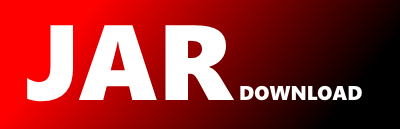
com.pulumi.azurenative.eventhub.ApplicationGroupArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.eventhub;
import com.pulumi.azurenative.eventhub.inputs.ThrottlingPolicyArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ApplicationGroupArgs extends com.pulumi.resources.ResourceArgs {
public static final ApplicationGroupArgs Empty = new ApplicationGroupArgs();
/**
* The Application Group name
*
*/
@Import(name="applicationGroupName")
private @Nullable Output applicationGroupName;
/**
* @return The Application Group name
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy