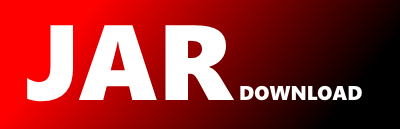
com.pulumi.azurenative.eventgrid.PartnerNamespaceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.eventgrid;
import com.pulumi.azurenative.eventgrid.enums.PartnerTopicRoutingMode;
import com.pulumi.azurenative.eventgrid.enums.PublicNetworkAccess;
import com.pulumi.azurenative.eventgrid.inputs.InboundIpRuleArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PartnerNamespaceArgs extends com.pulumi.resources.ResourceArgs {
public static final PartnerNamespaceArgs Empty = new PartnerNamespaceArgs();
/**
* This boolean is used to enable or disable local auth. Default value is false. When the property is set to true, only AAD token will be used to authenticate if user is allowed to publish to the partner namespace.
*
*/
@Import(name="disableLocalAuth")
private @Nullable Output disableLocalAuth;
/**
* @return This boolean is used to enable or disable local auth. Default value is false. When the property is set to true, only AAD token will be used to authenticate if user is allowed to publish to the partner namespace.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy