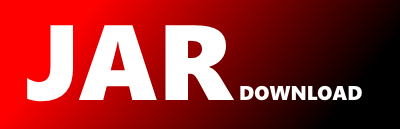
com.pulumi.azurenative.eventgrid.Domain Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.eventgrid;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.eventgrid.DomainArgs;
import com.pulumi.azurenative.eventgrid.outputs.IdentityInfoResponse;
import com.pulumi.azurenative.eventgrid.outputs.InboundIpRuleResponse;
import com.pulumi.azurenative.eventgrid.outputs.JsonInputSchemaMappingResponse;
import com.pulumi.azurenative.eventgrid.outputs.PrivateEndpointConnectionResponse;
import com.pulumi.azurenative.eventgrid.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* EventGrid Domain.
* Azure REST API version: 2022-06-15. Prior API version in Azure Native 1.x: 2020-06-01.
*
* Other available API versions: 2020-04-01-preview, 2023-06-01-preview, 2023-12-15-preview, 2024-06-01-preview.
*
* ## Example Usage
* ### Domains_CreateOrUpdate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.eventgrid.Domain;
* import com.pulumi.azurenative.eventgrid.DomainArgs;
* import com.pulumi.azurenative.eventgrid.inputs.InboundIpRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var domain = new Domain("domain", DomainArgs.builder()
* .domainName("exampledomain1")
* .inboundIpRules(
* InboundIpRuleArgs.builder()
* .action("Allow")
* .ipMask("12.18.30.15")
* .build(),
* InboundIpRuleArgs.builder()
* .action("Allow")
* .ipMask("12.18.176.1")
* .build())
* .location("westus2")
* .publicNetworkAccess("Enabled")
* .resourceGroupName("examplerg")
* .tags(Map.ofEntries(
* Map.entry("tag1", "value1"),
* Map.entry("tag2", "value2")
* ))
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:eventgrid:Domain exampledomain1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.EventGrid/domains/{domainName}
* ```
*
*/
@ResourceType(type="azure-native:eventgrid:Domain")
public class Domain extends com.pulumi.resources.CustomResource {
/**
* This Boolean is used to specify the creation mechanism for 'all' the Event Grid Domain Topics associated with this Event Grid Domain resource.
* In this context, creation of domain topic can be auto-managed (when true) or self-managed (when false). The default value for this property is true.
* When this property is null or set to true, Event Grid is responsible of automatically creating the domain topic when the first event subscription is
* created at the scope of the domain topic. If this property is set to false, then creating the first event subscription will require creating a domain topic
* by the user. The self-management mode can be used if the user wants full control of when the domain topic is created, while auto-managed mode provides the
* flexibility to perform less operations and manage fewer resources by the user. Also, note that in auto-managed creation mode, user is allowed to create the
* domain topic on demand if needed.
*
*/
@Export(name="autoCreateTopicWithFirstSubscription", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> autoCreateTopicWithFirstSubscription;
/**
* @return This Boolean is used to specify the creation mechanism for 'all' the Event Grid Domain Topics associated with this Event Grid Domain resource.
* In this context, creation of domain topic can be auto-managed (when true) or self-managed (when false). The default value for this property is true.
* When this property is null or set to true, Event Grid is responsible of automatically creating the domain topic when the first event subscription is
* created at the scope of the domain topic. If this property is set to false, then creating the first event subscription will require creating a domain topic
* by the user. The self-management mode can be used if the user wants full control of when the domain topic is created, while auto-managed mode provides the
* flexibility to perform less operations and manage fewer resources by the user. Also, note that in auto-managed creation mode, user is allowed to create the
* domain topic on demand if needed.
*
*/
public Output> autoCreateTopicWithFirstSubscription() {
return Codegen.optional(this.autoCreateTopicWithFirstSubscription);
}
/**
* This Boolean is used to specify the deletion mechanism for 'all' the Event Grid Domain Topics associated with this Event Grid Domain resource.
* In this context, deletion of domain topic can be auto-managed (when true) or self-managed (when false). The default value for this property is true.
* When this property is set to true, Event Grid is responsible of automatically deleting the domain topic when the last event subscription at the scope
* of the domain topic is deleted. If this property is set to false, then the user needs to manually delete the domain topic when it is no longer needed
* (e.g., when last event subscription is deleted and the resource needs to be cleaned up). The self-management mode can be used if the user wants full
* control of when the domain topic needs to be deleted, while auto-managed mode provides the flexibility to perform less operations and manage fewer
* resources by the user.
*
*/
@Export(name="autoDeleteTopicWithLastSubscription", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> autoDeleteTopicWithLastSubscription;
/**
* @return This Boolean is used to specify the deletion mechanism for 'all' the Event Grid Domain Topics associated with this Event Grid Domain resource.
* In this context, deletion of domain topic can be auto-managed (when true) or self-managed (when false). The default value for this property is true.
* When this property is set to true, Event Grid is responsible of automatically deleting the domain topic when the last event subscription at the scope
* of the domain topic is deleted. If this property is set to false, then the user needs to manually delete the domain topic when it is no longer needed
* (e.g., when last event subscription is deleted and the resource needs to be cleaned up). The self-management mode can be used if the user wants full
* control of when the domain topic needs to be deleted, while auto-managed mode provides the flexibility to perform less operations and manage fewer
* resources by the user.
*
*/
public Output> autoDeleteTopicWithLastSubscription() {
return Codegen.optional(this.autoDeleteTopicWithLastSubscription);
}
/**
* Data Residency Boundary of the resource.
*
*/
@Export(name="dataResidencyBoundary", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> dataResidencyBoundary;
/**
* @return Data Residency Boundary of the resource.
*
*/
public Output> dataResidencyBoundary() {
return Codegen.optional(this.dataResidencyBoundary);
}
/**
* This boolean is used to enable or disable local auth. Default value is false. When the property is set to true, only AAD token will be used to authenticate if user is allowed to publish to the domain.
*
*/
@Export(name="disableLocalAuth", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> disableLocalAuth;
/**
* @return This boolean is used to enable or disable local auth. Default value is false. When the property is set to true, only AAD token will be used to authenticate if user is allowed to publish to the domain.
*
*/
public Output> disableLocalAuth() {
return Codegen.optional(this.disableLocalAuth);
}
/**
* Endpoint for the Event Grid Domain Resource which is used for publishing the events.
*
*/
@Export(name="endpoint", refs={String.class}, tree="[0]")
private Output endpoint;
/**
* @return Endpoint for the Event Grid Domain Resource which is used for publishing the events.
*
*/
public Output endpoint() {
return this.endpoint;
}
/**
* Identity information for the Event Grid Domain resource.
*
*/
@Export(name="identity", refs={IdentityInfoResponse.class}, tree="[0]")
private Output* @Nullable */ IdentityInfoResponse> identity;
/**
* @return Identity information for the Event Grid Domain resource.
*
*/
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* This can be used to restrict traffic from specific IPs instead of all IPs. Note: These are considered only if PublicNetworkAccess is enabled.
*
*/
@Export(name="inboundIpRules", refs={List.class,InboundIpRuleResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> inboundIpRules;
/**
* @return This can be used to restrict traffic from specific IPs instead of all IPs. Note: These are considered only if PublicNetworkAccess is enabled.
*
*/
public Output>> inboundIpRules() {
return Codegen.optional(this.inboundIpRules);
}
/**
* This determines the format that Event Grid should expect for incoming events published to the Event Grid Domain Resource.
*
*/
@Export(name="inputSchema", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> inputSchema;
/**
* @return This determines the format that Event Grid should expect for incoming events published to the Event Grid Domain Resource.
*
*/
public Output> inputSchema() {
return Codegen.optional(this.inputSchema);
}
/**
* Information about the InputSchemaMapping which specified the info about mapping event payload.
*
*/
@Export(name="inputSchemaMapping", refs={JsonInputSchemaMappingResponse.class}, tree="[0]")
private Output* @Nullable */ JsonInputSchemaMappingResponse> inputSchemaMapping;
/**
* @return Information about the InputSchemaMapping which specified the info about mapping event payload.
*
*/
public Output> inputSchemaMapping() {
return Codegen.optional(this.inputSchemaMapping);
}
/**
* Location of the resource.
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return Location of the resource.
*
*/
public Output location() {
return this.location;
}
/**
* Metric resource id for the Event Grid Domain Resource.
*
*/
@Export(name="metricResourceId", refs={String.class}, tree="[0]")
private Output metricResourceId;
/**
* @return Metric resource id for the Event Grid Domain Resource.
*
*/
public Output metricResourceId() {
return this.metricResourceId;
}
/**
* Name of the resource.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Name of the resource.
*
*/
public Output name() {
return this.name;
}
/**
* List of private endpoint connections.
*
*/
@Export(name="privateEndpointConnections", refs={List.class,PrivateEndpointConnectionResponse.class}, tree="[0,1]")
private Output> privateEndpointConnections;
/**
* @return List of private endpoint connections.
*
*/
public Output> privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* Provisioning state of the Event Grid Domain Resource.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Provisioning state of the Event Grid Domain Resource.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* This determines if traffic is allowed over public network. By default it is enabled.
* You can further restrict to specific IPs by configuring <seealso cref="P:Microsoft.Azure.Events.ResourceProvider.Common.Contracts.DomainProperties.InboundIpRules" />
*
*/
@Export(name="publicNetworkAccess", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> publicNetworkAccess;
/**
* @return This determines if traffic is allowed over public network. By default it is enabled.
* You can further restrict to specific IPs by configuring <seealso cref="P:Microsoft.Azure.Events.ResourceProvider.Common.Contracts.DomainProperties.InboundIpRules" />
*
*/
public Output> publicNetworkAccess() {
return Codegen.optional(this.publicNetworkAccess);
}
/**
* The system metadata relating to the Event Grid Domain resource.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return The system metadata relating to the Event Grid Domain resource.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Tags of the resource.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Tags of the resource.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Type of the resource.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Type of the resource.
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Domain(java.lang.String name) {
this(name, DomainArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Domain(java.lang.String name, DomainArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Domain(java.lang.String name, DomainArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:eventgrid:Domain", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Domain(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:eventgrid:Domain", name, null, makeResourceOptions(options, id), false);
}
private static DomainArgs makeArgs(DomainArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? DomainArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:eventgrid/v20180915preview:Domain").build()),
Output.of(Alias.builder().type("azure-native:eventgrid/v20190201preview:Domain").build()),
Output.of(Alias.builder().type("azure-native:eventgrid/v20190601:Domain").build()),
Output.of(Alias.builder().type("azure-native:eventgrid/v20200101preview:Domain").build()),
Output.of(Alias.builder().type("azure-native:eventgrid/v20200401preview:Domain").build()),
Output.of(Alias.builder().type("azure-native:eventgrid/v20200601:Domain").build()),
Output.of(Alias.builder().type("azure-native:eventgrid/v20201015preview:Domain").build()),
Output.of(Alias.builder().type("azure-native:eventgrid/v20210601preview:Domain").build()),
Output.of(Alias.builder().type("azure-native:eventgrid/v20211015preview:Domain").build()),
Output.of(Alias.builder().type("azure-native:eventgrid/v20211201:Domain").build()),
Output.of(Alias.builder().type("azure-native:eventgrid/v20220615:Domain").build()),
Output.of(Alias.builder().type("azure-native:eventgrid/v20230601preview:Domain").build()),
Output.of(Alias.builder().type("azure-native:eventgrid/v20231215preview:Domain").build()),
Output.of(Alias.builder().type("azure-native:eventgrid/v20240601preview:Domain").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Domain get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Domain(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy