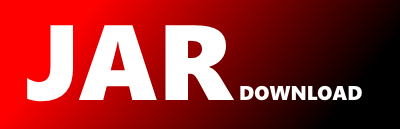
com.pulumi.azurenative.edgeorder.outputs.ConfigurationDeviceDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.edgeorder.outputs;
import com.pulumi.azurenative.edgeorder.outputs.DeviceDetailsResponse;
import com.pulumi.azurenative.edgeorder.outputs.DisplayInfoResponse;
import com.pulumi.azurenative.edgeorder.outputs.HierarchyInformationResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ConfigurationDeviceDetailsResponse {
/**
* @return List of device details.
*
*/
private List deviceDetails;
/**
* @return Display details of the product.
*
*/
private @Nullable DisplayInfoResponse displayInfo;
/**
* @return Hierarchy of the product which uniquely identifies the configuration.
*
*/
private HierarchyInformationResponse hierarchyInformation;
/**
* @return Identification type of the configuration.
*
*/
private String identificationType;
/**
* @return Quantity of the product.
*
*/
private Integer quantity;
private ConfigurationDeviceDetailsResponse() {}
/**
* @return List of device details.
*
*/
public List deviceDetails() {
return this.deviceDetails;
}
/**
* @return Display details of the product.
*
*/
public Optional displayInfo() {
return Optional.ofNullable(this.displayInfo);
}
/**
* @return Hierarchy of the product which uniquely identifies the configuration.
*
*/
public HierarchyInformationResponse hierarchyInformation() {
return this.hierarchyInformation;
}
/**
* @return Identification type of the configuration.
*
*/
public String identificationType() {
return this.identificationType;
}
/**
* @return Quantity of the product.
*
*/
public Integer quantity() {
return this.quantity;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ConfigurationDeviceDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List deviceDetails;
private @Nullable DisplayInfoResponse displayInfo;
private HierarchyInformationResponse hierarchyInformation;
private String identificationType;
private Integer quantity;
public Builder() {}
public Builder(ConfigurationDeviceDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.deviceDetails = defaults.deviceDetails;
this.displayInfo = defaults.displayInfo;
this.hierarchyInformation = defaults.hierarchyInformation;
this.identificationType = defaults.identificationType;
this.quantity = defaults.quantity;
}
@CustomType.Setter
public Builder deviceDetails(List deviceDetails) {
if (deviceDetails == null) {
throw new MissingRequiredPropertyException("ConfigurationDeviceDetailsResponse", "deviceDetails");
}
this.deviceDetails = deviceDetails;
return this;
}
public Builder deviceDetails(DeviceDetailsResponse... deviceDetails) {
return deviceDetails(List.of(deviceDetails));
}
@CustomType.Setter
public Builder displayInfo(@Nullable DisplayInfoResponse displayInfo) {
this.displayInfo = displayInfo;
return this;
}
@CustomType.Setter
public Builder hierarchyInformation(HierarchyInformationResponse hierarchyInformation) {
if (hierarchyInformation == null) {
throw new MissingRequiredPropertyException("ConfigurationDeviceDetailsResponse", "hierarchyInformation");
}
this.hierarchyInformation = hierarchyInformation;
return this;
}
@CustomType.Setter
public Builder identificationType(String identificationType) {
if (identificationType == null) {
throw new MissingRequiredPropertyException("ConfigurationDeviceDetailsResponse", "identificationType");
}
this.identificationType = identificationType;
return this;
}
@CustomType.Setter
public Builder quantity(Integer quantity) {
if (quantity == null) {
throw new MissingRequiredPropertyException("ConfigurationDeviceDetailsResponse", "quantity");
}
this.quantity = quantity;
return this;
}
public ConfigurationDeviceDetailsResponse build() {
final var _resultValue = new ConfigurationDeviceDetailsResponse();
_resultValue.deviceDetails = deviceDetails;
_resultValue.displayInfo = displayInfo;
_resultValue.hierarchyInformation = hierarchyInformation;
_resultValue.identificationType = identificationType;
_resultValue.quantity = quantity;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy