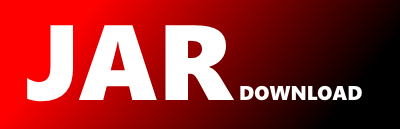
com.pulumi.azurenative.domainregistration.DomainArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.domainregistration;
import com.pulumi.azurenative.domainregistration.enums.DnsType;
import com.pulumi.azurenative.domainregistration.inputs.ContactArgs;
import com.pulumi.azurenative.domainregistration.inputs.DomainPurchaseConsentArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DomainArgs extends com.pulumi.resources.ResourceArgs {
public static final DomainArgs Empty = new DomainArgs();
@Import(name="authCode")
private @Nullable Output authCode;
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy