pulumi.azurenative.devices.inputs.GetPrivateEndpointConnectionArgs;
import com.pulumi.azurenative.devices.inputs.GetPrivateEndpointConnectionPlainArgs;
import com.pulumi.azurenative.devices.inputs.ListIotDpsResourceKeysArgs;
import com.pulumi.azurenative.devices.inputs.ListIotDpsResourceKeysForKeyNameArgs;
import com.pulumi.azurenative.devices.inputs.ListIotDpsResourceKeysForKeyNamePlainArgs;
import com.pulumi.azurenative.devices.inputs.ListIotDpsResourceKeysPlainArgs;
import com.pulumi.azurenative.devices.inputs.ListIotHubResourceKeysArgs;
import com.pulumi.azurenative.devices.inputs.ListIotHubResourceKeysForKeyNameArgs;
import com.pulumi.azurenative.devices.inputs.ListIotHubResourceKeysForKeyNamePlainArgs;
import com.pulumi.azurenative.devices.inputs.ListIotHubResourceKeysPlainArgs;
import com.pulumi.azurenative.devices.outputs.GetCertificateResult;
import com.pulumi.azurenative.devices.outputs.GetDpsCertificateResult;
import com.pulumi.azurenative.devices.outputs.GetIotDpsResourcePrivateEndpointConnectionResult;
import com.pulumi.azurenative.devices.outputs.GetIotDpsResourceResult;
import com.pulumi.azurenative.devices.outputs.GetIotHubResourceEventHubConsumerGroupResult;
import com.pulumi.azurenative.devices.outputs.GetIotHubResourceResult;
import com.pulumi.azurenative.devices.outputs.GetPrivateEndpointConnectionResult;
import com.pulumi.azurenative.devices.outputs.ListIotDpsResourceKeysForKeyNameResult;
import com.pulumi.azurenative.devices.outputs.ListIotDpsResourceKeysResult;
import com.pulumi.azurenative.devices.outputs.ListIotHubResourceKeysForKeyNameResult;
import com.pulumi.azurenative.devices.outputs.ListIotHubResourceKeysResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class DevicesFunctions {
/**
* Returns the certificate.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2020-04-01, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static Output getCertificate(GetCertificateArgs args) {
return getCertificate(args, InvokeOptions.Empty);
}
/**
* Returns the certificate.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2020-04-01, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static CompletableFuture getCertificatePlain(GetCertificatePlainArgs args) {
return getCertificatePlain(args, InvokeOptions.Empty);
}
/**
* Returns the certificate.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2020-04-01, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static Output getCertificate(GetCertificateArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:devices:getCertificate", TypeShape.of(GetCertificateResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the certificate.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2020-04-01, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static CompletableFuture getCertificatePlain(GetCertificatePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:devices:getCertificate", TypeShape.of(GetCertificateResult.class), args, Utilities.withVersion(options));
}
/**
* Get the certificate from the provisioning service.
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2021-10-15, 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static Output getDpsCertificate(GetDpsCertificateArgs args) {
return getDpsCertificate(args, InvokeOptions.Empty);
}
/**
* Get the certificate from the provisioning service.
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2021-10-15, 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static CompletableFuture getDpsCertificatePlain(GetDpsCertificatePlainArgs args) {
return getDpsCertificatePlain(args, InvokeOptions.Empty);
}
/**
* Get the certificate from the provisioning service.
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2021-10-15, 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static Output getDpsCertificate(GetDpsCertificateArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:devices:getDpsCertificate", TypeShape.of(GetDpsCertificateResult.class), args, Utilities.withVersion(options));
}
/**
* Get the certificate from the provisioning service.
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2021-10-15, 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static CompletableFuture getDpsCertificatePlain(GetDpsCertificatePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:devices:getDpsCertificate", TypeShape.of(GetDpsCertificateResult.class), args, Utilities.withVersion(options));
}
/**
* Get the metadata of the provisioning service without SAS keys.
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2020-09-01-preview, 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static Output getIotDpsResource(GetIotDpsResourceArgs args) {
return getIotDpsResource(args, InvokeOptions.Empty);
}
/**
* Get the metadata of the provisioning service without SAS keys.
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2020-09-01-preview, 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static CompletableFuture getIotDpsResourcePlain(GetIotDpsResourcePlainArgs args) {
return getIotDpsResourcePlain(args, InvokeOptions.Empty);
}
/**
* Get the metadata of the provisioning service without SAS keys.
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2020-09-01-preview, 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static Output getIotDpsResource(GetIotDpsResourceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:devices:getIotDpsResource", TypeShape.of(GetIotDpsResourceResult.class), args, Utilities.withVersion(options));
}
/**
* Get the metadata of the provisioning service without SAS keys.
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2020-09-01-preview, 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static CompletableFuture getIotDpsResourcePlain(GetIotDpsResourcePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:devices:getIotDpsResource", TypeShape.of(GetIotDpsResourceResult.class), args, Utilities.withVersion(options));
}
/**
* Get private endpoint connection properties
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static Output getIotDpsResourcePrivateEndpointConnection(GetIotDpsResourcePrivateEndpointConnectionArgs args) {
return getIotDpsResourcePrivateEndpointConnection(args, InvokeOptions.Empty);
}
/**
* Get private endpoint connection properties
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static CompletableFuture getIotDpsResourcePrivateEndpointConnectionPlain(GetIotDpsResourcePrivateEndpointConnectionPlainArgs args) {
return getIotDpsResourcePrivateEndpointConnectionPlain(args, InvokeOptions.Empty);
}
/**
* Get private endpoint connection properties
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static Output getIotDpsResourcePrivateEndpointConnection(GetIotDpsResourcePrivateEndpointConnectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:devices:getIotDpsResourcePrivateEndpointConnection", TypeShape.of(GetIotDpsResourcePrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Get private endpoint connection properties
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static CompletableFuture getIotDpsResourcePrivateEndpointConnectionPlain(GetIotDpsResourcePrivateEndpointConnectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:devices:getIotDpsResourcePrivateEndpointConnection", TypeShape.of(GetIotDpsResourcePrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Get the non-security related metadata of an IoT hub.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2017-07-01, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static Output getIotHubResource(GetIotHubResourceArgs args) {
return getIotHubResource(args, InvokeOptions.Empty);
}
/**
* Get the non-security related metadata of an IoT hub.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2017-07-01, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static CompletableFuture getIotHubResourcePlain(GetIotHubResourcePlainArgs args) {
return getIotHubResourcePlain(args, InvokeOptions.Empty);
}
/**
* Get the non-security related metadata of an IoT hub.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2017-07-01, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static Output getIotHubResource(GetIotHubResourceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:devices:getIotHubResource", TypeShape.of(GetIotHubResourceResult.class), args, Utilities.withVersion(options));
}
/**
* Get the non-security related metadata of an IoT hub.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2017-07-01, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static CompletableFuture getIotHubResourcePlain(GetIotHubResourcePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:devices:getIotHubResource", TypeShape.of(GetIotHubResourceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a consumer group from the Event Hub-compatible device-to-cloud endpoint for an IoT hub.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2017-07-01, 2021-03-03-preview, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static Output getIotHubResourceEventHubConsumerGroup(GetIotHubResourceEventHubConsumerGroupArgs args) {
return getIotHubResourceEventHubConsumerGroup(args, InvokeOptions.Empty);
}
/**
* Get a consumer group from the Event Hub-compatible device-to-cloud endpoint for an IoT hub.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2017-07-01, 2021-03-03-preview, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static CompletableFuture getIotHubResourceEventHubConsumerGroupPlain(GetIotHubResourceEventHubConsumerGroupPlainArgs args) {
return getIotHubResourceEventHubConsumerGroupPlain(args, InvokeOptions.Empty);
}
/**
* Get a consumer group from the Event Hub-compatible device-to-cloud endpoint for an IoT hub.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2017-07-01, 2021-03-03-preview, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static Output getIotHubResourceEventHubConsumerGroup(GetIotHubResourceEventHubConsumerGroupArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:devices:getIotHubResourceEventHubConsumerGroup", TypeShape.of(GetIotHubResourceEventHubConsumerGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Get a consumer group from the Event Hub-compatible device-to-cloud endpoint for an IoT hub.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2017-07-01, 2021-03-03-preview, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static CompletableFuture getIotHubResourceEventHubConsumerGroupPlain(GetIotHubResourceEventHubConsumerGroupPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:devices:getIotHubResourceEventHubConsumerGroup", TypeShape.of(GetIotHubResourceEventHubConsumerGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Get private endpoint connection properties
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args) {
return getPrivateEndpointConnection(args, InvokeOptions.Empty);
}
/**
* Get private endpoint connection properties
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args) {
return getPrivateEndpointConnectionPlain(args, InvokeOptions.Empty);
}
/**
* Get private endpoint connection properties
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:devices:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Get private endpoint connection properties
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:devices:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* List the primary and secondary keys for a provisioning service.
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2020-09-01-preview, 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static Output listIotDpsResourceKeys(ListIotDpsResourceKeysArgs args) {
return listIotDpsResourceKeys(args, InvokeOptions.Empty);
}
/**
* List the primary and secondary keys for a provisioning service.
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2020-09-01-preview, 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static CompletableFuture listIotDpsResourceKeysPlain(ListIotDpsResourceKeysPlainArgs args) {
return listIotDpsResourceKeysPlain(args, InvokeOptions.Empty);
}
/**
* List the primary and secondary keys for a provisioning service.
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2020-09-01-preview, 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static Output listIotDpsResourceKeys(ListIotDpsResourceKeysArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:devices:listIotDpsResourceKeys", TypeShape.of(ListIotDpsResourceKeysResult.class), args, Utilities.withVersion(options));
}
/**
* List the primary and secondary keys for a provisioning service.
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2020-09-01-preview, 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static CompletableFuture listIotDpsResourceKeysPlain(ListIotDpsResourceKeysPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:devices:listIotDpsResourceKeys", TypeShape.of(ListIotDpsResourceKeysResult.class), args, Utilities.withVersion(options));
}
/**
* List primary and secondary keys for a specific key name
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2020-09-01-preview, 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static Output listIotDpsResourceKeysForKeyName(ListIotDpsResourceKeysForKeyNameArgs args) {
return listIotDpsResourceKeysForKeyName(args, InvokeOptions.Empty);
}
/**
* List primary and secondary keys for a specific key name
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2020-09-01-preview, 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static CompletableFuture listIotDpsResourceKeysForKeyNamePlain(ListIotDpsResourceKeysForKeyNamePlainArgs args) {
return listIotDpsResourceKeysForKeyNamePlain(args, InvokeOptions.Empty);
}
/**
* List primary and secondary keys for a specific key name
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2020-09-01-preview, 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static Output listIotDpsResourceKeysForKeyName(ListIotDpsResourceKeysForKeyNameArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:devices:listIotDpsResourceKeysForKeyName", TypeShape.of(ListIotDpsResourceKeysForKeyNameResult.class), args, Utilities.withVersion(options));
}
/**
* List primary and secondary keys for a specific key name
* Azure REST API version: 2022-12-12.
*
* Other available API versions: 2020-09-01-preview, 2023-03-01-preview, 2025-02-01-preview.
*
*/
public static CompletableFuture listIotDpsResourceKeysForKeyNamePlain(ListIotDpsResourceKeysForKeyNamePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:devices:listIotDpsResourceKeysForKeyName", TypeShape.of(ListIotDpsResourceKeysForKeyNameResult.class), args, Utilities.withVersion(options));
}
/**
* Get the security metadata for an IoT hub. For more information, see: https://docs.microsoft.com/azure/iot-hub/iot-hub-devguide-security.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2017-07-01, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static Output listIotHubResourceKeys(ListIotHubResourceKeysArgs args) {
return listIotHubResourceKeys(args, InvokeOptions.Empty);
}
/**
* Get the security metadata for an IoT hub. For more information, see: https://docs.microsoft.com/azure/iot-hub/iot-hub-devguide-security.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2017-07-01, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static CompletableFuture listIotHubResourceKeysPlain(ListIotHubResourceKeysPlainArgs args) {
return listIotHubResourceKeysPlain(args, InvokeOptions.Empty);
}
/**
* Get the security metadata for an IoT hub. For more information, see: https://docs.microsoft.com/azure/iot-hub/iot-hub-devguide-security.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2017-07-01, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static Output listIotHubResourceKeys(ListIotHubResourceKeysArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:devices:listIotHubResourceKeys", TypeShape.of(ListIotHubResourceKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Get the security metadata for an IoT hub. For more information, see: https://docs.microsoft.com/azure/iot-hub/iot-hub-devguide-security.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2017-07-01, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static CompletableFuture listIotHubResourceKeysPlain(ListIotHubResourceKeysPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:devices:listIotHubResourceKeys", TypeShape.of(ListIotHubResourceKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Get a shared access policy by name from an IoT hub. For more information, see: https://docs.microsoft.com/azure/iot-hub/iot-hub-devguide-security.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2017-07-01, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static Output listIotHubResourceKeysForKeyName(ListIotHubResourceKeysForKeyNameArgs args) {
return listIotHubResourceKeysForKeyName(args, InvokeOptions.Empty);
}
/**
* Get a shared access policy by name from an IoT hub. For more information, see: https://docs.microsoft.com/azure/iot-hub/iot-hub-devguide-security.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2017-07-01, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static CompletableFuture listIotHubResourceKeysForKeyNamePlain(ListIotHubResourceKeysForKeyNamePlainArgs args) {
return listIotHubResourceKeysForKeyNamePlain(args, InvokeOptions.Empty);
}
/**
* Get a shared access policy by name from an IoT hub. For more information, see: https://docs.microsoft.com/azure/iot-hub/iot-hub-devguide-security.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2017-07-01, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static Output listIotHubResourceKeysForKeyName(ListIotHubResourceKeysForKeyNameArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:devices:listIotHubResourceKeysForKeyName", TypeShape.of(ListIotHubResourceKeysForKeyNameResult.class), args, Utilities.withVersion(options));
}
/**
* Get a shared access policy by name from an IoT hub. For more information, see: https://docs.microsoft.com/azure/iot-hub/iot-hub-devguide-security.
* Azure REST API version: 2022-04-30-preview.
*
* Other available API versions: 2017-07-01, 2022-11-15-preview, 2023-06-30, 2023-06-30-preview.
*
*/
public static CompletableFuture listIotHubResourceKeysForKeyNamePlain(ListIotHubResourceKeysForKeyNamePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:devices:listIotHubResourceKeysForKeyName", TypeShape.of(ListIotHubResourceKeysForKeyNameResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy