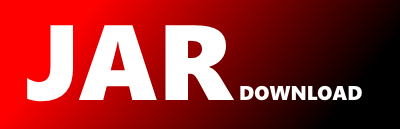
com.pulumi.azurenative.digitaltwins.inputs.EventHubArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.digitaltwins.inputs;
import com.pulumi.azurenative.digitaltwins.enums.AuthenticationType;
import com.pulumi.azurenative.digitaltwins.inputs.ManagedIdentityReferenceArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Properties related to EventHub.
*
*/
public final class EventHubArgs extends com.pulumi.resources.ResourceArgs {
public static final EventHubArgs Empty = new EventHubArgs();
/**
* Specifies the authentication type being used for connecting to the endpoint. Defaults to 'KeyBased'. If 'KeyBased' is selected, a connection string must be specified (at least the primary connection string). If 'IdentityBased' is select, the endpointUri and entityPath properties must be specified.
*
*/
@Import(name="authenticationType")
private @Nullable Output> authenticationType;
/**
* @return Specifies the authentication type being used for connecting to the endpoint. Defaults to 'KeyBased'. If 'KeyBased' is selected, a connection string must be specified (at least the primary connection string). If 'IdentityBased' is select, the endpointUri and entityPath properties must be specified.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy