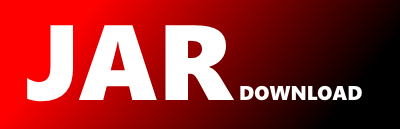
com.pulumi.azurenative.insights.outputs.GetLogProfileResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.insights.outputs;
import com.pulumi.azurenative.insights.outputs.RetentionPolicyResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetLogProfileResult {
/**
* @return the categories of the logs. These categories are created as is convenient to the user. Some values are: 'Write', 'Delete', and/or 'Action.'
*
*/
private List categories;
/**
* @return Azure resource Id
*
*/
private String id;
/**
* @return Resource location
*
*/
private String location;
/**
* @return List of regions for which Activity Log events should be stored or streamed. It is a comma separated list of valid ARM locations including the 'global' location.
*
*/
private List locations;
/**
* @return Azure resource name
*
*/
private String name;
/**
* @return the retention policy for the events in the log.
*
*/
private RetentionPolicyResponse retentionPolicy;
/**
* @return The service bus rule ID of the service bus namespace in which you would like to have Event Hubs created for streaming the Activity Log. The rule ID is of the format: '{service bus resource ID}/authorizationrules/{key name}'.
*
*/
private @Nullable String serviceBusRuleId;
/**
* @return the resource id of the storage account to which you would like to send the Activity Log.
*
*/
private @Nullable String storageAccountId;
/**
* @return Resource tags
*
*/
private @Nullable Map tags;
/**
* @return Azure resource type
*
*/
private String type;
private GetLogProfileResult() {}
/**
* @return the categories of the logs. These categories are created as is convenient to the user. Some values are: 'Write', 'Delete', and/or 'Action.'
*
*/
public List categories() {
return this.categories;
}
/**
* @return Azure resource Id
*
*/
public String id() {
return this.id;
}
/**
* @return Resource location
*
*/
public String location() {
return this.location;
}
/**
* @return List of regions for which Activity Log events should be stored or streamed. It is a comma separated list of valid ARM locations including the 'global' location.
*
*/
public List locations() {
return this.locations;
}
/**
* @return Azure resource name
*
*/
public String name() {
return this.name;
}
/**
* @return the retention policy for the events in the log.
*
*/
public RetentionPolicyResponse retentionPolicy() {
return this.retentionPolicy;
}
/**
* @return The service bus rule ID of the service bus namespace in which you would like to have Event Hubs created for streaming the Activity Log. The rule ID is of the format: '{service bus resource ID}/authorizationrules/{key name}'.
*
*/
public Optional serviceBusRuleId() {
return Optional.ofNullable(this.serviceBusRuleId);
}
/**
* @return the resource id of the storage account to which you would like to send the Activity Log.
*
*/
public Optional storageAccountId() {
return Optional.ofNullable(this.storageAccountId);
}
/**
* @return Resource tags
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Azure resource type
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetLogProfileResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List categories;
private String id;
private String location;
private List locations;
private String name;
private RetentionPolicyResponse retentionPolicy;
private @Nullable String serviceBusRuleId;
private @Nullable String storageAccountId;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetLogProfileResult defaults) {
Objects.requireNonNull(defaults);
this.categories = defaults.categories;
this.id = defaults.id;
this.location = defaults.location;
this.locations = defaults.locations;
this.name = defaults.name;
this.retentionPolicy = defaults.retentionPolicy;
this.serviceBusRuleId = defaults.serviceBusRuleId;
this.storageAccountId = defaults.storageAccountId;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder categories(List categories) {
if (categories == null) {
throw new MissingRequiredPropertyException("GetLogProfileResult", "categories");
}
this.categories = categories;
return this;
}
public Builder categories(String... categories) {
return categories(List.of(categories));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetLogProfileResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetLogProfileResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder locations(List locations) {
if (locations == null) {
throw new MissingRequiredPropertyException("GetLogProfileResult", "locations");
}
this.locations = locations;
return this;
}
public Builder locations(String... locations) {
return locations(List.of(locations));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetLogProfileResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder retentionPolicy(RetentionPolicyResponse retentionPolicy) {
if (retentionPolicy == null) {
throw new MissingRequiredPropertyException("GetLogProfileResult", "retentionPolicy");
}
this.retentionPolicy = retentionPolicy;
return this;
}
@CustomType.Setter
public Builder serviceBusRuleId(@Nullable String serviceBusRuleId) {
this.serviceBusRuleId = serviceBusRuleId;
return this;
}
@CustomType.Setter
public Builder storageAccountId(@Nullable String storageAccountId) {
this.storageAccountId = storageAccountId;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetLogProfileResult", "type");
}
this.type = type;
return this;
}
public GetLogProfileResult build() {
final var _resultValue = new GetLogProfileResult();
_resultValue.categories = categories;
_resultValue.id = id;
_resultValue.location = location;
_resultValue.locations = locations;
_resultValue.name = name;
_resultValue.retentionPolicy = retentionPolicy;
_resultValue.serviceBusRuleId = serviceBusRuleId;
_resultValue.storageAccountId = storageAccountId;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy