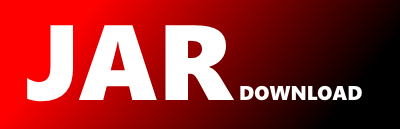
com.pulumi.azurenative.importexport.inputs.JobDetailsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.importexport.inputs;
import com.pulumi.azurenative.importexport.inputs.DeliveryPackageInformationArgs;
import com.pulumi.azurenative.importexport.inputs.DriveStatusArgs;
import com.pulumi.azurenative.importexport.inputs.EncryptionKeyDetailsArgs;
import com.pulumi.azurenative.importexport.inputs.ExportArgs;
import com.pulumi.azurenative.importexport.inputs.PackageInformationArgs;
import com.pulumi.azurenative.importexport.inputs.ReturnAddressArgs;
import com.pulumi.azurenative.importexport.inputs.ReturnShippingArgs;
import com.pulumi.azurenative.importexport.inputs.ShippingInformationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Specifies the job properties
*
*/
public final class JobDetailsArgs extends com.pulumi.resources.ResourceArgs {
public static final JobDetailsArgs Empty = new JobDetailsArgs();
/**
* Default value is false. Indicates whether the manifest files on the drives should be copied to block blobs.
*
*/
@Import(name="backupDriveManifest")
private @Nullable Output backupDriveManifest;
/**
* @return Default value is false. Indicates whether the manifest files on the drives should be copied to block blobs.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy