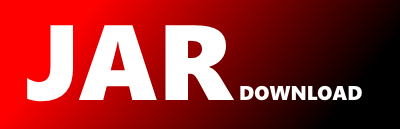
com.pulumi.azurenative.hybridcontainerservice.AgentPool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.hybridcontainerservice;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.hybridcontainerservice.AgentPoolArgs;
import com.pulumi.azurenative.hybridcontainerservice.outputs.AgentPoolProvisioningStatusResponseStatus;
import com.pulumi.azurenative.hybridcontainerservice.outputs.AgentPoolResponseExtendedLocation;
import com.pulumi.azurenative.hybridcontainerservice.outputs.CloudProviderProfileResponse;
import com.pulumi.azurenative.hybridcontainerservice.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The agentPool resource definition
* Azure REST API version: 2022-09-01-preview. Prior API version in Azure Native 1.x: 2022-05-01-preview.
*
* ## Example Usage
* ### PutAgentPool
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.hybridcontainerservice.AgentPool;
* import com.pulumi.azurenative.hybridcontainerservice.AgentPoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var agentPool = new AgentPool("agentPool", AgentPoolArgs.builder()
* .agentPoolName("test-hybridaksnodepool")
* .count(1)
* .location("westus")
* .osType("Linux")
* .resourceGroupName("test-arcappliance-resgrp")
* .resourceName("test-hybridakscluster")
* .vmSize("Standard_A4_v2")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:hybridcontainerservice:AgentPool test-hybridaksnodepool /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.HybridContainerService/provisionedClusters/{resourceName}/agentPools/{agentPoolName}
* ```
*
*/
@ResourceType(type="azure-native:hybridcontainerservice:AgentPool")
public class AgentPool extends com.pulumi.resources.CustomResource {
/**
* AvailabilityZones - The list of Availability zones to use for nodes. Datacenter racks modelled as zones
*
*/
@Export(name="availabilityZones", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> availabilityZones;
/**
* @return AvailabilityZones - The list of Availability zones to use for nodes. Datacenter racks modelled as zones
*
*/
public Output>> availabilityZones() {
return Codegen.optional(this.availabilityZones);
}
/**
* The underlying cloud infra provider properties.
*
*/
@Export(name="cloudProviderProfile", refs={CloudProviderProfileResponse.class}, tree="[0]")
private Output* @Nullable */ CloudProviderProfileResponse> cloudProviderProfile;
/**
* @return The underlying cloud infra provider properties.
*
*/
public Output> cloudProviderProfile() {
return Codegen.optional(this.cloudProviderProfile);
}
/**
* Count - Number of agents to host docker containers. Allowed values must be in the range of 1 to 100 (inclusive). The default value is 1.
*
*/
@Export(name="count", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> count;
/**
* @return Count - Number of agents to host docker containers. Allowed values must be in the range of 1 to 100 (inclusive). The default value is 1.
*
*/
public Output> count() {
return Codegen.optional(this.count);
}
@Export(name="extendedLocation", refs={AgentPoolResponseExtendedLocation.class}, tree="[0]")
private Output* @Nullable */ AgentPoolResponseExtendedLocation> extendedLocation;
public Output> extendedLocation() {
return Codegen.optional(this.extendedLocation);
}
/**
* The resource location
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> location;
/**
* @return The resource location
*
*/
public Output> location() {
return Codegen.optional(this.location);
}
/**
* The maximum number of nodes for auto-scaling
*
*/
@Export(name="maxCount", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> maxCount;
/**
* @return The maximum number of nodes for auto-scaling
*
*/
public Output> maxCount() {
return Codegen.optional(this.maxCount);
}
/**
* The maximum number of pods that can run on a node.
*
*/
@Export(name="maxPods", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> maxPods;
/**
* @return The maximum number of pods that can run on a node.
*
*/
public Output> maxPods() {
return Codegen.optional(this.maxPods);
}
/**
* The minimum number of nodes for auto-scaling
*
*/
@Export(name="minCount", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> minCount;
/**
* @return The minimum number of nodes for auto-scaling
*
*/
public Output> minCount() {
return Codegen.optional(this.minCount);
}
/**
* Mode - AgentPoolMode represents mode of an agent pool. Possible values include: 'System', 'LB', 'User'. Default is 'User'
*
*/
@Export(name="mode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> mode;
/**
* @return Mode - AgentPoolMode represents mode of an agent pool. Possible values include: 'System', 'LB', 'User'. Default is 'User'
*
*/
public Output> mode() {
return Codegen.optional(this.mode);
}
/**
* Resource Name
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource Name
*
*/
public Output name() {
return this.name;
}
/**
* The version of node image
*
*/
@Export(name="nodeImageVersion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> nodeImageVersion;
/**
* @return The version of node image
*
*/
public Output> nodeImageVersion() {
return Codegen.optional(this.nodeImageVersion);
}
/**
* NodeLabels - Agent pool node labels to be persisted across all nodes in agent pool.
*
*/
@Export(name="nodeLabels", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> nodeLabels;
/**
* @return NodeLabels - Agent pool node labels to be persisted across all nodes in agent pool.
*
*/
public Output>> nodeLabels() {
return Codegen.optional(this.nodeLabels);
}
/**
* NodeTaints - Taints added to new nodes during node pool create and scale. For example, key=value:NoSchedule.
*
*/
@Export(name="nodeTaints", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> nodeTaints;
/**
* @return NodeTaints - Taints added to new nodes during node pool create and scale. For example, key=value:NoSchedule.
*
*/
public Output>> nodeTaints() {
return Codegen.optional(this.nodeTaints);
}
/**
* OsType - OsType to be used to specify os type. Choose from Linux and Windows. Default to Linux. Possible values include: 'Linux', 'Windows'
*
*/
@Export(name="osType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> osType;
/**
* @return OsType - OsType to be used to specify os type. Choose from Linux and Windows. Default to Linux. Possible values include: 'Linux', 'Windows'
*
*/
public Output> osType() {
return Codegen.optional(this.osType);
}
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
public Output provisioningState() {
return this.provisioningState;
}
/**
* HybridAKSNodePoolStatus defines the observed state of HybridAKSNodePool
*
*/
@Export(name="status", refs={AgentPoolProvisioningStatusResponseStatus.class}, tree="[0]")
private Output* @Nullable */ AgentPoolProvisioningStatusResponseStatus> status;
/**
* @return HybridAKSNodePoolStatus defines the observed state of HybridAKSNodePool
*
*/
public Output> status() {
return Codegen.optional(this.status);
}
/**
* Metadata pertaining to creation and last modification of the resource.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Resource tags
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Resource Type
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource Type
*
*/
public Output type() {
return this.type;
}
/**
* VmSize - The size of the agent pool VMs.
*
*/
@Export(name="vmSize", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> vmSize;
/**
* @return VmSize - The size of the agent pool VMs.
*
*/
public Output> vmSize() {
return Codegen.optional(this.vmSize);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public AgentPool(java.lang.String name) {
this(name, AgentPoolArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public AgentPool(java.lang.String name, AgentPoolArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public AgentPool(java.lang.String name, AgentPoolArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:hybridcontainerservice:AgentPool", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private AgentPool(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:hybridcontainerservice:AgentPool", name, null, makeResourceOptions(options, id), false);
}
private static AgentPoolArgs makeArgs(AgentPoolArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? AgentPoolArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:hybridcontainerservice:agentPool").build()),
Output.of(Alias.builder().type("azure-native:hybridcontainerservice/v20220501preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:hybridcontainerservice/v20220501preview:agentPool").build()),
Output.of(Alias.builder().type("azure-native:hybridcontainerservice/v20220901preview:AgentPool").build()),
Output.of(Alias.builder().type("azure-native:hybridcontainerservice/v20220901preview:agentPool").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static AgentPool get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new AgentPool(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy