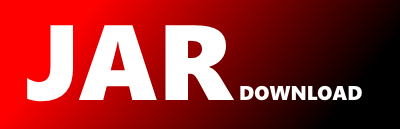
com.pulumi.azurenative.digitaltwins.outputs.AzureDataExplorerConnectionPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.digitaltwins.outputs;
import com.pulumi.azurenative.digitaltwins.outputs.ManagedIdentityReferenceResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AzureDataExplorerConnectionPropertiesResponse {
/**
* @return The name of the Azure Data Explorer database.
*
*/
private String adxDatabaseName;
/**
* @return The URI of the Azure Data Explorer endpoint.
*
*/
private String adxEndpointUri;
/**
* @return The name of the Azure Data Explorer table used for recording relationship lifecycle events. The table will not be created if this property is left unspecified.
*
*/
private @Nullable String adxRelationshipLifecycleEventsTableName;
/**
* @return The resource ID of the Azure Data Explorer cluster.
*
*/
private String adxResourceId;
/**
* @return The name of the Azure Data Explorer table used for storing updates to properties of twins and relationships. Defaults to AdtPropertyEvents.
*
*/
private @Nullable String adxTableName;
/**
* @return The name of the Azure Data Explorer table used for recording twin lifecycle events. The table will not be created if this property is left unspecified.
*
*/
private @Nullable String adxTwinLifecycleEventsTableName;
/**
* @return The type of time series connection resource.
* Expected value is 'AzureDataExplorer'.
*
*/
private String connectionType;
/**
* @return The EventHub consumer group to use when ADX reads from EventHub. Defaults to $Default.
*
*/
private @Nullable String eventHubConsumerGroup;
/**
* @return The URL of the EventHub namespace for identity-based authentication. It must include the protocol sb://
*
*/
private String eventHubEndpointUri;
/**
* @return The EventHub name in the EventHub namespace for identity-based authentication.
*
*/
private String eventHubEntityPath;
/**
* @return The resource ID of the EventHub namespace.
*
*/
private String eventHubNamespaceResourceId;
/**
* @return Managed identity properties for the time series database connection resource.
*
*/
private @Nullable ManagedIdentityReferenceResponse identity;
/**
* @return The provisioning state.
*
*/
private String provisioningState;
/**
* @return Specifies whether or not to record twin / relationship property and item removals, including removals of indexed or keyed values (such as map entries, array elements, etc.). This feature is de-activated unless explicitly set to 'true'. Setting this property to 'true' will generate an additional column in the property events table in ADX.
*
*/
private @Nullable String recordPropertyAndItemRemovals;
private AzureDataExplorerConnectionPropertiesResponse() {}
/**
* @return The name of the Azure Data Explorer database.
*
*/
public String adxDatabaseName() {
return this.adxDatabaseName;
}
/**
* @return The URI of the Azure Data Explorer endpoint.
*
*/
public String adxEndpointUri() {
return this.adxEndpointUri;
}
/**
* @return The name of the Azure Data Explorer table used for recording relationship lifecycle events. The table will not be created if this property is left unspecified.
*
*/
public Optional adxRelationshipLifecycleEventsTableName() {
return Optional.ofNullable(this.adxRelationshipLifecycleEventsTableName);
}
/**
* @return The resource ID of the Azure Data Explorer cluster.
*
*/
public String adxResourceId() {
return this.adxResourceId;
}
/**
* @return The name of the Azure Data Explorer table used for storing updates to properties of twins and relationships. Defaults to AdtPropertyEvents.
*
*/
public Optional adxTableName() {
return Optional.ofNullable(this.adxTableName);
}
/**
* @return The name of the Azure Data Explorer table used for recording twin lifecycle events. The table will not be created if this property is left unspecified.
*
*/
public Optional adxTwinLifecycleEventsTableName() {
return Optional.ofNullable(this.adxTwinLifecycleEventsTableName);
}
/**
* @return The type of time series connection resource.
* Expected value is 'AzureDataExplorer'.
*
*/
public String connectionType() {
return this.connectionType;
}
/**
* @return The EventHub consumer group to use when ADX reads from EventHub. Defaults to $Default.
*
*/
public Optional eventHubConsumerGroup() {
return Optional.ofNullable(this.eventHubConsumerGroup);
}
/**
* @return The URL of the EventHub namespace for identity-based authentication. It must include the protocol sb://
*
*/
public String eventHubEndpointUri() {
return this.eventHubEndpointUri;
}
/**
* @return The EventHub name in the EventHub namespace for identity-based authentication.
*
*/
public String eventHubEntityPath() {
return this.eventHubEntityPath;
}
/**
* @return The resource ID of the EventHub namespace.
*
*/
public String eventHubNamespaceResourceId() {
return this.eventHubNamespaceResourceId;
}
/**
* @return Managed identity properties for the time series database connection resource.
*
*/
public Optional identity() {
return Optional.ofNullable(this.identity);
}
/**
* @return The provisioning state.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Specifies whether or not to record twin / relationship property and item removals, including removals of indexed or keyed values (such as map entries, array elements, etc.). This feature is de-activated unless explicitly set to 'true'. Setting this property to 'true' will generate an additional column in the property events table in ADX.
*
*/
public Optional recordPropertyAndItemRemovals() {
return Optional.ofNullable(this.recordPropertyAndItemRemovals);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AzureDataExplorerConnectionPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String adxDatabaseName;
private String adxEndpointUri;
private @Nullable String adxRelationshipLifecycleEventsTableName;
private String adxResourceId;
private @Nullable String adxTableName;
private @Nullable String adxTwinLifecycleEventsTableName;
private String connectionType;
private @Nullable String eventHubConsumerGroup;
private String eventHubEndpointUri;
private String eventHubEntityPath;
private String eventHubNamespaceResourceId;
private @Nullable ManagedIdentityReferenceResponse identity;
private String provisioningState;
private @Nullable String recordPropertyAndItemRemovals;
public Builder() {}
public Builder(AzureDataExplorerConnectionPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.adxDatabaseName = defaults.adxDatabaseName;
this.adxEndpointUri = defaults.adxEndpointUri;
this.adxRelationshipLifecycleEventsTableName = defaults.adxRelationshipLifecycleEventsTableName;
this.adxResourceId = defaults.adxResourceId;
this.adxTableName = defaults.adxTableName;
this.adxTwinLifecycleEventsTableName = defaults.adxTwinLifecycleEventsTableName;
this.connectionType = defaults.connectionType;
this.eventHubConsumerGroup = defaults.eventHubConsumerGroup;
this.eventHubEndpointUri = defaults.eventHubEndpointUri;
this.eventHubEntityPath = defaults.eventHubEntityPath;
this.eventHubNamespaceResourceId = defaults.eventHubNamespaceResourceId;
this.identity = defaults.identity;
this.provisioningState = defaults.provisioningState;
this.recordPropertyAndItemRemovals = defaults.recordPropertyAndItemRemovals;
}
@CustomType.Setter
public Builder adxDatabaseName(String adxDatabaseName) {
if (adxDatabaseName == null) {
throw new MissingRequiredPropertyException("AzureDataExplorerConnectionPropertiesResponse", "adxDatabaseName");
}
this.adxDatabaseName = adxDatabaseName;
return this;
}
@CustomType.Setter
public Builder adxEndpointUri(String adxEndpointUri) {
if (adxEndpointUri == null) {
throw new MissingRequiredPropertyException("AzureDataExplorerConnectionPropertiesResponse", "adxEndpointUri");
}
this.adxEndpointUri = adxEndpointUri;
return this;
}
@CustomType.Setter
public Builder adxRelationshipLifecycleEventsTableName(@Nullable String adxRelationshipLifecycleEventsTableName) {
this.adxRelationshipLifecycleEventsTableName = adxRelationshipLifecycleEventsTableName;
return this;
}
@CustomType.Setter
public Builder adxResourceId(String adxResourceId) {
if (adxResourceId == null) {
throw new MissingRequiredPropertyException("AzureDataExplorerConnectionPropertiesResponse", "adxResourceId");
}
this.adxResourceId = adxResourceId;
return this;
}
@CustomType.Setter
public Builder adxTableName(@Nullable String adxTableName) {
this.adxTableName = adxTableName;
return this;
}
@CustomType.Setter
public Builder adxTwinLifecycleEventsTableName(@Nullable String adxTwinLifecycleEventsTableName) {
this.adxTwinLifecycleEventsTableName = adxTwinLifecycleEventsTableName;
return this;
}
@CustomType.Setter
public Builder connectionType(String connectionType) {
if (connectionType == null) {
throw new MissingRequiredPropertyException("AzureDataExplorerConnectionPropertiesResponse", "connectionType");
}
this.connectionType = connectionType;
return this;
}
@CustomType.Setter
public Builder eventHubConsumerGroup(@Nullable String eventHubConsumerGroup) {
this.eventHubConsumerGroup = eventHubConsumerGroup;
return this;
}
@CustomType.Setter
public Builder eventHubEndpointUri(String eventHubEndpointUri) {
if (eventHubEndpointUri == null) {
throw new MissingRequiredPropertyException("AzureDataExplorerConnectionPropertiesResponse", "eventHubEndpointUri");
}
this.eventHubEndpointUri = eventHubEndpointUri;
return this;
}
@CustomType.Setter
public Builder eventHubEntityPath(String eventHubEntityPath) {
if (eventHubEntityPath == null) {
throw new MissingRequiredPropertyException("AzureDataExplorerConnectionPropertiesResponse", "eventHubEntityPath");
}
this.eventHubEntityPath = eventHubEntityPath;
return this;
}
@CustomType.Setter
public Builder eventHubNamespaceResourceId(String eventHubNamespaceResourceId) {
if (eventHubNamespaceResourceId == null) {
throw new MissingRequiredPropertyException("AzureDataExplorerConnectionPropertiesResponse", "eventHubNamespaceResourceId");
}
this.eventHubNamespaceResourceId = eventHubNamespaceResourceId;
return this;
}
@CustomType.Setter
public Builder identity(@Nullable ManagedIdentityReferenceResponse identity) {
this.identity = identity;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("AzureDataExplorerConnectionPropertiesResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder recordPropertyAndItemRemovals(@Nullable String recordPropertyAndItemRemovals) {
this.recordPropertyAndItemRemovals = recordPropertyAndItemRemovals;
return this;
}
public AzureDataExplorerConnectionPropertiesResponse build() {
final var _resultValue = new AzureDataExplorerConnectionPropertiesResponse();
_resultValue.adxDatabaseName = adxDatabaseName;
_resultValue.adxEndpointUri = adxEndpointUri;
_resultValue.adxRelationshipLifecycleEventsTableName = adxRelationshipLifecycleEventsTableName;
_resultValue.adxResourceId = adxResourceId;
_resultValue.adxTableName = adxTableName;
_resultValue.adxTwinLifecycleEventsTableName = adxTwinLifecycleEventsTableName;
_resultValue.connectionType = connectionType;
_resultValue.eventHubConsumerGroup = eventHubConsumerGroup;
_resultValue.eventHubEndpointUri = eventHubEndpointUri;
_resultValue.eventHubEntityPath = eventHubEntityPath;
_resultValue.eventHubNamespaceResourceId = eventHubNamespaceResourceId;
_resultValue.identity = identity;
_resultValue.provisioningState = provisioningState;
_resultValue.recordPropertyAndItemRemovals = recordPropertyAndItemRemovals;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy