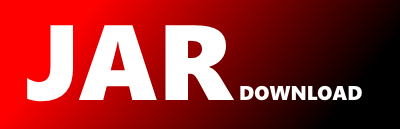
com.pulumi.azurenative.insights.WebTestArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.insights;
import com.pulumi.azurenative.insights.enums.WebTestKind;
import com.pulumi.azurenative.insights.inputs.WebTestGeolocationArgs;
import com.pulumi.azurenative.insights.inputs.WebTestPropertiesConfigurationArgs;
import com.pulumi.azurenative.insights.inputs.WebTestPropertiesRequestArgs;
import com.pulumi.azurenative.insights.inputs.WebTestPropertiesValidationRulesArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class WebTestArgs extends com.pulumi.resources.ResourceArgs {
public static final WebTestArgs Empty = new WebTestArgs();
/**
* An XML configuration specification for a WebTest.
*
*/
@Import(name="configuration")
private @Nullable Output configuration;
/**
* @return An XML configuration specification for a WebTest.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy