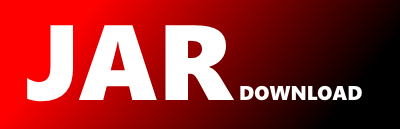
com.pulumi.azurenative.devices.outputs.IotHubPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.devices.outputs;
import com.pulumi.azurenative.devices.outputs.CloudToDevicePropertiesResponse;
import com.pulumi.azurenative.devices.outputs.EncryptionPropertiesDescriptionResponse;
import com.pulumi.azurenative.devices.outputs.EventHubPropertiesResponse;
import com.pulumi.azurenative.devices.outputs.IotHubLocationDescriptionResponse;
import com.pulumi.azurenative.devices.outputs.IotHubPropertiesResponseDeviceStreams;
import com.pulumi.azurenative.devices.outputs.IpFilterRuleResponse;
import com.pulumi.azurenative.devices.outputs.MessagingEndpointPropertiesResponse;
import com.pulumi.azurenative.devices.outputs.NetworkRuleSetPropertiesResponse;
import com.pulumi.azurenative.devices.outputs.PrivateEndpointConnectionResponse;
import com.pulumi.azurenative.devices.outputs.RootCertificatePropertiesResponse;
import com.pulumi.azurenative.devices.outputs.RoutingPropertiesResponse;
import com.pulumi.azurenative.devices.outputs.SharedAccessSignatureAuthorizationRuleResponse;
import com.pulumi.azurenative.devices.outputs.StorageEndpointPropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class IotHubPropertiesResponse {
/**
* @return List of allowed FQDNs(Fully Qualified Domain Name) for egress from Iot Hub.
*
*/
private @Nullable List allowedFqdnList;
/**
* @return The shared access policies you can use to secure a connection to the IoT hub.
*
*/
private @Nullable List authorizationPolicies;
/**
* @return The IoT hub cloud-to-device messaging properties.
*
*/
private @Nullable CloudToDevicePropertiesResponse cloudToDevice;
/**
* @return IoT hub comments.
*
*/
private @Nullable String comments;
/**
* @return The device streams properties of iothub.
*
*/
private @Nullable IotHubPropertiesResponseDeviceStreams deviceStreams;
/**
* @return If true, all device(including Edge devices but excluding modules) scoped SAS keys cannot be used for authentication.
*
*/
private @Nullable Boolean disableDeviceSAS;
/**
* @return If true, SAS tokens with Iot hub scoped SAS keys cannot be used for authentication.
*
*/
private @Nullable Boolean disableLocalAuth;
/**
* @return If true, all module scoped SAS keys cannot be used for authentication.
*
*/
private @Nullable Boolean disableModuleSAS;
/**
* @return This property when set to true, will enable data residency, thus, disabling disaster recovery.
*
*/
private @Nullable Boolean enableDataResidency;
/**
* @return If True, file upload notifications are enabled.
*
*/
private @Nullable Boolean enableFileUploadNotifications;
/**
* @return The encryption properties for the IoT hub.
*
*/
private @Nullable EncryptionPropertiesDescriptionResponse encryption;
/**
* @return The Event Hub-compatible endpoint properties. The only possible keys to this dictionary is events. This key has to be present in the dictionary while making create or update calls for the IoT hub.
*
*/
private @Nullable Map eventHubEndpoints;
/**
* @return The capabilities and features enabled for the IoT hub.
*
*/
private @Nullable String features;
/**
* @return The name of the host.
*
*/
private String hostName;
/**
* @return The IP filter rules.
*
*/
private @Nullable List ipFilterRules;
/**
* @return Primary and secondary location for iot hub
*
*/
private List locations;
/**
* @return The messaging endpoint properties for the file upload notification queue.
*
*/
private @Nullable Map messagingEndpoints;
/**
* @return Specifies the minimum TLS version to support for this hub. Can be set to "1.2" to have clients that use a TLS version below 1.2 to be rejected.
*
*/
private @Nullable String minTlsVersion;
/**
* @return Network Rule Set Properties of IotHub
*
*/
private @Nullable NetworkRuleSetPropertiesResponse networkRuleSets;
/**
* @return Private endpoint connections created on this IotHub
*
*/
private @Nullable List privateEndpointConnections;
/**
* @return The provisioning state.
*
*/
private String provisioningState;
/**
* @return Whether requests from Public Network are allowed
*
*/
private @Nullable String publicNetworkAccess;
/**
* @return If true, egress from IotHub will be restricted to only the allowed FQDNs that are configured via allowedFqdnList.
*
*/
private @Nullable Boolean restrictOutboundNetworkAccess;
/**
* @return This property store root certificate related information
*
*/
private @Nullable RootCertificatePropertiesResponse rootCertificate;
/**
* @return The routing related properties of the IoT hub. See: https://docs.microsoft.com/azure/iot-hub/iot-hub-devguide-messaging
*
*/
private @Nullable RoutingPropertiesResponse routing;
/**
* @return The hub state.
*
*/
private String state;
/**
* @return The list of Azure Storage endpoints where you can upload files. Currently you can configure only one Azure Storage account and that MUST have its key as $default. Specifying more than one storage account causes an error to be thrown. Not specifying a value for this property when the enableFileUploadNotifications property is set to True, causes an error to be thrown.
*
*/
private @Nullable Map storageEndpoints;
private IotHubPropertiesResponse() {}
/**
* @return List of allowed FQDNs(Fully Qualified Domain Name) for egress from Iot Hub.
*
*/
public List allowedFqdnList() {
return this.allowedFqdnList == null ? List.of() : this.allowedFqdnList;
}
/**
* @return The shared access policies you can use to secure a connection to the IoT hub.
*
*/
public List authorizationPolicies() {
return this.authorizationPolicies == null ? List.of() : this.authorizationPolicies;
}
/**
* @return The IoT hub cloud-to-device messaging properties.
*
*/
public Optional cloudToDevice() {
return Optional.ofNullable(this.cloudToDevice);
}
/**
* @return IoT hub comments.
*
*/
public Optional comments() {
return Optional.ofNullable(this.comments);
}
/**
* @return The device streams properties of iothub.
*
*/
public Optional deviceStreams() {
return Optional.ofNullable(this.deviceStreams);
}
/**
* @return If true, all device(including Edge devices but excluding modules) scoped SAS keys cannot be used for authentication.
*
*/
public Optional disableDeviceSAS() {
return Optional.ofNullable(this.disableDeviceSAS);
}
/**
* @return If true, SAS tokens with Iot hub scoped SAS keys cannot be used for authentication.
*
*/
public Optional disableLocalAuth() {
return Optional.ofNullable(this.disableLocalAuth);
}
/**
* @return If true, all module scoped SAS keys cannot be used for authentication.
*
*/
public Optional disableModuleSAS() {
return Optional.ofNullable(this.disableModuleSAS);
}
/**
* @return This property when set to true, will enable data residency, thus, disabling disaster recovery.
*
*/
public Optional enableDataResidency() {
return Optional.ofNullable(this.enableDataResidency);
}
/**
* @return If True, file upload notifications are enabled.
*
*/
public Optional enableFileUploadNotifications() {
return Optional.ofNullable(this.enableFileUploadNotifications);
}
/**
* @return The encryption properties for the IoT hub.
*
*/
public Optional encryption() {
return Optional.ofNullable(this.encryption);
}
/**
* @return The Event Hub-compatible endpoint properties. The only possible keys to this dictionary is events. This key has to be present in the dictionary while making create or update calls for the IoT hub.
*
*/
public Map eventHubEndpoints() {
return this.eventHubEndpoints == null ? Map.of() : this.eventHubEndpoints;
}
/**
* @return The capabilities and features enabled for the IoT hub.
*
*/
public Optional features() {
return Optional.ofNullable(this.features);
}
/**
* @return The name of the host.
*
*/
public String hostName() {
return this.hostName;
}
/**
* @return The IP filter rules.
*
*/
public List ipFilterRules() {
return this.ipFilterRules == null ? List.of() : this.ipFilterRules;
}
/**
* @return Primary and secondary location for iot hub
*
*/
public List locations() {
return this.locations;
}
/**
* @return The messaging endpoint properties for the file upload notification queue.
*
*/
public Map messagingEndpoints() {
return this.messagingEndpoints == null ? Map.of() : this.messagingEndpoints;
}
/**
* @return Specifies the minimum TLS version to support for this hub. Can be set to "1.2" to have clients that use a TLS version below 1.2 to be rejected.
*
*/
public Optional minTlsVersion() {
return Optional.ofNullable(this.minTlsVersion);
}
/**
* @return Network Rule Set Properties of IotHub
*
*/
public Optional networkRuleSets() {
return Optional.ofNullable(this.networkRuleSets);
}
/**
* @return Private endpoint connections created on this IotHub
*
*/
public List privateEndpointConnections() {
return this.privateEndpointConnections == null ? List.of() : this.privateEndpointConnections;
}
/**
* @return The provisioning state.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Whether requests from Public Network are allowed
*
*/
public Optional publicNetworkAccess() {
return Optional.ofNullable(this.publicNetworkAccess);
}
/**
* @return If true, egress from IotHub will be restricted to only the allowed FQDNs that are configured via allowedFqdnList.
*
*/
public Optional restrictOutboundNetworkAccess() {
return Optional.ofNullable(this.restrictOutboundNetworkAccess);
}
/**
* @return This property store root certificate related information
*
*/
public Optional rootCertificate() {
return Optional.ofNullable(this.rootCertificate);
}
/**
* @return The routing related properties of the IoT hub. See: https://docs.microsoft.com/azure/iot-hub/iot-hub-devguide-messaging
*
*/
public Optional routing() {
return Optional.ofNullable(this.routing);
}
/**
* @return The hub state.
*
*/
public String state() {
return this.state;
}
/**
* @return The list of Azure Storage endpoints where you can upload files. Currently you can configure only one Azure Storage account and that MUST have its key as $default. Specifying more than one storage account causes an error to be thrown. Not specifying a value for this property when the enableFileUploadNotifications property is set to True, causes an error to be thrown.
*
*/
public Map storageEndpoints() {
return this.storageEndpoints == null ? Map.of() : this.storageEndpoints;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(IotHubPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List allowedFqdnList;
private @Nullable List authorizationPolicies;
private @Nullable CloudToDevicePropertiesResponse cloudToDevice;
private @Nullable String comments;
private @Nullable IotHubPropertiesResponseDeviceStreams deviceStreams;
private @Nullable Boolean disableDeviceSAS;
private @Nullable Boolean disableLocalAuth;
private @Nullable Boolean disableModuleSAS;
private @Nullable Boolean enableDataResidency;
private @Nullable Boolean enableFileUploadNotifications;
private @Nullable EncryptionPropertiesDescriptionResponse encryption;
private @Nullable Map eventHubEndpoints;
private @Nullable String features;
private String hostName;
private @Nullable List ipFilterRules;
private List locations;
private @Nullable Map messagingEndpoints;
private @Nullable String minTlsVersion;
private @Nullable NetworkRuleSetPropertiesResponse networkRuleSets;
private @Nullable List privateEndpointConnections;
private String provisioningState;
private @Nullable String publicNetworkAccess;
private @Nullable Boolean restrictOutboundNetworkAccess;
private @Nullable RootCertificatePropertiesResponse rootCertificate;
private @Nullable RoutingPropertiesResponse routing;
private String state;
private @Nullable Map storageEndpoints;
public Builder() {}
public Builder(IotHubPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.allowedFqdnList = defaults.allowedFqdnList;
this.authorizationPolicies = defaults.authorizationPolicies;
this.cloudToDevice = defaults.cloudToDevice;
this.comments = defaults.comments;
this.deviceStreams = defaults.deviceStreams;
this.disableDeviceSAS = defaults.disableDeviceSAS;
this.disableLocalAuth = defaults.disableLocalAuth;
this.disableModuleSAS = defaults.disableModuleSAS;
this.enableDataResidency = defaults.enableDataResidency;
this.enableFileUploadNotifications = defaults.enableFileUploadNotifications;
this.encryption = defaults.encryption;
this.eventHubEndpoints = defaults.eventHubEndpoints;
this.features = defaults.features;
this.hostName = defaults.hostName;
this.ipFilterRules = defaults.ipFilterRules;
this.locations = defaults.locations;
this.messagingEndpoints = defaults.messagingEndpoints;
this.minTlsVersion = defaults.minTlsVersion;
this.networkRuleSets = defaults.networkRuleSets;
this.privateEndpointConnections = defaults.privateEndpointConnections;
this.provisioningState = defaults.provisioningState;
this.publicNetworkAccess = defaults.publicNetworkAccess;
this.restrictOutboundNetworkAccess = defaults.restrictOutboundNetworkAccess;
this.rootCertificate = defaults.rootCertificate;
this.routing = defaults.routing;
this.state = defaults.state;
this.storageEndpoints = defaults.storageEndpoints;
}
@CustomType.Setter
public Builder allowedFqdnList(@Nullable List allowedFqdnList) {
this.allowedFqdnList = allowedFqdnList;
return this;
}
public Builder allowedFqdnList(String... allowedFqdnList) {
return allowedFqdnList(List.of(allowedFqdnList));
}
@CustomType.Setter
public Builder authorizationPolicies(@Nullable List authorizationPolicies) {
this.authorizationPolicies = authorizationPolicies;
return this;
}
public Builder authorizationPolicies(SharedAccessSignatureAuthorizationRuleResponse... authorizationPolicies) {
return authorizationPolicies(List.of(authorizationPolicies));
}
@CustomType.Setter
public Builder cloudToDevice(@Nullable CloudToDevicePropertiesResponse cloudToDevice) {
this.cloudToDevice = cloudToDevice;
return this;
}
@CustomType.Setter
public Builder comments(@Nullable String comments) {
this.comments = comments;
return this;
}
@CustomType.Setter
public Builder deviceStreams(@Nullable IotHubPropertiesResponseDeviceStreams deviceStreams) {
this.deviceStreams = deviceStreams;
return this;
}
@CustomType.Setter
public Builder disableDeviceSAS(@Nullable Boolean disableDeviceSAS) {
this.disableDeviceSAS = disableDeviceSAS;
return this;
}
@CustomType.Setter
public Builder disableLocalAuth(@Nullable Boolean disableLocalAuth) {
this.disableLocalAuth = disableLocalAuth;
return this;
}
@CustomType.Setter
public Builder disableModuleSAS(@Nullable Boolean disableModuleSAS) {
this.disableModuleSAS = disableModuleSAS;
return this;
}
@CustomType.Setter
public Builder enableDataResidency(@Nullable Boolean enableDataResidency) {
this.enableDataResidency = enableDataResidency;
return this;
}
@CustomType.Setter
public Builder enableFileUploadNotifications(@Nullable Boolean enableFileUploadNotifications) {
this.enableFileUploadNotifications = enableFileUploadNotifications;
return this;
}
@CustomType.Setter
public Builder encryption(@Nullable EncryptionPropertiesDescriptionResponse encryption) {
this.encryption = encryption;
return this;
}
@CustomType.Setter
public Builder eventHubEndpoints(@Nullable Map eventHubEndpoints) {
this.eventHubEndpoints = eventHubEndpoints;
return this;
}
@CustomType.Setter
public Builder features(@Nullable String features) {
this.features = features;
return this;
}
@CustomType.Setter
public Builder hostName(String hostName) {
if (hostName == null) {
throw new MissingRequiredPropertyException("IotHubPropertiesResponse", "hostName");
}
this.hostName = hostName;
return this;
}
@CustomType.Setter
public Builder ipFilterRules(@Nullable List ipFilterRules) {
this.ipFilterRules = ipFilterRules;
return this;
}
public Builder ipFilterRules(IpFilterRuleResponse... ipFilterRules) {
return ipFilterRules(List.of(ipFilterRules));
}
@CustomType.Setter
public Builder locations(List locations) {
if (locations == null) {
throw new MissingRequiredPropertyException("IotHubPropertiesResponse", "locations");
}
this.locations = locations;
return this;
}
public Builder locations(IotHubLocationDescriptionResponse... locations) {
return locations(List.of(locations));
}
@CustomType.Setter
public Builder messagingEndpoints(@Nullable Map messagingEndpoints) {
this.messagingEndpoints = messagingEndpoints;
return this;
}
@CustomType.Setter
public Builder minTlsVersion(@Nullable String minTlsVersion) {
this.minTlsVersion = minTlsVersion;
return this;
}
@CustomType.Setter
public Builder networkRuleSets(@Nullable NetworkRuleSetPropertiesResponse networkRuleSets) {
this.networkRuleSets = networkRuleSets;
return this;
}
@CustomType.Setter
public Builder privateEndpointConnections(@Nullable List privateEndpointConnections) {
this.privateEndpointConnections = privateEndpointConnections;
return this;
}
public Builder privateEndpointConnections(PrivateEndpointConnectionResponse... privateEndpointConnections) {
return privateEndpointConnections(List.of(privateEndpointConnections));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("IotHubPropertiesResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder publicNetworkAccess(@Nullable String publicNetworkAccess) {
this.publicNetworkAccess = publicNetworkAccess;
return this;
}
@CustomType.Setter
public Builder restrictOutboundNetworkAccess(@Nullable Boolean restrictOutboundNetworkAccess) {
this.restrictOutboundNetworkAccess = restrictOutboundNetworkAccess;
return this;
}
@CustomType.Setter
public Builder rootCertificate(@Nullable RootCertificatePropertiesResponse rootCertificate) {
this.rootCertificate = rootCertificate;
return this;
}
@CustomType.Setter
public Builder routing(@Nullable RoutingPropertiesResponse routing) {
this.routing = routing;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("IotHubPropertiesResponse", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder storageEndpoints(@Nullable Map storageEndpoints) {
this.storageEndpoints = storageEndpoints;
return this;
}
public IotHubPropertiesResponse build() {
final var _resultValue = new IotHubPropertiesResponse();
_resultValue.allowedFqdnList = allowedFqdnList;
_resultValue.authorizationPolicies = authorizationPolicies;
_resultValue.cloudToDevice = cloudToDevice;
_resultValue.comments = comments;
_resultValue.deviceStreams = deviceStreams;
_resultValue.disableDeviceSAS = disableDeviceSAS;
_resultValue.disableLocalAuth = disableLocalAuth;
_resultValue.disableModuleSAS = disableModuleSAS;
_resultValue.enableDataResidency = enableDataResidency;
_resultValue.enableFileUploadNotifications = enableFileUploadNotifications;
_resultValue.encryption = encryption;
_resultValue.eventHubEndpoints = eventHubEndpoints;
_resultValue.features = features;
_resultValue.hostName = hostName;
_resultValue.ipFilterRules = ipFilterRules;
_resultValue.locations = locations;
_resultValue.messagingEndpoints = messagingEndpoints;
_resultValue.minTlsVersion = minTlsVersion;
_resultValue.networkRuleSets = networkRuleSets;
_resultValue.privateEndpointConnections = privateEndpointConnections;
_resultValue.provisioningState = provisioningState;
_resultValue.publicNetworkAccess = publicNetworkAccess;
_resultValue.restrictOutboundNetworkAccess = restrictOutboundNetworkAccess;
_resultValue.rootCertificate = rootCertificate;
_resultValue.routing = routing;
_resultValue.state = state;
_resultValue.storageEndpoints = storageEndpoints;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy