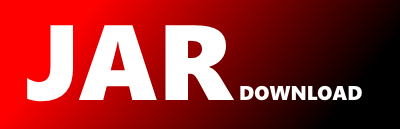
com.pulumi.azurenative.iotoperationsmq.outputs.DataLakeConnectorMapResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.iotoperationsmq.outputs;
import com.pulumi.azurenative.iotoperationsmq.outputs.DeltaTableResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DataLakeConnectorMapResponse {
/**
* @return Allowed latency for transferring data.
*
*/
private Integer allowedLatencySecs;
/**
* @return Client Id to use.
*
*/
private String clientId;
/**
* @return Maximum messages to send per Batch.
*
*/
private Double maxMessagesPerBatch;
/**
* @return Message payload type.
*
*/
private String messagePayloadType;
/**
* @return Mqtt source topic.
*
*/
private String mqttSourceTopic;
/**
* @return Quality of Service.
*
*/
private @Nullable Integer qos;
/**
* @return Delta table properties to use.
*
*/
private DeltaTableResponse table;
private DataLakeConnectorMapResponse() {}
/**
* @return Allowed latency for transferring data.
*
*/
public Integer allowedLatencySecs() {
return this.allowedLatencySecs;
}
/**
* @return Client Id to use.
*
*/
public String clientId() {
return this.clientId;
}
/**
* @return Maximum messages to send per Batch.
*
*/
public Double maxMessagesPerBatch() {
return this.maxMessagesPerBatch;
}
/**
* @return Message payload type.
*
*/
public String messagePayloadType() {
return this.messagePayloadType;
}
/**
* @return Mqtt source topic.
*
*/
public String mqttSourceTopic() {
return this.mqttSourceTopic;
}
/**
* @return Quality of Service.
*
*/
public Optional qos() {
return Optional.ofNullable(this.qos);
}
/**
* @return Delta table properties to use.
*
*/
public DeltaTableResponse table() {
return this.table;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DataLakeConnectorMapResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Integer allowedLatencySecs;
private String clientId;
private Double maxMessagesPerBatch;
private String messagePayloadType;
private String mqttSourceTopic;
private @Nullable Integer qos;
private DeltaTableResponse table;
public Builder() {}
public Builder(DataLakeConnectorMapResponse defaults) {
Objects.requireNonNull(defaults);
this.allowedLatencySecs = defaults.allowedLatencySecs;
this.clientId = defaults.clientId;
this.maxMessagesPerBatch = defaults.maxMessagesPerBatch;
this.messagePayloadType = defaults.messagePayloadType;
this.mqttSourceTopic = defaults.mqttSourceTopic;
this.qos = defaults.qos;
this.table = defaults.table;
}
@CustomType.Setter
public Builder allowedLatencySecs(Integer allowedLatencySecs) {
if (allowedLatencySecs == null) {
throw new MissingRequiredPropertyException("DataLakeConnectorMapResponse", "allowedLatencySecs");
}
this.allowedLatencySecs = allowedLatencySecs;
return this;
}
@CustomType.Setter
public Builder clientId(String clientId) {
if (clientId == null) {
throw new MissingRequiredPropertyException("DataLakeConnectorMapResponse", "clientId");
}
this.clientId = clientId;
return this;
}
@CustomType.Setter
public Builder maxMessagesPerBatch(Double maxMessagesPerBatch) {
if (maxMessagesPerBatch == null) {
throw new MissingRequiredPropertyException("DataLakeConnectorMapResponse", "maxMessagesPerBatch");
}
this.maxMessagesPerBatch = maxMessagesPerBatch;
return this;
}
@CustomType.Setter
public Builder messagePayloadType(String messagePayloadType) {
if (messagePayloadType == null) {
throw new MissingRequiredPropertyException("DataLakeConnectorMapResponse", "messagePayloadType");
}
this.messagePayloadType = messagePayloadType;
return this;
}
@CustomType.Setter
public Builder mqttSourceTopic(String mqttSourceTopic) {
if (mqttSourceTopic == null) {
throw new MissingRequiredPropertyException("DataLakeConnectorMapResponse", "mqttSourceTopic");
}
this.mqttSourceTopic = mqttSourceTopic;
return this;
}
@CustomType.Setter
public Builder qos(@Nullable Integer qos) {
this.qos = qos;
return this;
}
@CustomType.Setter
public Builder table(DeltaTableResponse table) {
if (table == null) {
throw new MissingRequiredPropertyException("DataLakeConnectorMapResponse", "table");
}
this.table = table;
return this;
}
public DataLakeConnectorMapResponse build() {
final var _resultValue = new DataLakeConnectorMapResponse();
_resultValue.allowedLatencySecs = allowedLatencySecs;
_resultValue.clientId = clientId;
_resultValue.maxMessagesPerBatch = maxMessagesPerBatch;
_resultValue.messagePayloadType = messagePayloadType;
_resultValue.mqttSourceTopic = mqttSourceTopic;
_resultValue.qos = qos;
_resultValue.table = table;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy