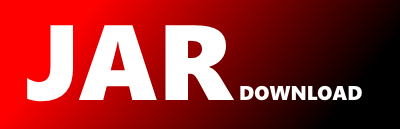
com.pulumi.azurenative.engagementfabric.ChannelArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.engagementfabric;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ChannelArgs extends com.pulumi.resources.ResourceArgs {
public static final ChannelArgs Empty = new ChannelArgs();
/**
* Account Name
*
*/
@Import(name="accountName", required=true)
private Output accountName;
/**
* @return Account Name
*
*/
public Output accountName() {
return this.accountName;
}
/**
* The functions to be enabled for the channel
*
*/
@Import(name="channelFunctions")
private @Nullable Output> channelFunctions;
/**
* @return The functions to be enabled for the channel
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy