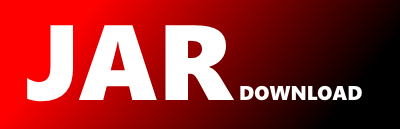
com.pulumi.azurenative.edgeorder.outputs.ProductLineResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.edgeorder.outputs;
import com.pulumi.azurenative.edgeorder.outputs.AvailabilityInformationResponse;
import com.pulumi.azurenative.edgeorder.outputs.CostInformationResponse;
import com.pulumi.azurenative.edgeorder.outputs.DescriptionResponse;
import com.pulumi.azurenative.edgeorder.outputs.FilterablePropertyResponse;
import com.pulumi.azurenative.edgeorder.outputs.HierarchyInformationResponse;
import com.pulumi.azurenative.edgeorder.outputs.ImageInformationResponse;
import com.pulumi.azurenative.edgeorder.outputs.ProductResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class ProductLineResponse {
/**
* @return Availability information of the product system.
*
*/
private AvailabilityInformationResponse availabilityInformation;
/**
* @return Cost information for the product system.
*
*/
private CostInformationResponse costInformation;
/**
* @return Description related to the product system.
*
*/
private DescriptionResponse description;
/**
* @return Display Name for the product system.
*
*/
private String displayName;
/**
* @return List of filters supported for a product.
*
*/
private List filterableProperties;
/**
* @return The entity responsible for fulfillment of the item at the given hierarchy level.
*
*/
private String fulfilledBy;
/**
* @return Hierarchy information of a product.
*
*/
private HierarchyInformationResponse hierarchyInformation;
/**
* @return Image information for the product system.
*
*/
private List imageInformation;
/**
* @return List of products in the product line.
*
*/
private List products;
private ProductLineResponse() {}
/**
* @return Availability information of the product system.
*
*/
public AvailabilityInformationResponse availabilityInformation() {
return this.availabilityInformation;
}
/**
* @return Cost information for the product system.
*
*/
public CostInformationResponse costInformation() {
return this.costInformation;
}
/**
* @return Description related to the product system.
*
*/
public DescriptionResponse description() {
return this.description;
}
/**
* @return Display Name for the product system.
*
*/
public String displayName() {
return this.displayName;
}
/**
* @return List of filters supported for a product.
*
*/
public List filterableProperties() {
return this.filterableProperties;
}
/**
* @return The entity responsible for fulfillment of the item at the given hierarchy level.
*
*/
public String fulfilledBy() {
return this.fulfilledBy;
}
/**
* @return Hierarchy information of a product.
*
*/
public HierarchyInformationResponse hierarchyInformation() {
return this.hierarchyInformation;
}
/**
* @return Image information for the product system.
*
*/
public List imageInformation() {
return this.imageInformation;
}
/**
* @return List of products in the product line.
*
*/
public List products() {
return this.products;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ProductLineResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private AvailabilityInformationResponse availabilityInformation;
private CostInformationResponse costInformation;
private DescriptionResponse description;
private String displayName;
private List filterableProperties;
private String fulfilledBy;
private HierarchyInformationResponse hierarchyInformation;
private List imageInformation;
private List products;
public Builder() {}
public Builder(ProductLineResponse defaults) {
Objects.requireNonNull(defaults);
this.availabilityInformation = defaults.availabilityInformation;
this.costInformation = defaults.costInformation;
this.description = defaults.description;
this.displayName = defaults.displayName;
this.filterableProperties = defaults.filterableProperties;
this.fulfilledBy = defaults.fulfilledBy;
this.hierarchyInformation = defaults.hierarchyInformation;
this.imageInformation = defaults.imageInformation;
this.products = defaults.products;
}
@CustomType.Setter
public Builder availabilityInformation(AvailabilityInformationResponse availabilityInformation) {
if (availabilityInformation == null) {
throw new MissingRequiredPropertyException("ProductLineResponse", "availabilityInformation");
}
this.availabilityInformation = availabilityInformation;
return this;
}
@CustomType.Setter
public Builder costInformation(CostInformationResponse costInformation) {
if (costInformation == null) {
throw new MissingRequiredPropertyException("ProductLineResponse", "costInformation");
}
this.costInformation = costInformation;
return this;
}
@CustomType.Setter
public Builder description(DescriptionResponse description) {
if (description == null) {
throw new MissingRequiredPropertyException("ProductLineResponse", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder displayName(String displayName) {
if (displayName == null) {
throw new MissingRequiredPropertyException("ProductLineResponse", "displayName");
}
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder filterableProperties(List filterableProperties) {
if (filterableProperties == null) {
throw new MissingRequiredPropertyException("ProductLineResponse", "filterableProperties");
}
this.filterableProperties = filterableProperties;
return this;
}
public Builder filterableProperties(FilterablePropertyResponse... filterableProperties) {
return filterableProperties(List.of(filterableProperties));
}
@CustomType.Setter
public Builder fulfilledBy(String fulfilledBy) {
if (fulfilledBy == null) {
throw new MissingRequiredPropertyException("ProductLineResponse", "fulfilledBy");
}
this.fulfilledBy = fulfilledBy;
return this;
}
@CustomType.Setter
public Builder hierarchyInformation(HierarchyInformationResponse hierarchyInformation) {
if (hierarchyInformation == null) {
throw new MissingRequiredPropertyException("ProductLineResponse", "hierarchyInformation");
}
this.hierarchyInformation = hierarchyInformation;
return this;
}
@CustomType.Setter
public Builder imageInformation(List imageInformation) {
if (imageInformation == null) {
throw new MissingRequiredPropertyException("ProductLineResponse", "imageInformation");
}
this.imageInformation = imageInformation;
return this;
}
public Builder imageInformation(ImageInformationResponse... imageInformation) {
return imageInformation(List.of(imageInformation));
}
@CustomType.Setter
public Builder products(List products) {
if (products == null) {
throw new MissingRequiredPropertyException("ProductLineResponse", "products");
}
this.products = products;
return this;
}
public Builder products(ProductResponse... products) {
return products(List.of(products));
}
public ProductLineResponse build() {
final var _resultValue = new ProductLineResponse();
_resultValue.availabilityInformation = availabilityInformation;
_resultValue.costInformation = costInformation;
_resultValue.description = description;
_resultValue.displayName = displayName;
_resultValue.filterableProperties = filterableProperties;
_resultValue.fulfilledBy = fulfilledBy;
_resultValue.hierarchyInformation = hierarchyInformation;
_resultValue.imageInformation = imageInformation;
_resultValue.products = products;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy