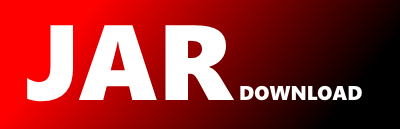
com.pulumi.azurenative.devtestlab.VirtualMachine Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.devtestlab;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.devtestlab.VirtualMachineArgs;
import com.pulumi.azurenative.devtestlab.outputs.ApplicableScheduleResponse;
import com.pulumi.azurenative.devtestlab.outputs.ArtifactDeploymentStatusPropertiesResponse;
import com.pulumi.azurenative.devtestlab.outputs.ArtifactInstallPropertiesResponse;
import com.pulumi.azurenative.devtestlab.outputs.ComputeVmPropertiesResponse;
import com.pulumi.azurenative.devtestlab.outputs.DataDiskPropertiesResponse;
import com.pulumi.azurenative.devtestlab.outputs.GalleryImageReferenceResponse;
import com.pulumi.azurenative.devtestlab.outputs.NetworkInterfacePropertiesResponse;
import com.pulumi.azurenative.devtestlab.outputs.ScheduleCreationParameterResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A virtual machine.
* Azure REST API version: 2018-09-15. Prior API version in Azure Native 1.x: 2018-09-15.
*
* Other available API versions: 2016-05-15.
*
* ## Example Usage
* ### VirtualMachines_CreateOrUpdate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.devtestlab.VirtualMachine;
* import com.pulumi.azurenative.devtestlab.VirtualMachineArgs;
* import com.pulumi.azurenative.devtestlab.inputs.GalleryImageReferenceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var virtualMachine = new VirtualMachine("virtualMachine", VirtualMachineArgs.builder()
* .allowClaim(true)
* .disallowPublicIpAddress(true)
* .galleryImageReference(GalleryImageReferenceArgs.builder()
* .offer("UbuntuServer")
* .osType("Linux")
* .publisher("Canonical")
* .sku("16.04-LTS")
* .version("Latest")
* .build())
* .labName("{labName}")
* .labSubnetName("{virtualNetworkName}Subnet")
* .labVirtualNetworkId("/subscriptions/{subscriptionId}/resourcegroups/resourceGroupName/providers/microsoft.devtestlab/labs/{labName}/virtualnetworks/{virtualNetworkName}")
* .location("{location}")
* .name("{vmName}")
* .password("{userPassword}")
* .resourceGroupName("resourceGroupName")
* .size("Standard_A2_v2")
* .storageType("Standard")
* .tags(Map.of("tagName1", "tagValue1"))
* .userName("{userName}")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:devtestlab:VirtualMachine {vmName} /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DevTestLab/labs/{labName}/virtualmachines/{name}
* ```
*
*/
@ResourceType(type="azure-native:devtestlab:VirtualMachine")
public class VirtualMachine extends com.pulumi.resources.CustomResource {
/**
* Indicates whether another user can take ownership of the virtual machine
*
*/
@Export(name="allowClaim", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> allowClaim;
/**
* @return Indicates whether another user can take ownership of the virtual machine
*
*/
public Output> allowClaim() {
return Codegen.optional(this.allowClaim);
}
/**
* The applicable schedule for the virtual machine.
*
*/
@Export(name="applicableSchedule", refs={ApplicableScheduleResponse.class}, tree="[0]")
private Output applicableSchedule;
/**
* @return The applicable schedule for the virtual machine.
*
*/
public Output applicableSchedule() {
return this.applicableSchedule;
}
/**
* The artifact deployment status for the virtual machine.
*
*/
@Export(name="artifactDeploymentStatus", refs={ArtifactDeploymentStatusPropertiesResponse.class}, tree="[0]")
private Output artifactDeploymentStatus;
/**
* @return The artifact deployment status for the virtual machine.
*
*/
public Output artifactDeploymentStatus() {
return this.artifactDeploymentStatus;
}
/**
* The artifacts to be installed on the virtual machine.
*
*/
@Export(name="artifacts", refs={List.class,ArtifactInstallPropertiesResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> artifacts;
/**
* @return The artifacts to be installed on the virtual machine.
*
*/
public Output>> artifacts() {
return Codegen.optional(this.artifacts);
}
/**
* The resource identifier (Microsoft.Compute) of the virtual machine.
*
*/
@Export(name="computeId", refs={String.class}, tree="[0]")
private Output computeId;
/**
* @return The resource identifier (Microsoft.Compute) of the virtual machine.
*
*/
public Output computeId() {
return this.computeId;
}
/**
* The compute virtual machine properties.
*
*/
@Export(name="computeVm", refs={ComputeVmPropertiesResponse.class}, tree="[0]")
private Output computeVm;
/**
* @return The compute virtual machine properties.
*
*/
public Output computeVm() {
return this.computeVm;
}
/**
* The email address of creator of the virtual machine.
*
*/
@Export(name="createdByUser", refs={String.class}, tree="[0]")
private Output createdByUser;
/**
* @return The email address of creator of the virtual machine.
*
*/
public Output createdByUser() {
return this.createdByUser;
}
/**
* The object identifier of the creator of the virtual machine.
*
*/
@Export(name="createdByUserId", refs={String.class}, tree="[0]")
private Output createdByUserId;
/**
* @return The object identifier of the creator of the virtual machine.
*
*/
public Output createdByUserId() {
return this.createdByUserId;
}
/**
* The creation date of the virtual machine.
*
*/
@Export(name="createdDate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> createdDate;
/**
* @return The creation date of the virtual machine.
*
*/
public Output> createdDate() {
return Codegen.optional(this.createdDate);
}
/**
* The custom image identifier of the virtual machine.
*
*/
@Export(name="customImageId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> customImageId;
/**
* @return The custom image identifier of the virtual machine.
*
*/
public Output> customImageId() {
return Codegen.optional(this.customImageId);
}
/**
* New or existing data disks to attach to the virtual machine after creation
*
*/
@Export(name="dataDiskParameters", refs={List.class,DataDiskPropertiesResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> dataDiskParameters;
/**
* @return New or existing data disks to attach to the virtual machine after creation
*
*/
public Output>> dataDiskParameters() {
return Codegen.optional(this.dataDiskParameters);
}
/**
* Indicates whether the virtual machine is to be created without a public IP address.
*
*/
@Export(name="disallowPublicIpAddress", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> disallowPublicIpAddress;
/**
* @return Indicates whether the virtual machine is to be created without a public IP address.
*
*/
public Output> disallowPublicIpAddress() {
return Codegen.optional(this.disallowPublicIpAddress);
}
/**
* The resource ID of the environment that contains this virtual machine, if any.
*
*/
@Export(name="environmentId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> environmentId;
/**
* @return The resource ID of the environment that contains this virtual machine, if any.
*
*/
public Output> environmentId() {
return Codegen.optional(this.environmentId);
}
/**
* The expiration date for VM.
*
*/
@Export(name="expirationDate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> expirationDate;
/**
* @return The expiration date for VM.
*
*/
public Output> expirationDate() {
return Codegen.optional(this.expirationDate);
}
/**
* The fully-qualified domain name of the virtual machine.
*
*/
@Export(name="fqdn", refs={String.class}, tree="[0]")
private Output fqdn;
/**
* @return The fully-qualified domain name of the virtual machine.
*
*/
public Output fqdn() {
return this.fqdn;
}
/**
* The Microsoft Azure Marketplace image reference of the virtual machine.
*
*/
@Export(name="galleryImageReference", refs={GalleryImageReferenceResponse.class}, tree="[0]")
private Output* @Nullable */ GalleryImageReferenceResponse> galleryImageReference;
/**
* @return The Microsoft Azure Marketplace image reference of the virtual machine.
*
*/
public Output> galleryImageReference() {
return Codegen.optional(this.galleryImageReference);
}
/**
* Indicates whether this virtual machine uses an SSH key for authentication.
*
*/
@Export(name="isAuthenticationWithSshKey", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> isAuthenticationWithSshKey;
/**
* @return Indicates whether this virtual machine uses an SSH key for authentication.
*
*/
public Output> isAuthenticationWithSshKey() {
return Codegen.optional(this.isAuthenticationWithSshKey);
}
/**
* The lab subnet name of the virtual machine.
*
*/
@Export(name="labSubnetName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> labSubnetName;
/**
* @return The lab subnet name of the virtual machine.
*
*/
public Output> labSubnetName() {
return Codegen.optional(this.labSubnetName);
}
/**
* The lab virtual network identifier of the virtual machine.
*
*/
@Export(name="labVirtualNetworkId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> labVirtualNetworkId;
/**
* @return The lab virtual network identifier of the virtual machine.
*
*/
public Output> labVirtualNetworkId() {
return Codegen.optional(this.labVirtualNetworkId);
}
/**
* Last known compute power state captured in DTL
*
*/
@Export(name="lastKnownPowerState", refs={String.class}, tree="[0]")
private Output lastKnownPowerState;
/**
* @return Last known compute power state captured in DTL
*
*/
public Output lastKnownPowerState() {
return this.lastKnownPowerState;
}
/**
* The location of the resource.
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> location;
/**
* @return The location of the resource.
*
*/
public Output> location() {
return Codegen.optional(this.location);
}
/**
* The name of the resource.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource.
*
*/
public Output name() {
return this.name;
}
/**
* The network interface properties.
*
*/
@Export(name="networkInterface", refs={NetworkInterfacePropertiesResponse.class}, tree="[0]")
private Output* @Nullable */ NetworkInterfacePropertiesResponse> networkInterface;
/**
* @return The network interface properties.
*
*/
public Output> networkInterface() {
return Codegen.optional(this.networkInterface);
}
/**
* The notes of the virtual machine.
*
*/
@Export(name="notes", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> notes;
/**
* @return The notes of the virtual machine.
*
*/
public Output> notes() {
return Codegen.optional(this.notes);
}
/**
* The OS type of the virtual machine.
*
*/
@Export(name="osType", refs={String.class}, tree="[0]")
private Output osType;
/**
* @return The OS type of the virtual machine.
*
*/
public Output osType() {
return this.osType;
}
/**
* The object identifier of the owner of the virtual machine.
*
*/
@Export(name="ownerObjectId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> ownerObjectId;
/**
* @return The object identifier of the owner of the virtual machine.
*
*/
public Output> ownerObjectId() {
return Codegen.optional(this.ownerObjectId);
}
/**
* The user principal name of the virtual machine owner.
*
*/
@Export(name="ownerUserPrincipalName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> ownerUserPrincipalName;
/**
* @return The user principal name of the virtual machine owner.
*
*/
public Output> ownerUserPrincipalName() {
return Codegen.optional(this.ownerUserPrincipalName);
}
/**
* The password of the virtual machine administrator.
*
*/
@Export(name="password", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> password;
/**
* @return The password of the virtual machine administrator.
*
*/
public Output> password() {
return Codegen.optional(this.password);
}
/**
* The id of the plan associated with the virtual machine image
*
*/
@Export(name="planId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> planId;
/**
* @return The id of the plan associated with the virtual machine image
*
*/
public Output> planId() {
return Codegen.optional(this.planId);
}
/**
* The provisioning status of the resource.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The provisioning status of the resource.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* Virtual Machine schedules to be created
*
*/
@Export(name="scheduleParameters", refs={List.class,ScheduleCreationParameterResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> scheduleParameters;
/**
* @return Virtual Machine schedules to be created
*
*/
public Output>> scheduleParameters() {
return Codegen.optional(this.scheduleParameters);
}
/**
* The size of the virtual machine.
*
*/
@Export(name="size", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> size;
/**
* @return The size of the virtual machine.
*
*/
public Output> size() {
return Codegen.optional(this.size);
}
/**
* The SSH key of the virtual machine administrator.
*
*/
@Export(name="sshKey", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> sshKey;
/**
* @return The SSH key of the virtual machine administrator.
*
*/
public Output> sshKey() {
return Codegen.optional(this.sshKey);
}
/**
* Storage type to use for virtual machine (i.e. Standard, Premium).
*
*/
@Export(name="storageType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> storageType;
/**
* @return Storage type to use for virtual machine (i.e. Standard, Premium).
*
*/
public Output> storageType() {
return Codegen.optional(this.storageType);
}
/**
* The tags of the resource.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return The tags of the resource.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* The type of the resource.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource.
*
*/
public Output type() {
return this.type;
}
/**
* The unique immutable identifier of a resource (Guid).
*
*/
@Export(name="uniqueIdentifier", refs={String.class}, tree="[0]")
private Output uniqueIdentifier;
/**
* @return The unique immutable identifier of a resource (Guid).
*
*/
public Output uniqueIdentifier() {
return this.uniqueIdentifier;
}
/**
* The user name of the virtual machine.
*
*/
@Export(name="userName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> userName;
/**
* @return The user name of the virtual machine.
*
*/
public Output> userName() {
return Codegen.optional(this.userName);
}
/**
* Tells source of creation of lab virtual machine. Output property only.
*
*/
@Export(name="virtualMachineCreationSource", refs={String.class}, tree="[0]")
private Output virtualMachineCreationSource;
/**
* @return Tells source of creation of lab virtual machine. Output property only.
*
*/
public Output virtualMachineCreationSource() {
return this.virtualMachineCreationSource;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public VirtualMachine(java.lang.String name) {
this(name, VirtualMachineArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public VirtualMachine(java.lang.String name, VirtualMachineArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public VirtualMachine(java.lang.String name, VirtualMachineArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:devtestlab:VirtualMachine", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private VirtualMachine(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:devtestlab:VirtualMachine", name, null, makeResourceOptions(options, id), false);
}
private static VirtualMachineArgs makeArgs(VirtualMachineArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? VirtualMachineArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:devtestlab/v20150521preview:VirtualMachine").build()),
Output.of(Alias.builder().type("azure-native:devtestlab/v20160515:VirtualMachine").build()),
Output.of(Alias.builder().type("azure-native:devtestlab/v20180915:VirtualMachine").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static VirtualMachine get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new VirtualMachine(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy