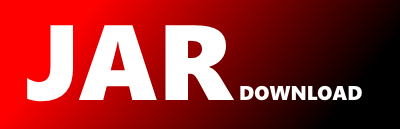
com.pulumi.azurenative.hdinsight.outputs.RoleResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.hdinsight.outputs;
import com.pulumi.azurenative.hdinsight.outputs.AutoscaleResponse;
import com.pulumi.azurenative.hdinsight.outputs.DataDisksGroupsResponse;
import com.pulumi.azurenative.hdinsight.outputs.HardwareProfileResponse;
import com.pulumi.azurenative.hdinsight.outputs.OsProfileResponse;
import com.pulumi.azurenative.hdinsight.outputs.ScriptActionResponse;
import com.pulumi.azurenative.hdinsight.outputs.VirtualNetworkProfileResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class RoleResponse {
/**
* @return The autoscale configurations.
*
*/
private @Nullable AutoscaleResponse autoscaleConfiguration;
/**
* @return The data disks groups for the role.
*
*/
private @Nullable List dataDisksGroups;
/**
* @return Indicates whether encrypt the data disks.
*
*/
private @Nullable Boolean encryptDataDisks;
/**
* @return The hardware profile.
*
*/
private @Nullable HardwareProfileResponse hardwareProfile;
/**
* @return The minimum instance count of the cluster.
*
*/
private @Nullable Integer minInstanceCount;
/**
* @return The name of the role.
*
*/
private @Nullable String name;
/**
* @return The operating system profile.
*
*/
private @Nullable OsProfileResponse osProfile;
/**
* @return The list of script actions on the role.
*
*/
private @Nullable List scriptActions;
/**
* @return The instance count of the cluster.
*
*/
private @Nullable Integer targetInstanceCount;
/**
* @return The name of the virtual machine group.
*
*/
private @Nullable String vMGroupName;
/**
* @return The virtual network profile.
*
*/
private @Nullable VirtualNetworkProfileResponse virtualNetworkProfile;
private RoleResponse() {}
/**
* @return The autoscale configurations.
*
*/
public Optional autoscaleConfiguration() {
return Optional.ofNullable(this.autoscaleConfiguration);
}
/**
* @return The data disks groups for the role.
*
*/
public List dataDisksGroups() {
return this.dataDisksGroups == null ? List.of() : this.dataDisksGroups;
}
/**
* @return Indicates whether encrypt the data disks.
*
*/
public Optional encryptDataDisks() {
return Optional.ofNullable(this.encryptDataDisks);
}
/**
* @return The hardware profile.
*
*/
public Optional hardwareProfile() {
return Optional.ofNullable(this.hardwareProfile);
}
/**
* @return The minimum instance count of the cluster.
*
*/
public Optional minInstanceCount() {
return Optional.ofNullable(this.minInstanceCount);
}
/**
* @return The name of the role.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return The operating system profile.
*
*/
public Optional osProfile() {
return Optional.ofNullable(this.osProfile);
}
/**
* @return The list of script actions on the role.
*
*/
public List scriptActions() {
return this.scriptActions == null ? List.of() : this.scriptActions;
}
/**
* @return The instance count of the cluster.
*
*/
public Optional targetInstanceCount() {
return Optional.ofNullable(this.targetInstanceCount);
}
/**
* @return The name of the virtual machine group.
*
*/
public Optional vMGroupName() {
return Optional.ofNullable(this.vMGroupName);
}
/**
* @return The virtual network profile.
*
*/
public Optional virtualNetworkProfile() {
return Optional.ofNullable(this.virtualNetworkProfile);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(RoleResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable AutoscaleResponse autoscaleConfiguration;
private @Nullable List dataDisksGroups;
private @Nullable Boolean encryptDataDisks;
private @Nullable HardwareProfileResponse hardwareProfile;
private @Nullable Integer minInstanceCount;
private @Nullable String name;
private @Nullable OsProfileResponse osProfile;
private @Nullable List scriptActions;
private @Nullable Integer targetInstanceCount;
private @Nullable String vMGroupName;
private @Nullable VirtualNetworkProfileResponse virtualNetworkProfile;
public Builder() {}
public Builder(RoleResponse defaults) {
Objects.requireNonNull(defaults);
this.autoscaleConfiguration = defaults.autoscaleConfiguration;
this.dataDisksGroups = defaults.dataDisksGroups;
this.encryptDataDisks = defaults.encryptDataDisks;
this.hardwareProfile = defaults.hardwareProfile;
this.minInstanceCount = defaults.minInstanceCount;
this.name = defaults.name;
this.osProfile = defaults.osProfile;
this.scriptActions = defaults.scriptActions;
this.targetInstanceCount = defaults.targetInstanceCount;
this.vMGroupName = defaults.vMGroupName;
this.virtualNetworkProfile = defaults.virtualNetworkProfile;
}
@CustomType.Setter
public Builder autoscaleConfiguration(@Nullable AutoscaleResponse autoscaleConfiguration) {
this.autoscaleConfiguration = autoscaleConfiguration;
return this;
}
@CustomType.Setter
public Builder dataDisksGroups(@Nullable List dataDisksGroups) {
this.dataDisksGroups = dataDisksGroups;
return this;
}
public Builder dataDisksGroups(DataDisksGroupsResponse... dataDisksGroups) {
return dataDisksGroups(List.of(dataDisksGroups));
}
@CustomType.Setter
public Builder encryptDataDisks(@Nullable Boolean encryptDataDisks) {
this.encryptDataDisks = encryptDataDisks;
return this;
}
@CustomType.Setter
public Builder hardwareProfile(@Nullable HardwareProfileResponse hardwareProfile) {
this.hardwareProfile = hardwareProfile;
return this;
}
@CustomType.Setter
public Builder minInstanceCount(@Nullable Integer minInstanceCount) {
this.minInstanceCount = minInstanceCount;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder osProfile(@Nullable OsProfileResponse osProfile) {
this.osProfile = osProfile;
return this;
}
@CustomType.Setter
public Builder scriptActions(@Nullable List scriptActions) {
this.scriptActions = scriptActions;
return this;
}
public Builder scriptActions(ScriptActionResponse... scriptActions) {
return scriptActions(List.of(scriptActions));
}
@CustomType.Setter
public Builder targetInstanceCount(@Nullable Integer targetInstanceCount) {
this.targetInstanceCount = targetInstanceCount;
return this;
}
@CustomType.Setter
public Builder vMGroupName(@Nullable String vMGroupName) {
this.vMGroupName = vMGroupName;
return this;
}
@CustomType.Setter
public Builder virtualNetworkProfile(@Nullable VirtualNetworkProfileResponse virtualNetworkProfile) {
this.virtualNetworkProfile = virtualNetworkProfile;
return this;
}
public RoleResponse build() {
final var _resultValue = new RoleResponse();
_resultValue.autoscaleConfiguration = autoscaleConfiguration;
_resultValue.dataDisksGroups = dataDisksGroups;
_resultValue.encryptDataDisks = encryptDataDisks;
_resultValue.hardwareProfile = hardwareProfile;
_resultValue.minInstanceCount = minInstanceCount;
_resultValue.name = name;
_resultValue.osProfile = osProfile;
_resultValue.scriptActions = scriptActions;
_resultValue.targetInstanceCount = targetInstanceCount;
_resultValue.vMGroupName = vMGroupName;
_resultValue.virtualNetworkProfile = virtualNetworkProfile;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy