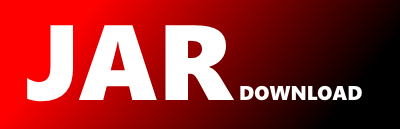
com.pulumi.azurenative.iotoperationsmq.BrokerArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.iotoperationsmq;
import com.pulumi.azurenative.iotoperationsmq.enums.BrokerMemoryProfile;
import com.pulumi.azurenative.iotoperationsmq.enums.RunMode;
import com.pulumi.azurenative.iotoperationsmq.inputs.BrokerDiagnosticsArgs;
import com.pulumi.azurenative.iotoperationsmq.inputs.CardinalityArgs;
import com.pulumi.azurenative.iotoperationsmq.inputs.CertManagerCertOptionsArgs;
import com.pulumi.azurenative.iotoperationsmq.inputs.ContainerImageArgs;
import com.pulumi.azurenative.iotoperationsmq.inputs.DiskBackedMessageBufferSettingsArgs;
import com.pulumi.azurenative.iotoperationsmq.inputs.ExtendedLocationPropertyArgs;
import com.pulumi.azurenative.iotoperationsmq.inputs.NodeTolerationsArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class BrokerArgs extends com.pulumi.resources.ResourceArgs {
public static final BrokerArgs Empty = new BrokerArgs();
/**
* The details of Authentication Docker Image.
*
*/
@Import(name="authImage", required=true)
private Output authImage;
/**
* @return The details of Authentication Docker Image.
*
*/
public Output authImage() {
return this.authImage;
}
/**
* The details of Broker Docker Image.
*
*/
@Import(name="brokerImage", required=true)
private Output brokerImage;
/**
* @return The details of Broker Docker Image.
*
*/
public Output brokerImage() {
return this.brokerImage;
}
/**
* Name of MQ broker resource
*
*/
@Import(name="brokerName")
private @Nullable Output brokerName;
/**
* @return Name of MQ broker resource
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy