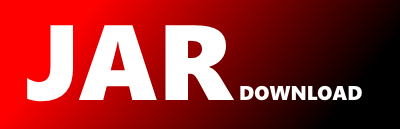
com.pulumi.azurenative.engagementfabric.EngagementfabricFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.engagementfabric;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.engagementfabric.inputs.GetAccountArgs;
import com.pulumi.azurenative.engagementfabric.inputs.GetAccountPlainArgs;
import com.pulumi.azurenative.engagementfabric.inputs.GetChannelArgs;
import com.pulumi.azurenative.engagementfabric.inputs.GetChannelPlainArgs;
import com.pulumi.azurenative.engagementfabric.inputs.ListAccountChannelTypesArgs;
import com.pulumi.azurenative.engagementfabric.inputs.ListAccountChannelTypesPlainArgs;
import com.pulumi.azurenative.engagementfabric.inputs.ListAccountKeysArgs;
import com.pulumi.azurenative.engagementfabric.inputs.ListAccountKeysPlainArgs;
import com.pulumi.azurenative.engagementfabric.outputs.GetAccountResult;
import com.pulumi.azurenative.engagementfabric.outputs.GetChannelResult;
import com.pulumi.azurenative.engagementfabric.outputs.ListAccountChannelTypesResult;
import com.pulumi.azurenative.engagementfabric.outputs.ListAccountKeysResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class EngagementfabricFunctions {
/**
* The EngagementFabric account
* Azure REST API version: 2018-09-01-preview.
*
*/
public static Output getAccount(GetAccountArgs args) {
return getAccount(args, InvokeOptions.Empty);
}
/**
* The EngagementFabric account
* Azure REST API version: 2018-09-01-preview.
*
*/
public static CompletableFuture getAccountPlain(GetAccountPlainArgs args) {
return getAccountPlain(args, InvokeOptions.Empty);
}
/**
* The EngagementFabric account
* Azure REST API version: 2018-09-01-preview.
*
*/
public static Output getAccount(GetAccountArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:engagementfabric:getAccount", TypeShape.of(GetAccountResult.class), args, Utilities.withVersion(options));
}
/**
* The EngagementFabric account
* Azure REST API version: 2018-09-01-preview.
*
*/
public static CompletableFuture getAccountPlain(GetAccountPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:engagementfabric:getAccount", TypeShape.of(GetAccountResult.class), args, Utilities.withVersion(options));
}
/**
* The EngagementFabric channel
* Azure REST API version: 2018-09-01-preview.
*
*/
public static Output getChannel(GetChannelArgs args) {
return getChannel(args, InvokeOptions.Empty);
}
/**
* The EngagementFabric channel
* Azure REST API version: 2018-09-01-preview.
*
*/
public static CompletableFuture getChannelPlain(GetChannelPlainArgs args) {
return getChannelPlain(args, InvokeOptions.Empty);
}
/**
* The EngagementFabric channel
* Azure REST API version: 2018-09-01-preview.
*
*/
public static Output getChannel(GetChannelArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:engagementfabric:getChannel", TypeShape.of(GetChannelResult.class), args, Utilities.withVersion(options));
}
/**
* The EngagementFabric channel
* Azure REST API version: 2018-09-01-preview.
*
*/
public static CompletableFuture getChannelPlain(GetChannelPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:engagementfabric:getChannel", TypeShape.of(GetChannelResult.class), args, Utilities.withVersion(options));
}
/**
* List of the EngagementFabric channel descriptions
* Azure REST API version: 2018-09-01-preview.
*
*/
public static Output listAccountChannelTypes(ListAccountChannelTypesArgs args) {
return listAccountChannelTypes(args, InvokeOptions.Empty);
}
/**
* List of the EngagementFabric channel descriptions
* Azure REST API version: 2018-09-01-preview.
*
*/
public static CompletableFuture listAccountChannelTypesPlain(ListAccountChannelTypesPlainArgs args) {
return listAccountChannelTypesPlain(args, InvokeOptions.Empty);
}
/**
* List of the EngagementFabric channel descriptions
* Azure REST API version: 2018-09-01-preview.
*
*/
public static Output listAccountChannelTypes(ListAccountChannelTypesArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:engagementfabric:listAccountChannelTypes", TypeShape.of(ListAccountChannelTypesResult.class), args, Utilities.withVersion(options));
}
/**
* List of the EngagementFabric channel descriptions
* Azure REST API version: 2018-09-01-preview.
*
*/
public static CompletableFuture listAccountChannelTypesPlain(ListAccountChannelTypesPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:engagementfabric:listAccountChannelTypes", TypeShape.of(ListAccountChannelTypesResult.class), args, Utilities.withVersion(options));
}
/**
* The list of the EngagementFabric account keys
* Azure REST API version: 2018-09-01-preview.
*
*/
public static Output listAccountKeys(ListAccountKeysArgs args) {
return listAccountKeys(args, InvokeOptions.Empty);
}
/**
* The list of the EngagementFabric account keys
* Azure REST API version: 2018-09-01-preview.
*
*/
public static CompletableFuture listAccountKeysPlain(ListAccountKeysPlainArgs args) {
return listAccountKeysPlain(args, InvokeOptions.Empty);
}
/**
* The list of the EngagementFabric account keys
* Azure REST API version: 2018-09-01-preview.
*
*/
public static Output listAccountKeys(ListAccountKeysArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:engagementfabric:listAccountKeys", TypeShape.of(ListAccountKeysResult.class), args, Utilities.withVersion(options));
}
/**
* The list of the EngagementFabric account keys
* Azure REST API version: 2018-09-01-preview.
*
*/
public static CompletableFuture listAccountKeysPlain(ListAccountKeysPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:engagementfabric:listAccountKeys", TypeShape.of(ListAccountKeysResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy