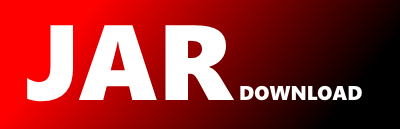
com.pulumi.azurenative.iotoperations.inputs.DataFlowEndpointKafkaArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.iotoperations.inputs;
import com.pulumi.azurenative.iotoperations.enums.DataFlowEndpointKafkaAcks;
import com.pulumi.azurenative.iotoperations.enums.DataFlowEndpointKafkaCompression;
import com.pulumi.azurenative.iotoperations.enums.DataFlowEndpointKafkaPartitionStrategy;
import com.pulumi.azurenative.iotoperations.enums.OperationalMode;
import com.pulumi.azurenative.iotoperations.inputs.DataFlowEndpointKafkaBatchingArgs;
import com.pulumi.azurenative.iotoperations.inputs.TlsPropertiesArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Kafka endpoint properties
*
*/
public final class DataFlowEndpointKafkaArgs extends com.pulumi.resources.ResourceArgs {
public static final DataFlowEndpointKafkaArgs Empty = new DataFlowEndpointKafkaArgs();
/**
* Batching configuration.
*
*/
@Import(name="batching")
private @Nullable Output batching;
/**
* @return Batching configuration.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy